Using XPath in Selenium: A Technical Guide
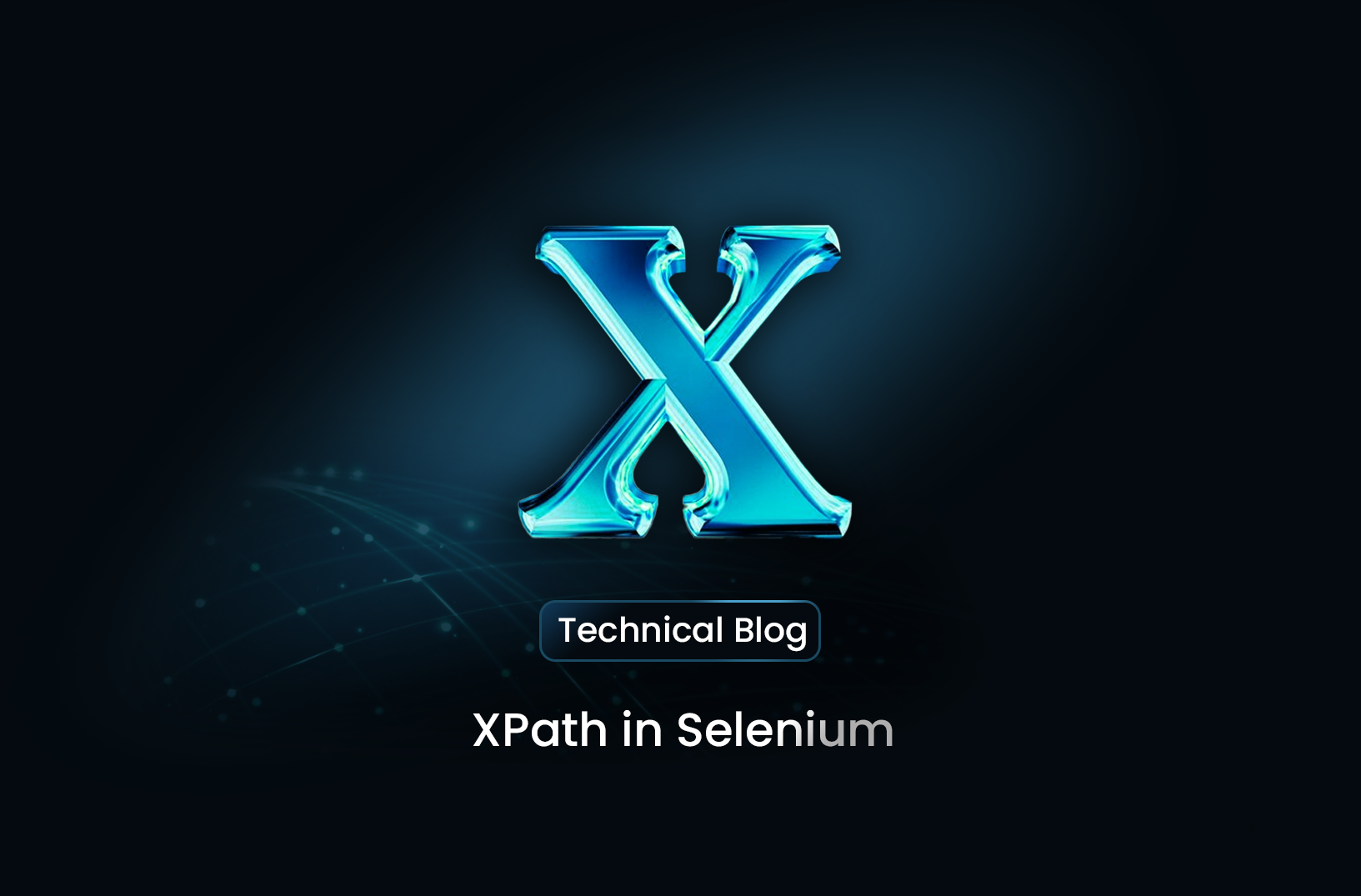
XPath (XML Path Language) is a powerful tool for navigating XML documents and HTML elements. In Selenium, XPath is one of the most common locators for finding and interacting with elements on a web page. This guide dives into the technical aspects of using XPath with Selenium, covering syntax, examples, and best practices to make your automated tests more efficient and reliable.
What is XPath?
XPath is a query language for selecting nodes from an XML or HTML document. It allows for navigation through elements and attributes in XML and HTML structures using path expressions. This makes XPath highly suitable for web scraping and browser automation tasks, as it can locate elements in a document with complex structures.
Why Use XPath in Selenium?
XPath is versatile in locating elements and supports:
- Access to elements using attributes, hierarchies, and relationships
- Searching for elements based on text content
- Complex query capabilities, such as filtering and sibling relations
Basic XPath Syntax
XPath expressions are composed of steps and functions. Here are common types:
- Absolute XPath:
/html/body/div[1]/div[2]/span
- Finds an element from the document root (not recommended for dynamic pages).
- Relative XPath:
//div[@class='container']
- Starts searching from anywhere in the document. This is more flexible and reliable.
- XPath with Attributes:
//*[@id='submit']
- Locates elements using their attributes.
- XPath with Text:
//button[text()='Submit']
- Finds elements by their exact text.
Advanced XPath Functions
XPath supports many functions that make it more powerful than other locators:
- Contains:
//div[contains(@class, 'header')]
- Finds elements with a partial match of an attribute.
- Starts-with:
//input[starts-with(@id, 'user')]
- Selects elements where an attribute value starts with specific text.
- Text Contains:
//*[contains(text(), 'Login')]
- Searches for elements containing specific text.
- Position and Index:
//div[position()=2]
- Selects elements based on their position among siblings.
- Logical Operators:
//input[@type='text' and @name='email']
- Allows combining conditions using
and/or
for finer control.
- Allows combining conditions using
How to Use XPath in Selenium with Python
First, ensure you have Selenium installed:
pip install selenium
Then, import the required modules and set up a simple script:
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.chrome.service import Service
# Initialize the WebDriver (replace with your WebDriver path)
driver = webdriver.Chrome(service=Service('path/to/chromedriver'))
# Navigate to the webpage
driver.get('https://example.com')
# Example XPath selections
element = driver.find_element(By.XPATH, "//div[@class='header']")
text_element = driver.find_element(By.XPATH, "//p[contains(text(), 'Welcome')]")
# Interact with the elements
element.click()
print(text_element.text)
# Close the browser
driver.quit()
Practical Examples and Use Cases
- Finding Buttons by Text Use this to click a button with specific text:
button = driver.find_element(By.XPATH, "//button[text()='Submit']")
button.click()
- Locating Form Fields by Partial Attribute Match
To locate form fields with similar
id
attributes:
username_field = driver.find_element(By.XPATH, "//input[contains(@id, 'user')]")
- Selecting Elements Based on Hierarchy Useful for selecting elements within nested structures:
nested_element = driver.find_element(By.XPATH, "//div[@class='parent']//span[@class='child']")
- Filtering with Logical Conditions Select elements matching multiple conditions:
filtered_element = driver.find_element(By.XPATH, "//input[@type='text' and @name='email']")
Best Practices for XPath in Selenium
-
Prefer Relative XPaths Absolute paths are fragile. Use relative paths like ‘//div[@class='content']’ to make scripts resilient to layout changes.
-
Use Unique Identifiers If elements have unique ‘id’ attributes, always prefer them for better performance.
-
Avoid Overly Complex XPaths Avoid chaining multiple conditions in one XPath expression; they are harder to maintain and may slow down execution.
-
Optimize for Readability and Maintainability Write XPaths that are clear and easy to understand, especially when working in teams.
-
Use contains Wisely While
contains
is powerful, overusing it can lead to unreliable tests. Combine it with other filters for accuracy.
Conclusion
XPath is a robust tool within Selenium for locating and interacting with web elements. By understanding XPath functions and best practices, you can write effective, maintainable, and powerful test scripts. The examples above provide a starting point for using XPath in Selenium with Python. Be sure to optimize your XPaths and test them to ensure consistent results across different web environments.
Table of Contents
Take a Taste of Easy Scraping!
Get started now!
Step up your web scraping
Find more insights here
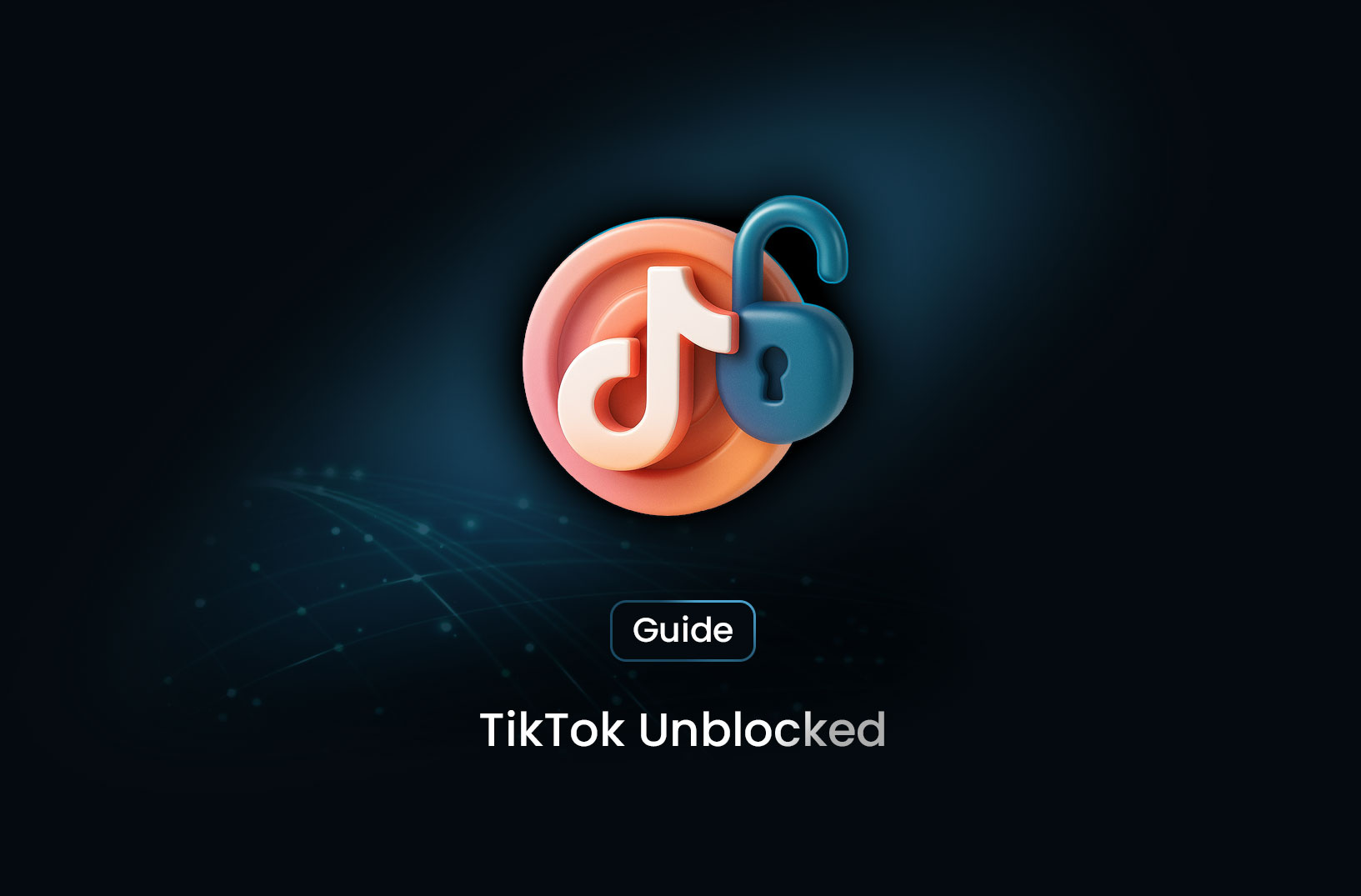
How to Access TikTok Unblocked: A Complete Guide
TikTok continues to face restrictions in various regions due to government bans, network policies, or institutional firewalls. Whether you're a student, traveler, or reside in a country where TikTok is inaccessible, this guide provides effective methods to unblock TikTok and enjoy uninterrupted access.
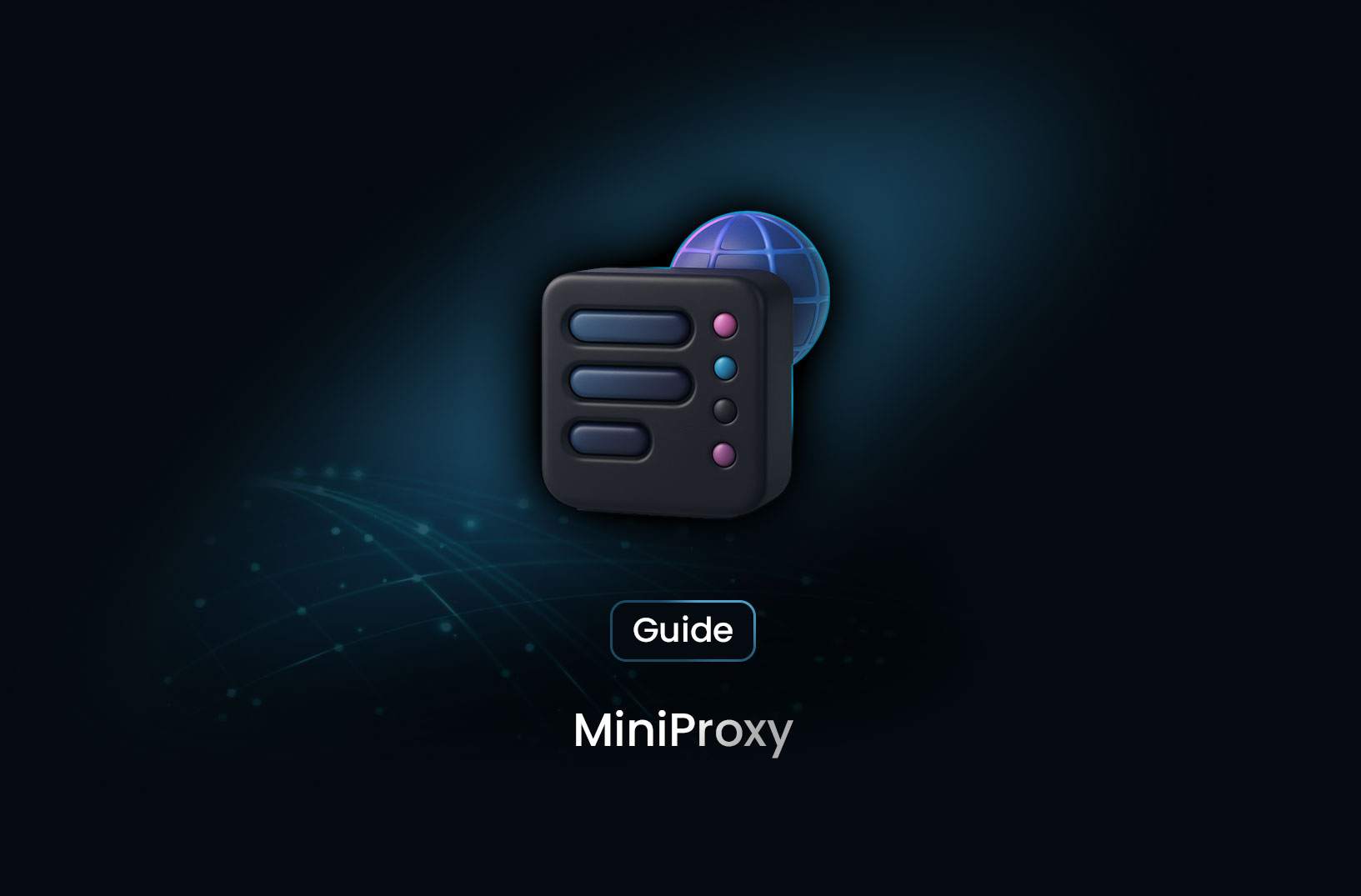
MiniProxy: How This Tool Helps You Bypass Web Restrictions
MiniProxy is a free, open-source web proxy written in PHP. It allows users to access websites through a server, effectively hiding the user's IP address and helping bypass network or geographical restrictions.
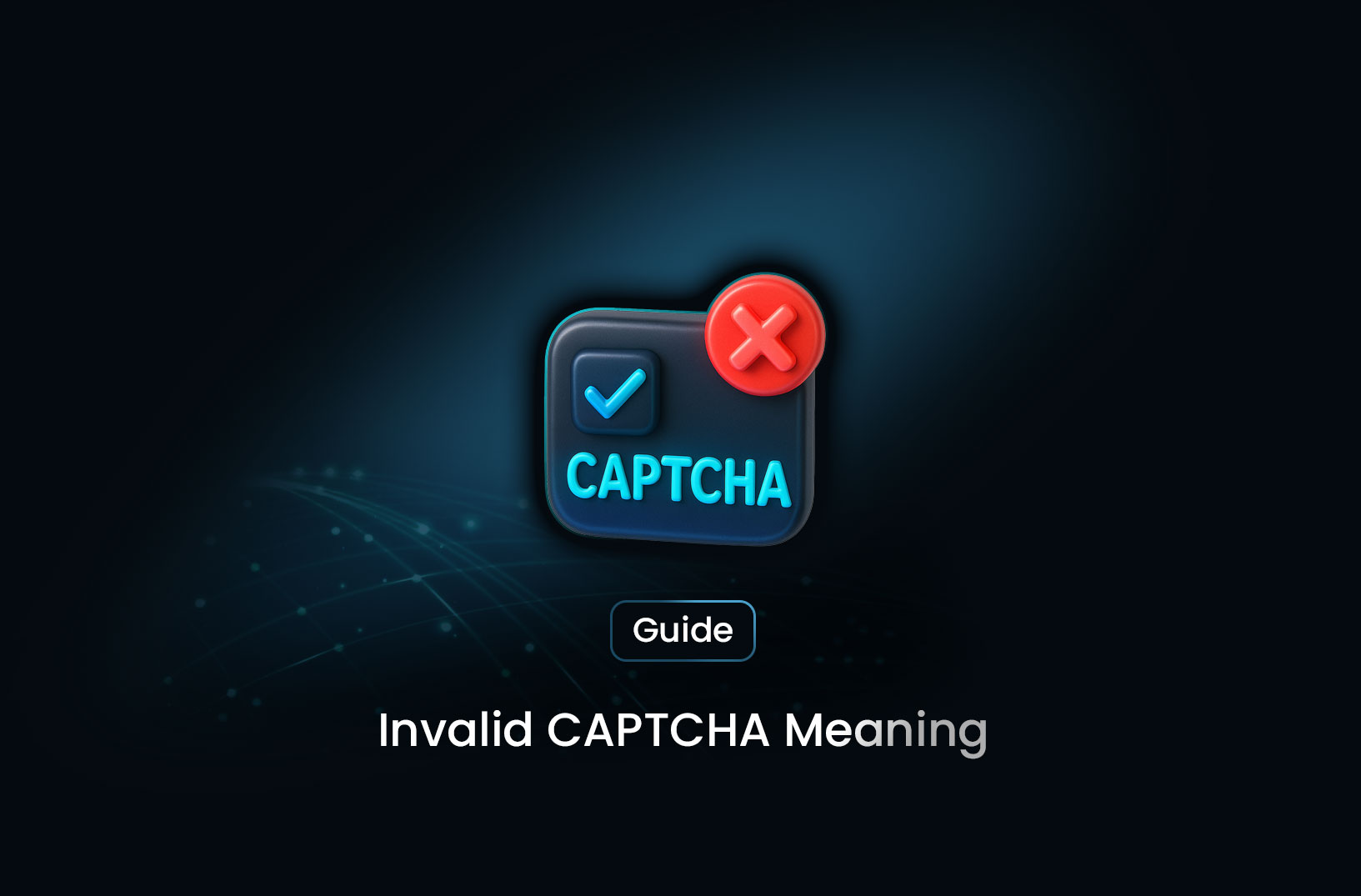
Invalid CAPTCHA Meaning: What It Is and How to Fix It
A CAPTCHA (Completely Automated Public Turing test to tell Computers and Humans Apart) is a security feature designed to differentiate between human users and automated bots.
@MrScraper_
@MrScraper