Mastering Python Web Scraping: A Step-by-Step Guide
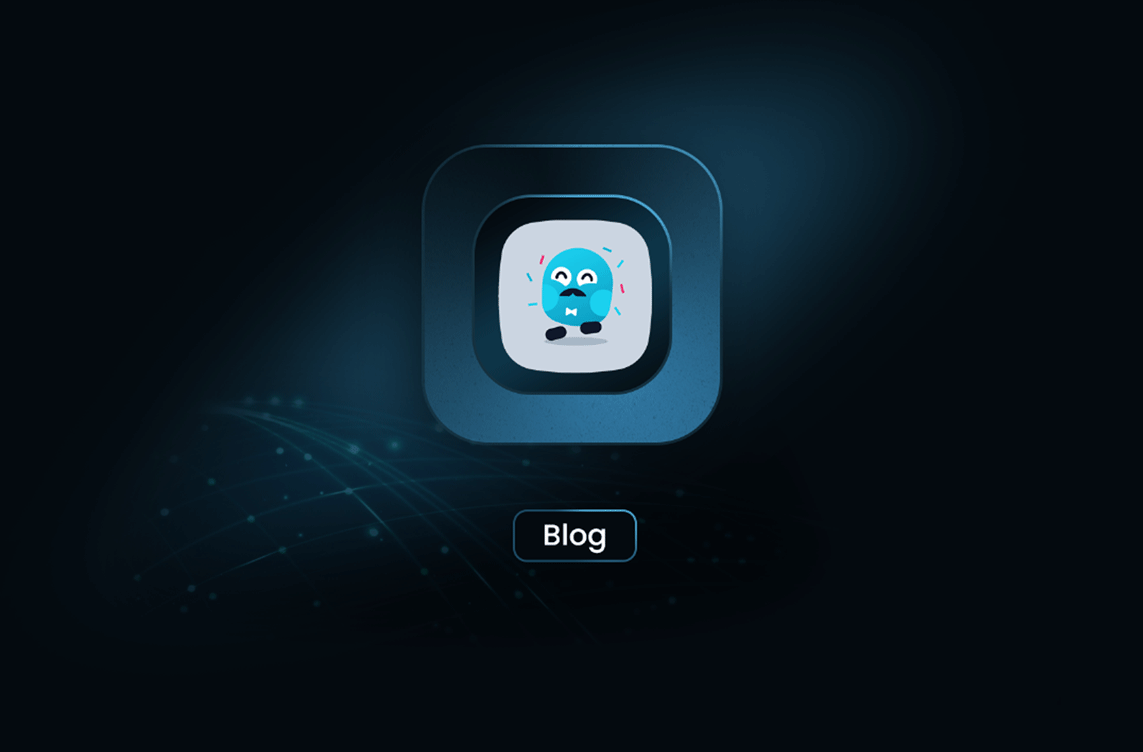
Web scraping is a powerful technique for extracting data from websites, and Python offers excellent libraries to help you do this efficiently. Whether you're looking to gather product information, social media data, or research content, Python’s simplicity and the robust ecosystem of libraries like BeautifulSoup, Scrapy, and Selenium make it the go-to language for web scraping.
1. What is Web Scraping?
Web scraping is the process of extracting information from websites. Some common use cases include:
- Gathering data from e-commerce websites for price monitoring.
- Extracting data from job postings.
- Scraping news websites for headlines or articles.
It’s important to follow ethical guidelines while scraping, such as checking the robots.txt
file of websites and ensuring you are not violating terms of service.
2. Setting Up Your Environment
To get started with Python web scraping, you need the following libraries:
Required Tools:
- Python (Make sure Python is installed)
- BeautifulSoup (for parsing HTML)
- Requests (for making HTTP requests)
- Selenium (optional, for websites requiring JavaScript interaction)
Install Required Libraries:
pip install beautifulsoup4 requests
(Optional, for Selenium)
pip install selenium
3. Making HTTP Requests with Python
Use the Requests library to fetch the HTML content of a webpage. Here’s a basic example:
import requests
url = 'https://example.com'
response = requests.get(url)
html_content = response.text
print(html_content)
Make sure to handle errors and timeouts properly in case the website is down or slow.
4. Parsing HTML with BeautifulSoup
Once you've fetched the HTML, use BeautifulSoup to extract specific elements from the page.
from bs4 import BeautifulSoup
soup = BeautifulSoup(html_content, 'html.parser')
headline = soup.find('h1').text # Find the first headline
print(headline)
Example: Extracting a list of items (e.g., product listings):
products = soup.find_all('div', class_='product-item')
for product in products:
title = product.find('h2').text
price = product.find('span', class_='price').text
print(f"Product: {title}, Price: {price}")
5. Web Scraping with Selenium (Optional)
For websites that require interaction or load content dynamically with JavaScript, use Selenium.
from selenium import webdriver
driver = webdriver.Chrome() # Make sure to install the Chrome WebDriver
driver.get('https://example.com')
html = driver.page_source
soup = BeautifulSoup(html, 'html.parser')
With Selenium, you can also interact with web elements like clicking buttons or filling forms.
6. Storing Scraped Data
After extracting data, you can store it in a CSV or JSON format.
Storing data in CSV:
import csv
data = [['Title', 'Price'], ['Item 1', '$20'], ['Item 2', '$30']]
with open('output.csv', mode='w', newline='') as file:
writer = csv.writer(file)
writer.writerows(data)
Storing data in JSON:
import json
data = [
{"title": "Item 1", "price": "$20"},
{"title": "Item 2", "price": "$30"}
]
with open('output.json', 'w') as f:
json.dump(data, f, indent=4)
7. Handling Common Issues
- Avoiding blocks: Use rotating proxies, adjust headers, and implement user-agent rotation.
- Rate-limiting: Add delays between requests to avoid overwhelming the target server.
- CAPTCHA handling: Some websites use CAPTCHAs to block bots. Consider using services to bypass them, or use manual interventions.
- Error handling: Implement try-except blocks to catch errors when requests fail.
8. Ethics and Legalities of Web Scraping
Web scraping can sometimes raise legal and ethical concerns. Always ensure:
- You adhere to the
robots.txt
rules for websites. - You comply with the website’s terms of service.
- Avoid overloading the website with too many requests in a short period.
Conclusion
Web scraping is a valuable skill that can unlock vast amounts of data from the web. With Python and the right libraries, you can easily extract, manipulate, and store this data. Always be mindful of the ethical guidelines, and explore different websites to practice and refine your skills.
That being said, while Python web scraping offers flexibility and control, it can also be time-consuming and complex for beginners. Managing headers, proxies, CAPTCHAs, and dynamically loaded content requires both technical knowledge and maintenance.
Is there any faster way to do web-scraping stuff?
If you're looking for a faster, simpler, and more user-friendly solution, mrscraper.com can be a great alternative:
- No Coding Required: Unlike Python web scraping, mrscraper is designed for users with no coding experience. With just a few clicks, you can set up and run your scraping tasks.
- AI-Powered Scraping: Mrscraper uses AI to intelligently extract data based on your prompts, saving you from manually writing scraping scripts.
- Built-in Pagination: For tasks that require scraping multiple pages, Mrscraper’s interface makes it easy to automate pagination without complex coding logic.
- Quick Results: Get the data you need without worrying about the technical hurdles of maintaining your own scripts and scraping infrastructure.
For those who need maximum flexibility and have experience with Python, writing your own scripts is still an excellent choice. But if you want to save time and get reliable results effortlessly, give mrscraper.coma try!
Table of Contents
Take a Taste of Easy Scraping!
Get started now!
Step up your web scraping
Find more insights here
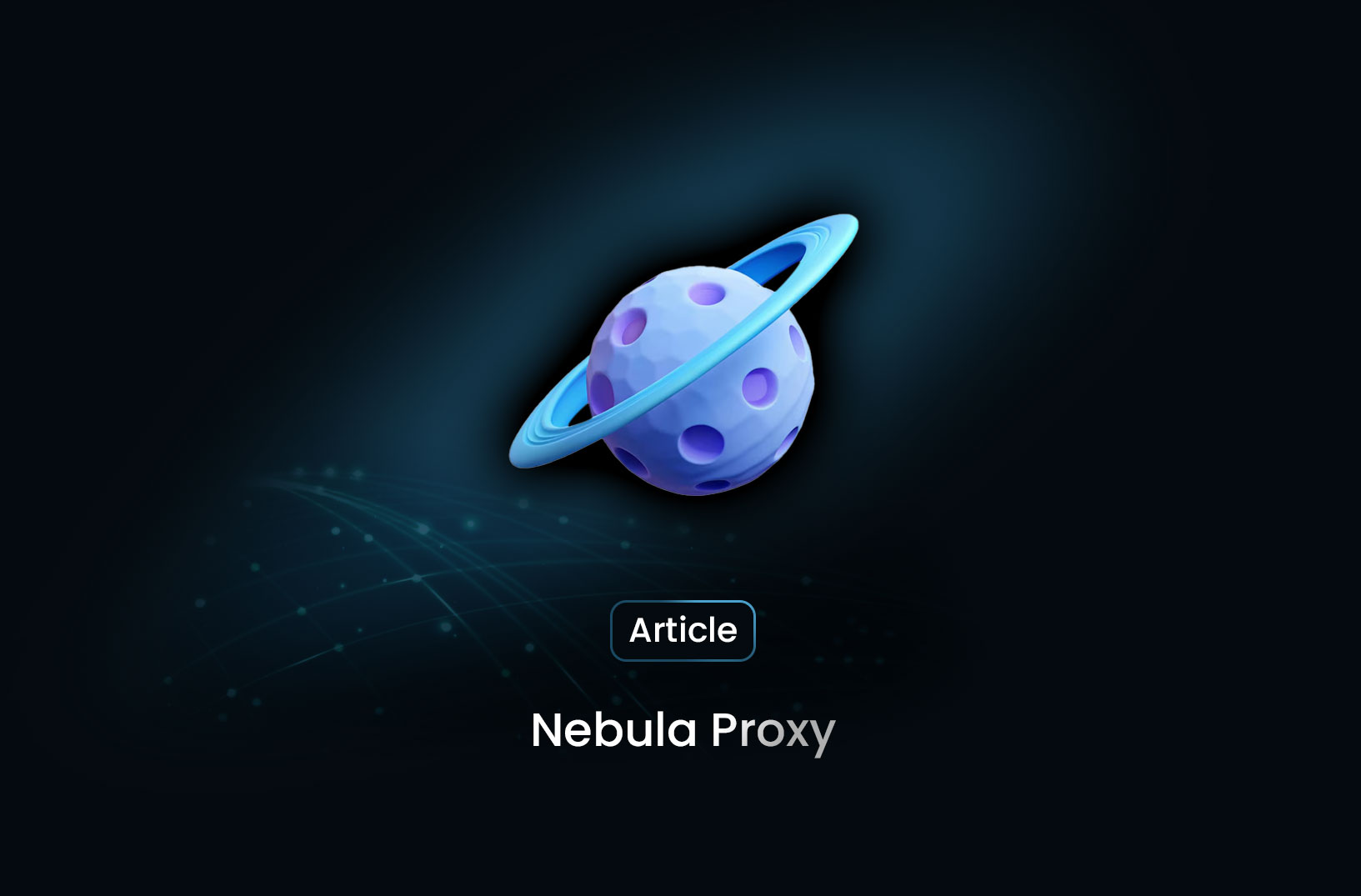
What is Nebula Proxy? A Powerful Tool for Web Scraping, SEO, and Online Privacy
Discover how Nebula Proxy enhances web scraping, SEO monitoring, and online privacy. Learn about its features, use cases, and setup, plus top alternatives for seamless data extraction and secure browsing.
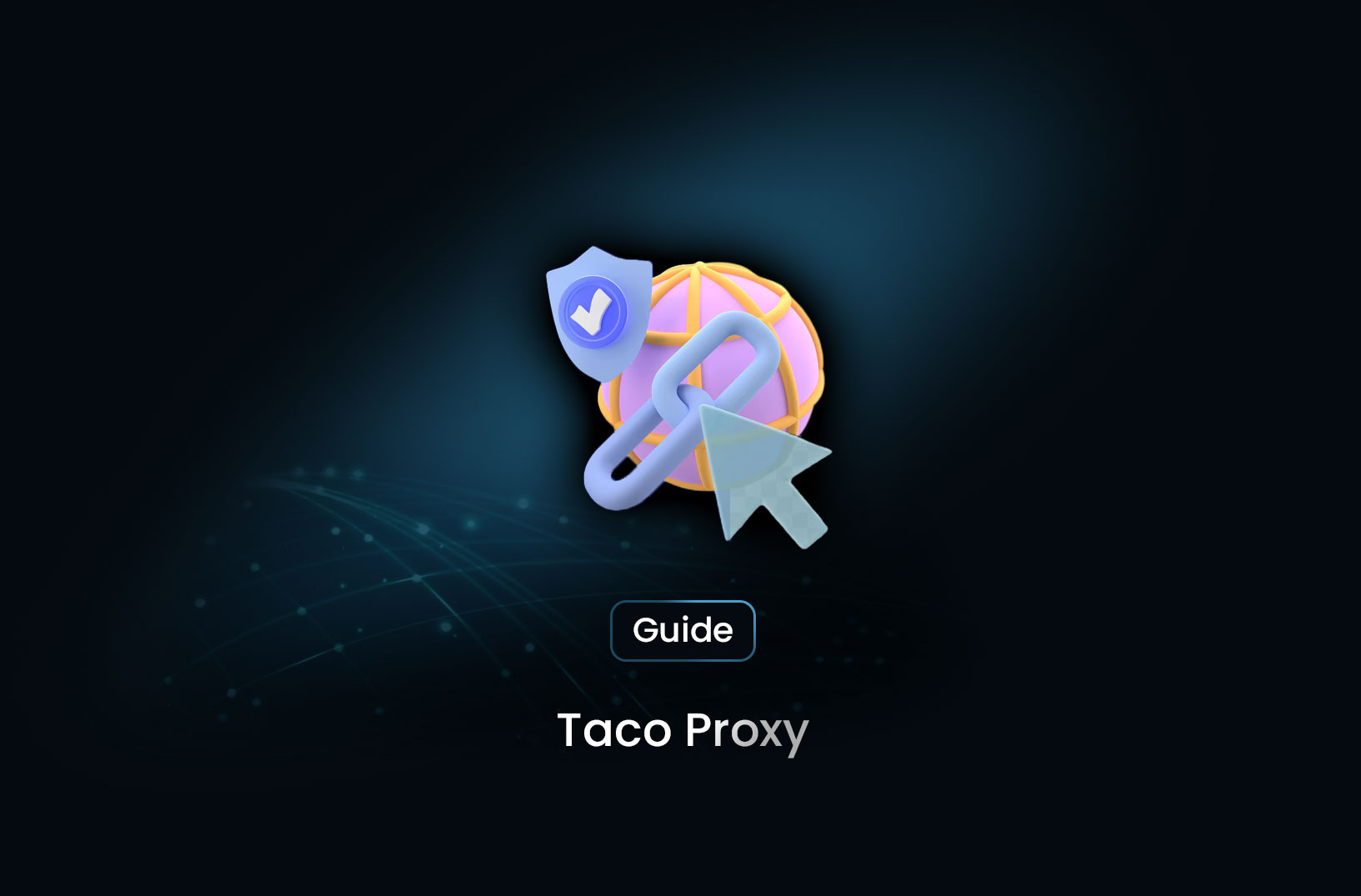
Taco Proxy: Understanding Its Role and Use Cases
Learn what Taco Proxy is, how it works, and its key use cases for web scraping, SEO monitoring, cybersecurity, and bypassing geo-restrictions. Get step-by-step proxy configuration guides for Python, Scrapy, and cURL.
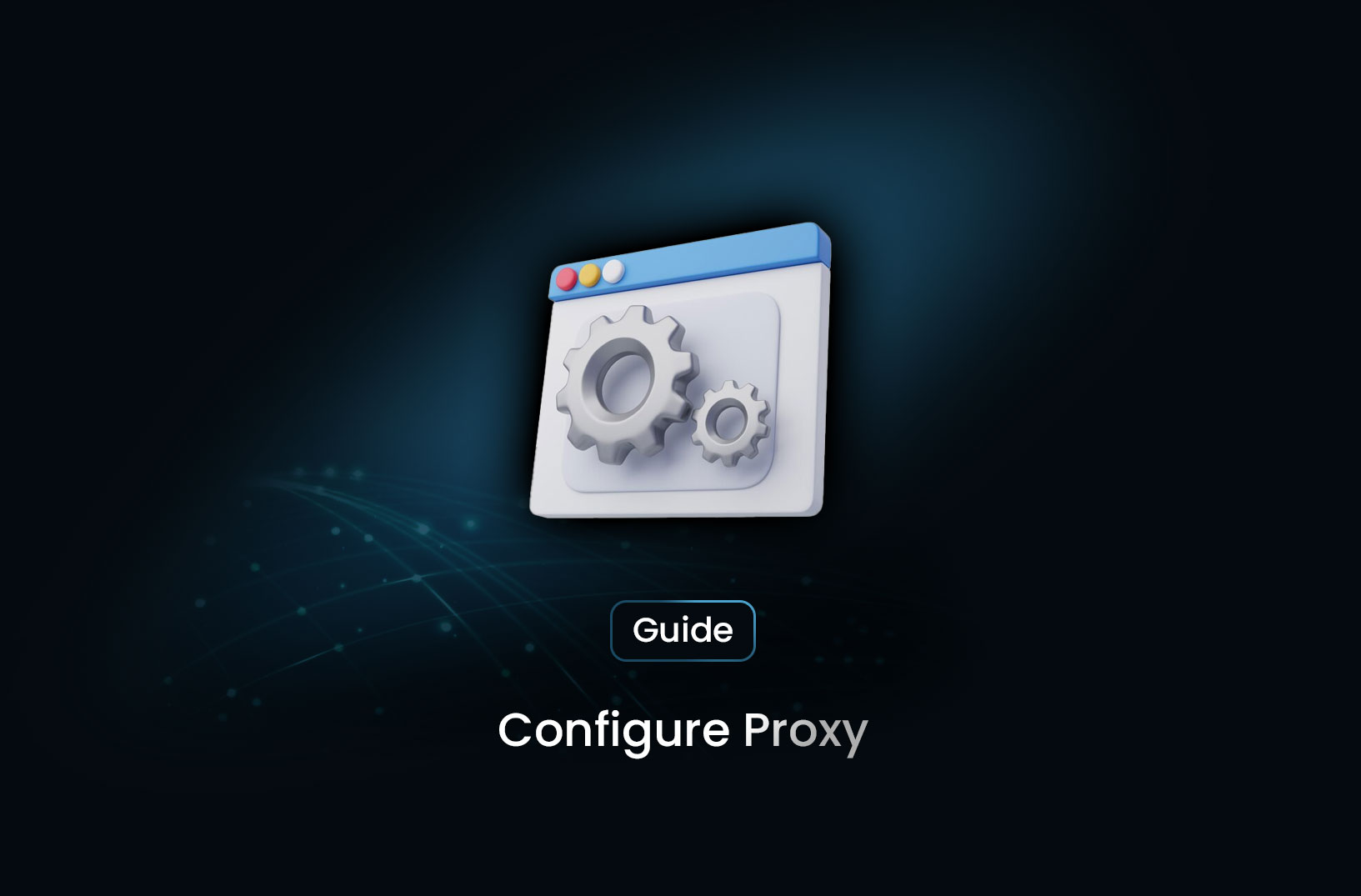
How to Configure Proxy
Learn how to set up a proxy on Windows, macOS, Linux, browsers, and command-line tools like cURL and Python.
@MrScraper_
@MrScraper