Using Proxy Chains to Increase Scraping Anonymity
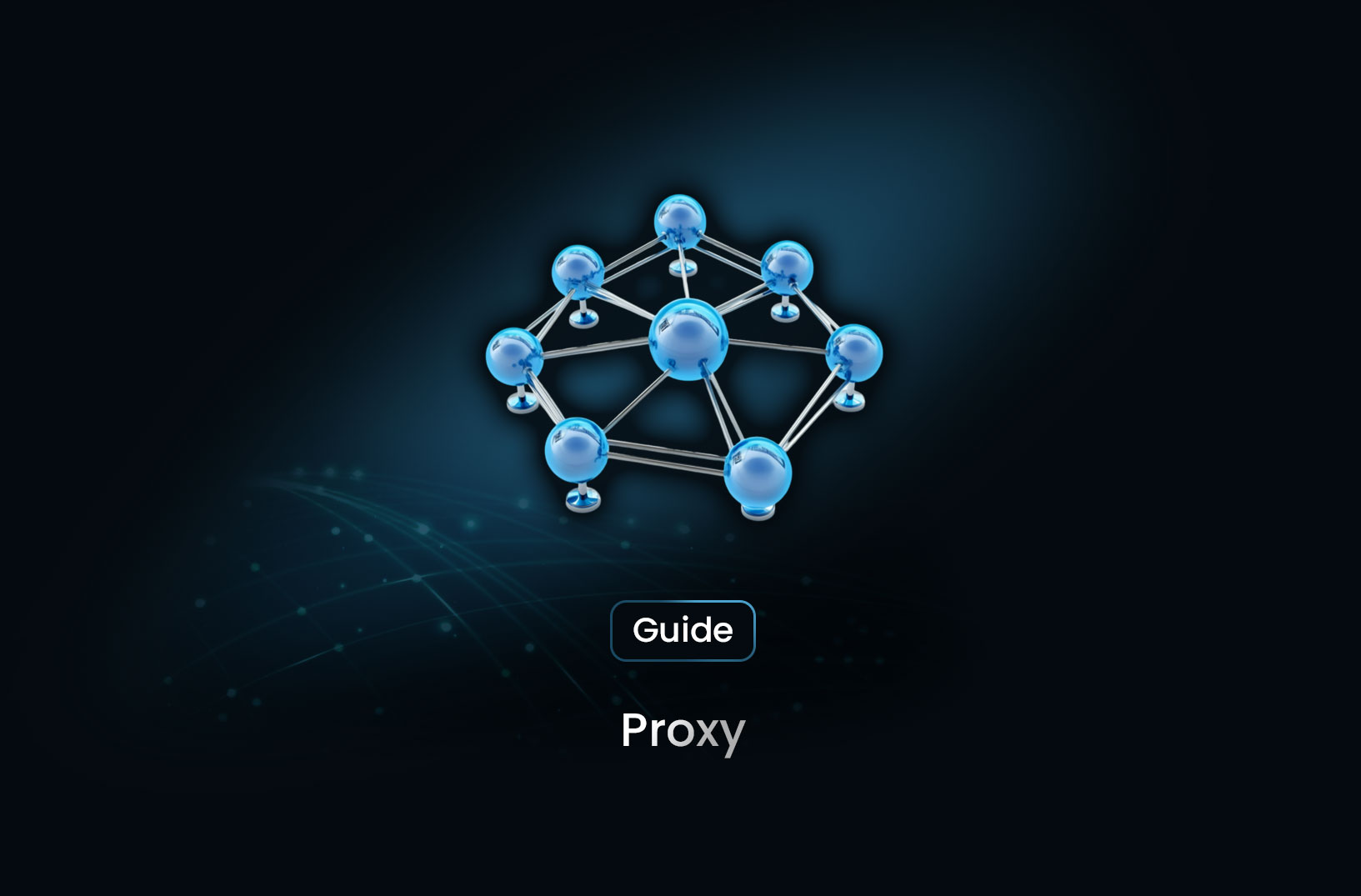
When web scraping, using a single proxy may not always be enough to maintain anonymity. Proxy chains, which route traffic through multiple proxies, add extra layers of obfuscation and reduce the chances of detection and blocking. This technique is especially useful when scraping sensitive data or bypassing aggressive anti-bot mechanisms.
Use Case: Scraping Without Detection for Market Research
A market research company needs to scrape competitor data without revealing its real IP. Using proxy chains, it routes requests through multiple proxies to ensure maximum anonymity and avoid detection.
How Proxy Chains Work
Proxy chaining involves passing a request through multiple proxies before reaching the target website. Each proxy in the chain masks the previous proxy’s IP, making it harder for websites to track and block the original source.
Example Flow of a Proxy Chain
Your Scraper → Proxy 1 (US IP) → Proxy 2 (UK IP) → Proxy 3 (Germany IP) → Target Website
Setting Up a Proxy Chain
1. Selecting Proxies for Chaining
Use a mix of:
- Residential proxies for legitimacy
- Datacenter proxies for speed
- SOCKS5 proxies for extra security
2. Configuring a Proxy Chain with cURL
Use cURL
to route traffic through multiple proxies:
curl -x socks5://proxy1:port -x socks5://proxy2:port -x socks5://proxy3:port https://example.com
This routes traffic through three proxies before reaching the target site.
3. Using Proxy Chains in Python
With the PySocks
library, you can create a SOCKS5 proxy chain:
import socks
import socket
import requests
# Set up SOCKS5 proxy
socks.set_default_proxy(socks.SOCKS5, "proxy1.com", 1080)
socket.socket = socks.socksocket
# First proxy request
response = requests.get("http://proxy2.com:port")
# Use the second proxy to forward the request
socks.set_default_proxy(socks.SOCKS5, "proxy2.com", 1080)
socket.socket = socks.socksocket
# Final request to target website
response = requests.get("https://example.com")
print(response.text)
4. Rotating Proxies for Additional Anonymity
To make proxy chains even more effective, rotate proxies at intervals to prevent detection.
import random
proxy_list = ["proxy1:port", "proxy2:port", "proxy3:port"]
selected_proxies = random.sample(proxy_list, 2)
socks.set_default_proxy(socks.SOCKS5, selected_proxies[0].split(":")[0], int(selected_proxies[0].split(":")[1]))
socket.socket = socks.socksocket
5. Using Tor as a Proxy Chain
Tor automatically routes traffic through multiple relays, creating a proxy chain.
Start Tor and configure it in Python:
proxy = {"http": "socks5h://127.0.0.1:9050", "https": "socks5h://127.0.0.1:9050"}
response = requests.get("https://check.torproject.org", proxies=proxy)
print(response.text)
Conclusion
Using proxy chains significantly increases anonymity when scraping by routing requests through multiple IPs. This method is useful for bypassing strict anti-bot mechanisms and protecting identity.
For a hassle-free scraping experience with built-in proxy rotation and anonymity, consider using Mrscraper.com to manage proxy chaining automatically.
Table of Contents
Take a Taste of Easy Scraping!
Get started now!
Step up your web scraping
Find more insights here
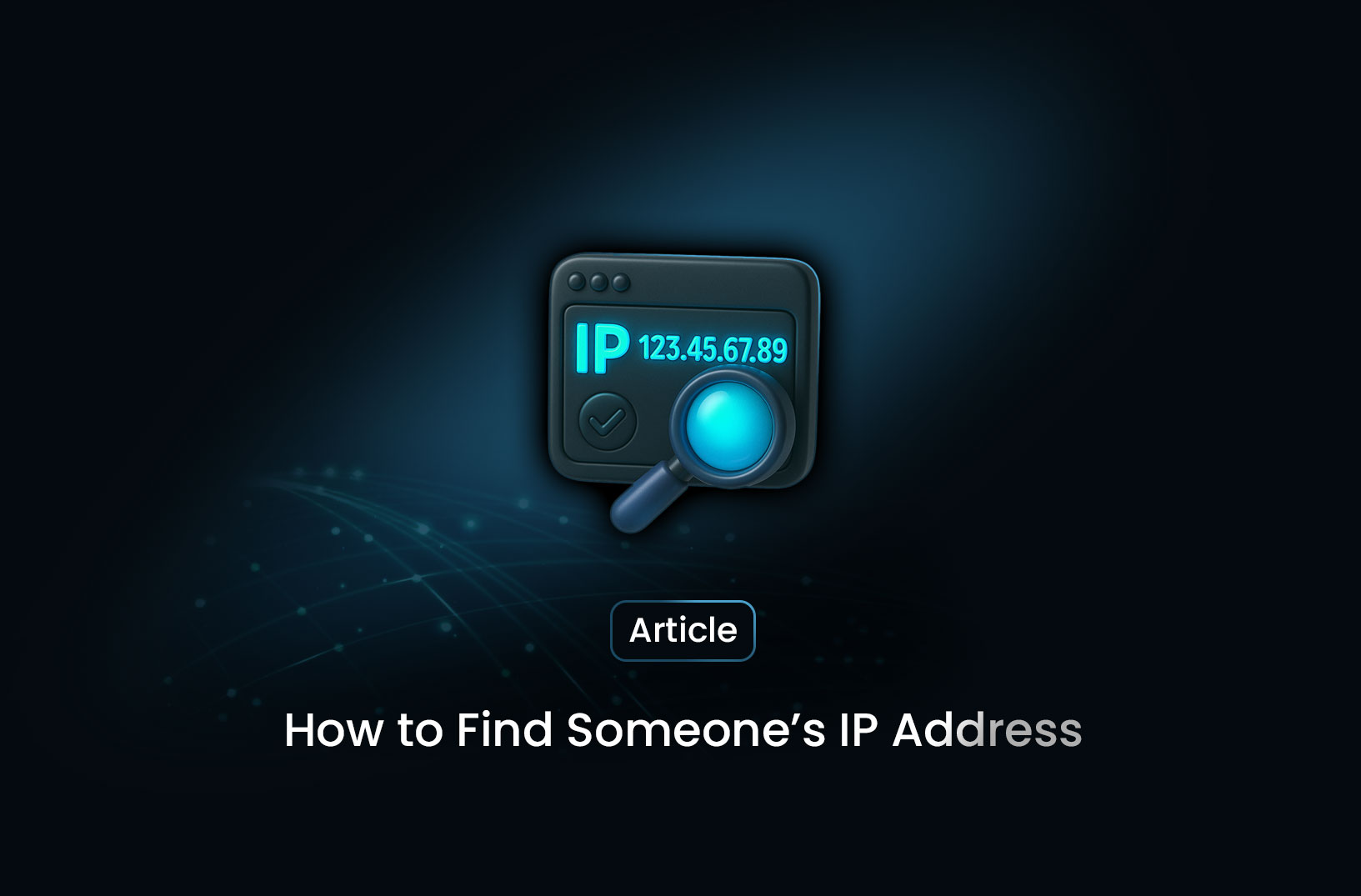
How to Find Someone’s IP Address (Legally and Safely)
Curious about how to find someone's IP address? Learn the legal, safe, and common ways people locate IPs—and why it's important to use this knowledge responsibly.
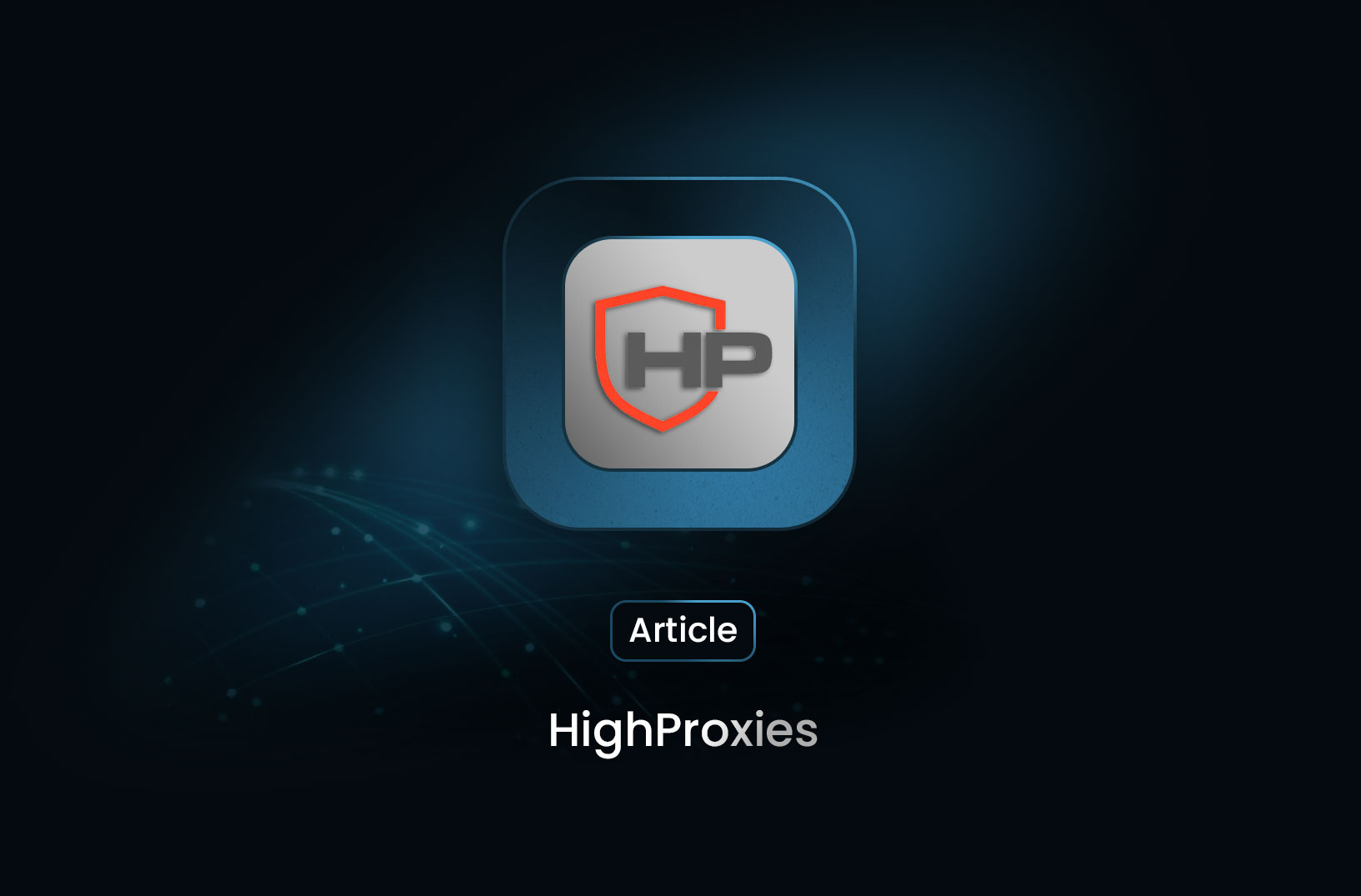
HighProxies: What They Are and When to Use Them
Discover how HighProxies can help with web scraping, social media automation, sneaker bots, and more. Learn when to use dedicated proxies for better online performance.
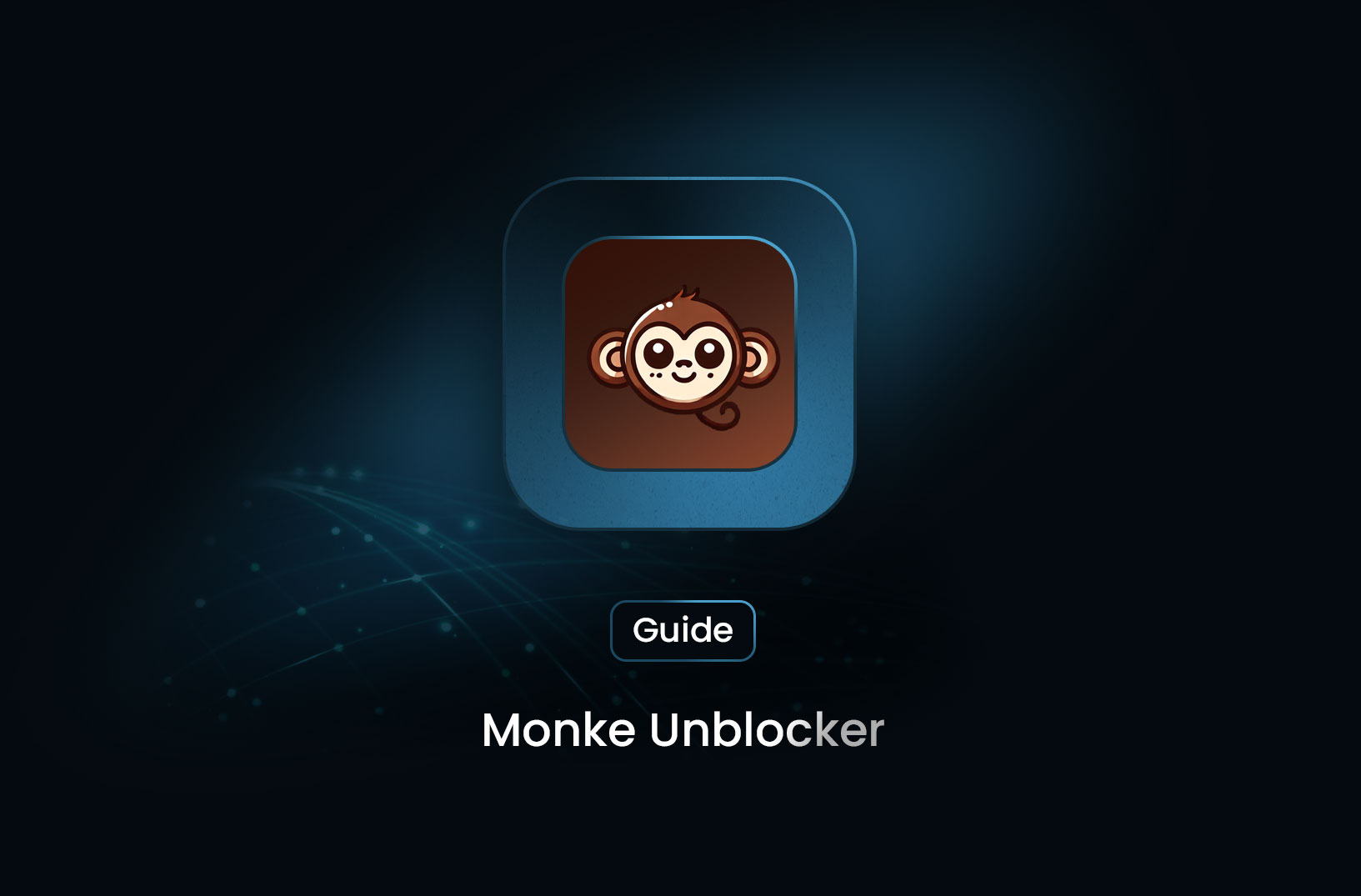
Monke Unblocker: Bypass School Restrictions on Chromebooks
Discover how Monke Unblocker enables students to bypass school network restrictions on Chromebooks, offering secure access to educational and entertainment websites.
@MrScraper_
@MrScraper