YouTube Channel Crawler
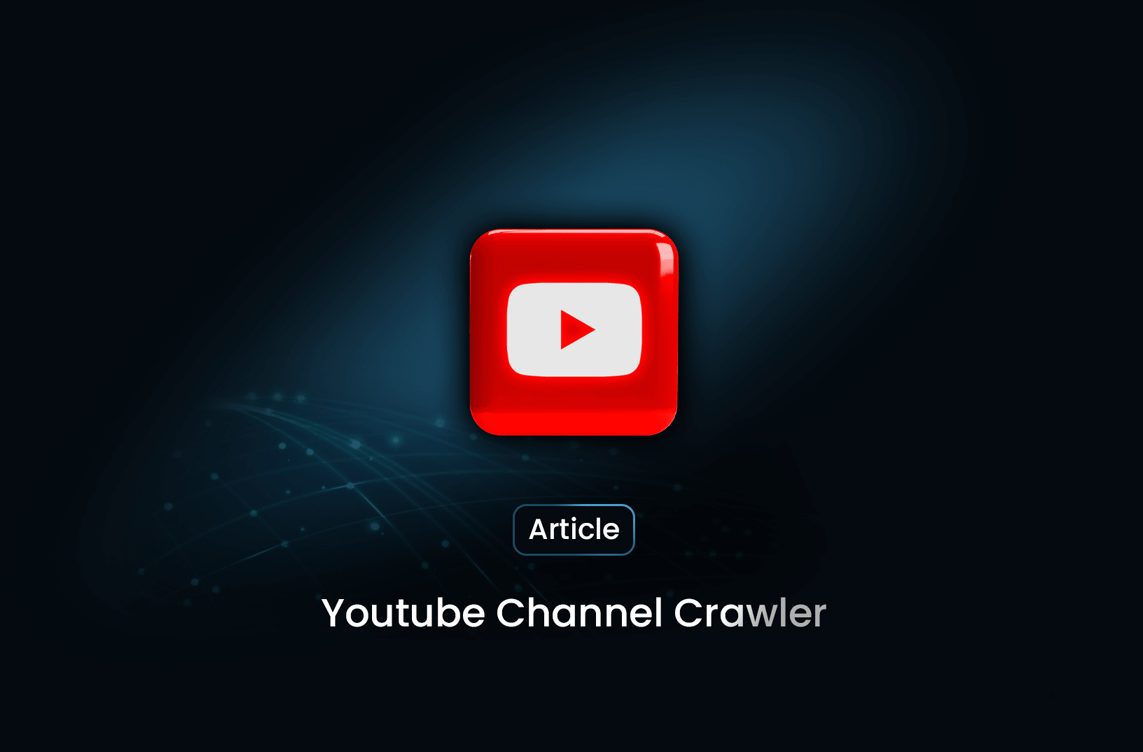
Web scraping and crawling YouTube channels can help you gather valuable insights such as video metadata, channel statistics, and more. This guide explains how to create a YouTube channel crawler using Python, complete with a practical use case and beginner-friendly technical steps.
What is a YouTube Channel Crawler?
A YouTube channel crawler is a tool that automatically collects data from YouTube channels. It can extract information like video titles, descriptions, upload dates, views, likes, and comments, enabling efficient data analysis or research.
Why Create a YouTube Channel Crawler?
- Market Research: Analyze trends, popular topics, or competitor channels.
- Content Insights: Collect data to improve your content strategy.
- Automation: Save time by automating data extraction from multiple channels.
Use Case: Analyzing Competitor Channels
Suppose you want to analyze competitors’ YouTube channels to understand their most engaging content. By crawling their channels, you can extract video details and identify patterns like popular topics or posting frequency.
Beginner-Friendly Steps to Create a YouTube Channel Crawler
Step 1: Install Required Libraries
You’ll need the google-api-python-client
library to interact with the YouTube Data API. Install it using:
pip install google-api-python-client
Step 2: Obtain a YouTube Data API Key
- Go to the Google Cloud Console.
- Create a new project.
- Enable the YouTube Data API v3 for your project.
- Generate an API key.
3: Set Up the Python Script
Import the necessary modules and configure your API key:
from googleapiclient.discovery import build
API_KEY = "YOUR_YOUTUBE_API_KEY"
YOUTUBE = build("youtube", "v3", developerKey=API_KEY)
Step 4: Retrieve Channel Information
Use the YouTube Data API to fetch channel details:
def get_channel_videos(channel_id):
request = YOUTUBE.search().list(
part="snippet",
channelId=channel_id,
maxResults=50,
order="date"
)
response = request.execute()
videos = []
for item in response.get("items", []):
video = {
"title": item["snippet"]["title"],
"videoId": item["id"].get("videoId"),
"publishedAt": item["snippet"]["publishedAt"]
}
videos.append(video)
return videos
# Example: Fetch videos from a specific channel
channel_id = "UC_x5XG1OV2P6uZZ5FSM9Ttw" # Example channel ID
videos = get_channel_videos(channel_id)
Step 5: Save the Data
Store the retrieved data in a JSON file:
import json
with open("youtube_channel_videos.json", "w") as file:
json.dump(videos, file, indent=4)
Step 6: Analyze the Data
Print the video details for analysis:
for video in videos:
print(f"Title: {video['title']}, Video ID: {video['videoId']}, Published At: {video['publishedAt']}")
Best Practices
- Respect API Limits: The YouTube Data API has usage quotas. Avoid making excessive requests.
- Handle Errors Gracefully: Use try-except blocks to handle API errors or timeouts.
- Follow YouTube Policies: Ensure compliance with YouTube’s terms of service.
Conclusion
Creating a YouTube channel crawler using Python enables you to automate the extraction of valuable channel and video data. By following this guide, you can analyze competitors, track trends, or optimize your content strategy effectively. Always respect YouTube’s policies and use the data responsibly.
Table of Contents
Take a Taste of Easy Scraping!
Get started now!
Step up your web scraping
Find more insights here
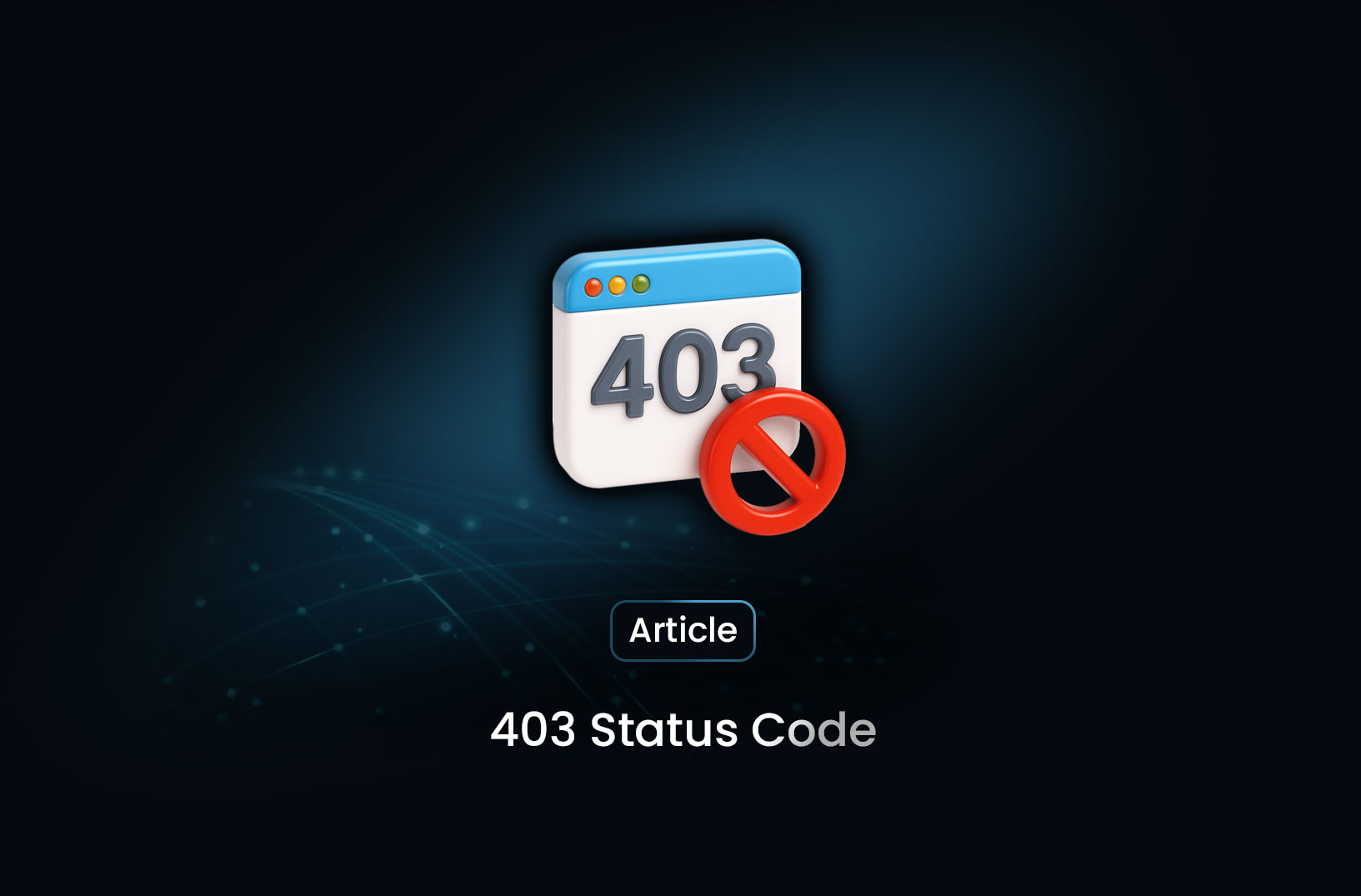
403 Status Code: Meaning, Causes, and Solutions
403 Forbidden status means the server received a valid request but refuses to process it due to insufficient permissions.
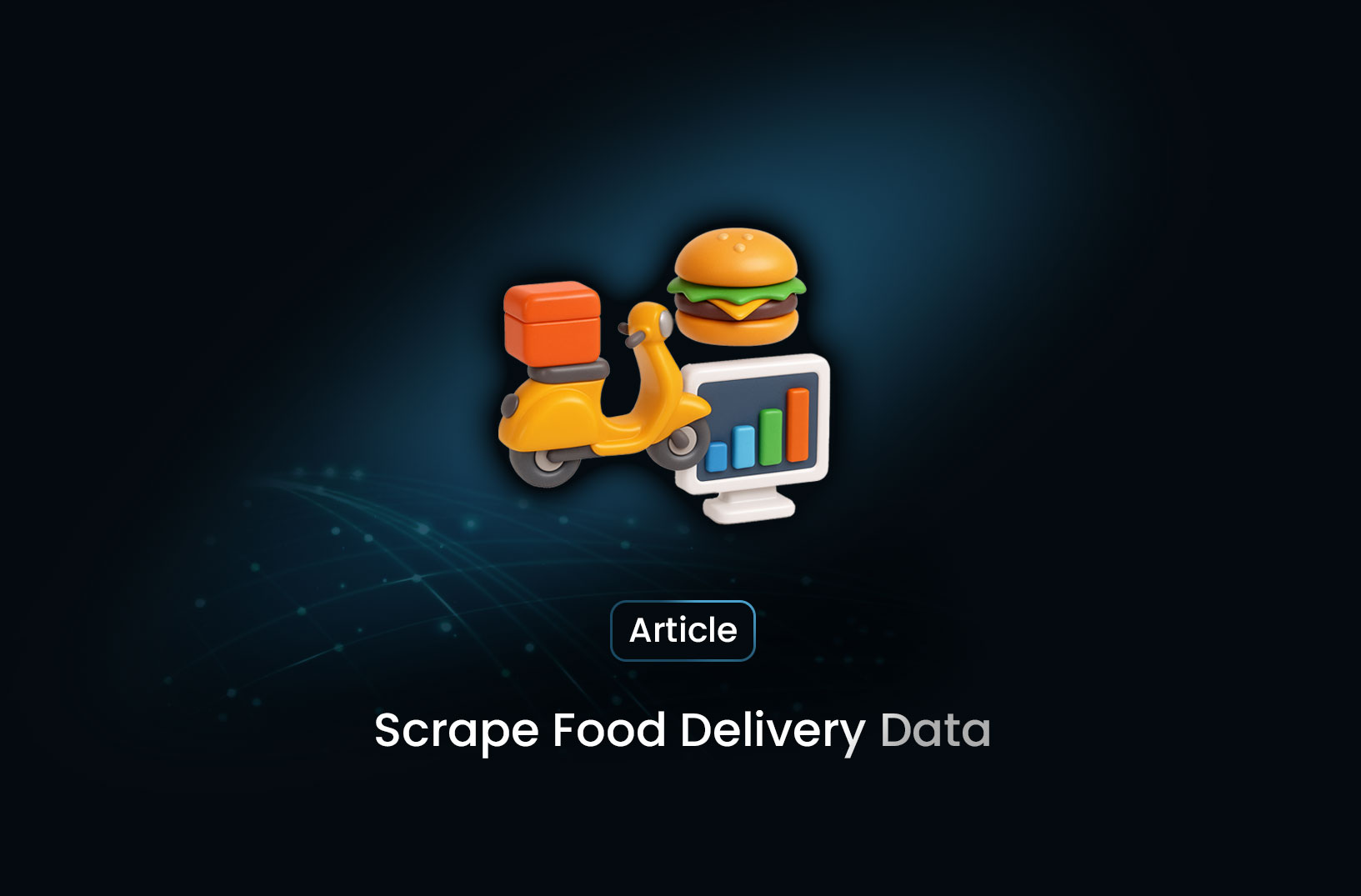
How to Scrape Food Delivery Data for Competitive Intelligence
Learn how to scrape food delivery data from platforms like ShopeeFood, GrabFood, and GoFood to uncover pricing trends, customer reviews, and market insights. Discover the tools, challenges, and how MrScraper can simplify the process.
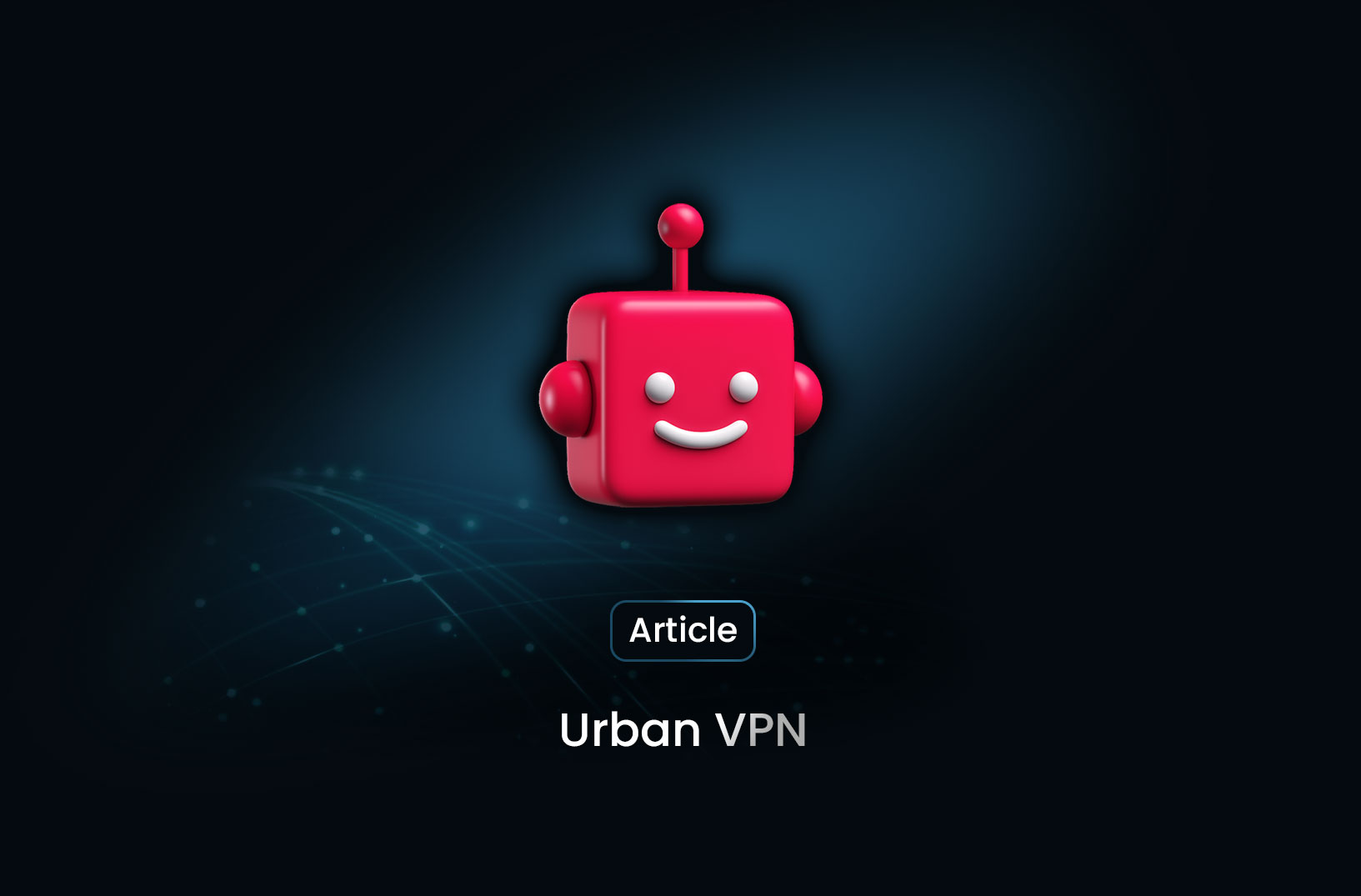
Urban VPN: A Free VPN Worth Using?
Urban VPN is a 100% free VPN with global server options and unlimited bandwidth—but its usability comes with significant trade-offs.
@MrScraper_
@MrScraper