Use Case and Guide in Website Logging
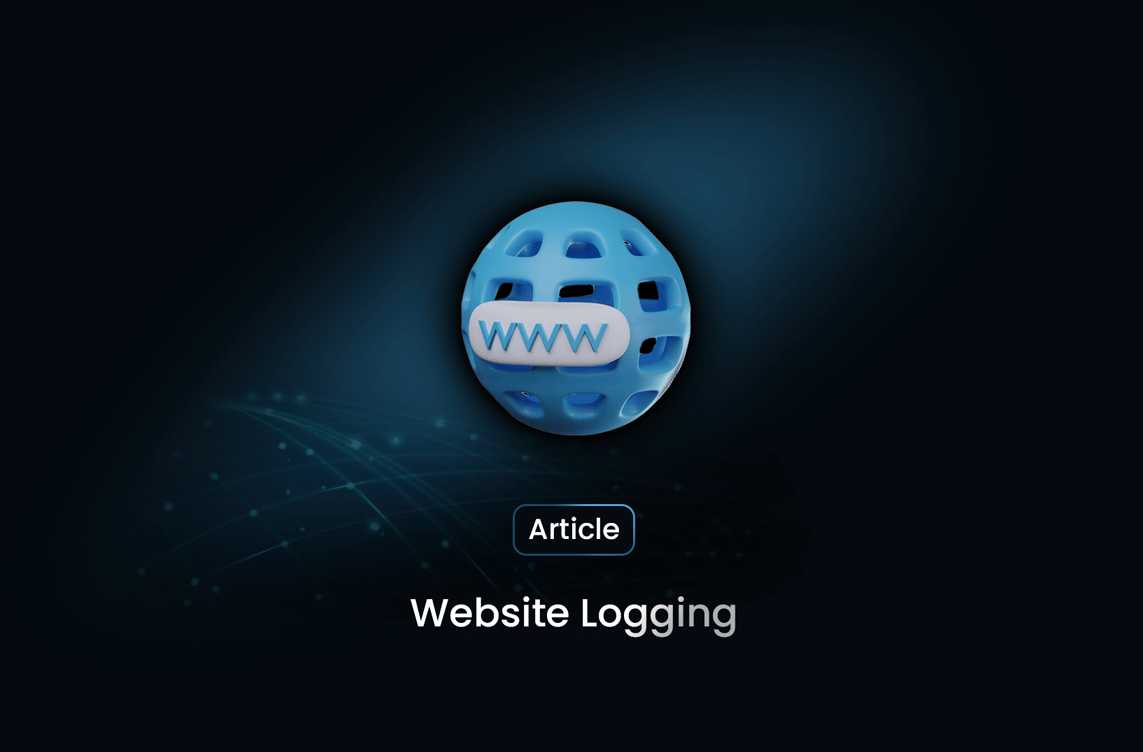
What is Website Logging?
Website logging refers to the process of automating login operations on a website using tools like Puppeteer, Playwright, or other web automation frameworks. It involves interacting with website elements such as login forms, buttons, and CAPTCHA challenges programmatically.
Why is Website Logging Important?
- Data Automation: Enables automated workflows that require user authentication.
- Testing: Facilitates automated testing of authentication features.
- Monitoring: Provides access to user-restricted pages for monitoring updates or changes.
- Scalability: Handles multiple logins efficiently in scenarios like API testing or scraping with multiple accounts.
Use Case: Automating Login to an E-commerce Website
Imagine you want to scrape product data from a website that requires user authentication. Automating the login process allows your scraper to access restricted pages programmatically.
Technical Guide to Automate Website Logging
Tools and Setup
- Node.js: Install the latest stable version.
- Puppeteer or Playwright: Choose one based on your preference.
Dependencies
- To install Puppeteer:
npm install puppeteer
- To install Playwright:
npm install playwright
Step-by-Step Implementation
1. Initialize the Project Create a new directory and initialize a Node.js project:
mkdir website-logging
cd website-logging
npm init -y
Install Puppeteer or Playwright:
npm install puppeteer
# or
npm install playwright
2. Write the Login Script Below is an example using Puppeteer to log in to a website:
const puppeteer = require('puppeteer');
(async () => {
// Launch the browser
const browser = await puppeteer.launch({ headless: false });
const page = await browser.newPage();
// Navigate to the login page
await page.goto('https://example.com/login');
// Fill in the username and password
await page.type('#username', 'your-username');
await page.type('#password', 'your-password');
// Click the login button
await page.click('#login-button');
// Wait for navigation to the dashboard or next page
await page.waitForNavigation();
// Verify login success (e.g., check URL or element presence)
const loggedIn = await page.evaluate(() => {
return document.querySelector('h1.dashboard-title') !== null;
});
console.log(`Login ${loggedIn ? 'successful' : 'failed'}`);
// Close the browser
await browser.close();
})();
3. Handle CAPTCHA Challenges (Optional) If the website uses CAPTCHA, integrate services like AntiCaptcha or CapSolver to solve it programmatically. For example:
const solveCaptcha = async (page) => {
// Use an external CAPTCHA-solving service here
};
await solveCaptcha(page);
4. Save Cookies for Session Reuse To avoid logging in repeatedly, save session cookies:
const cookies = await page.cookies();
const fs = require('fs');
fs.writeFileSync('cookies.json', JSON.stringify(cookies));
// To reuse cookies
const savedCookies = JSON.parse(fs.readFileSync('cookies.json'));
await page.setCookie(...savedCookies);
Best Practices
- Use Headless Mode Cautiously: Some websites block headless browsers.
- Rotate User Agents: Mimic different browsers to avoid detection.
- Delay Actions: Introduce delays to simulate human behavior.
- Handle Errors Gracefully: Implement robust error-handling mechanisms.
Conclusion
Website logging is an essential technique for tasks requiring authenticated access to web content. Using tools like Puppeteer or Playwright, you can automate this process efficiently. By following the steps outlined in this guide, you can implement a robust solution for logging into websites programmatically.
For more advanced scenarios, such as handling multi-factor authentication (MFA) or API-based login flows, consider exploring the documentation of your chosen web automation framework.
Table of Contents
Take a Taste of Easy Scraping!
Get started now!
Step up your web scraping
Find more insights here
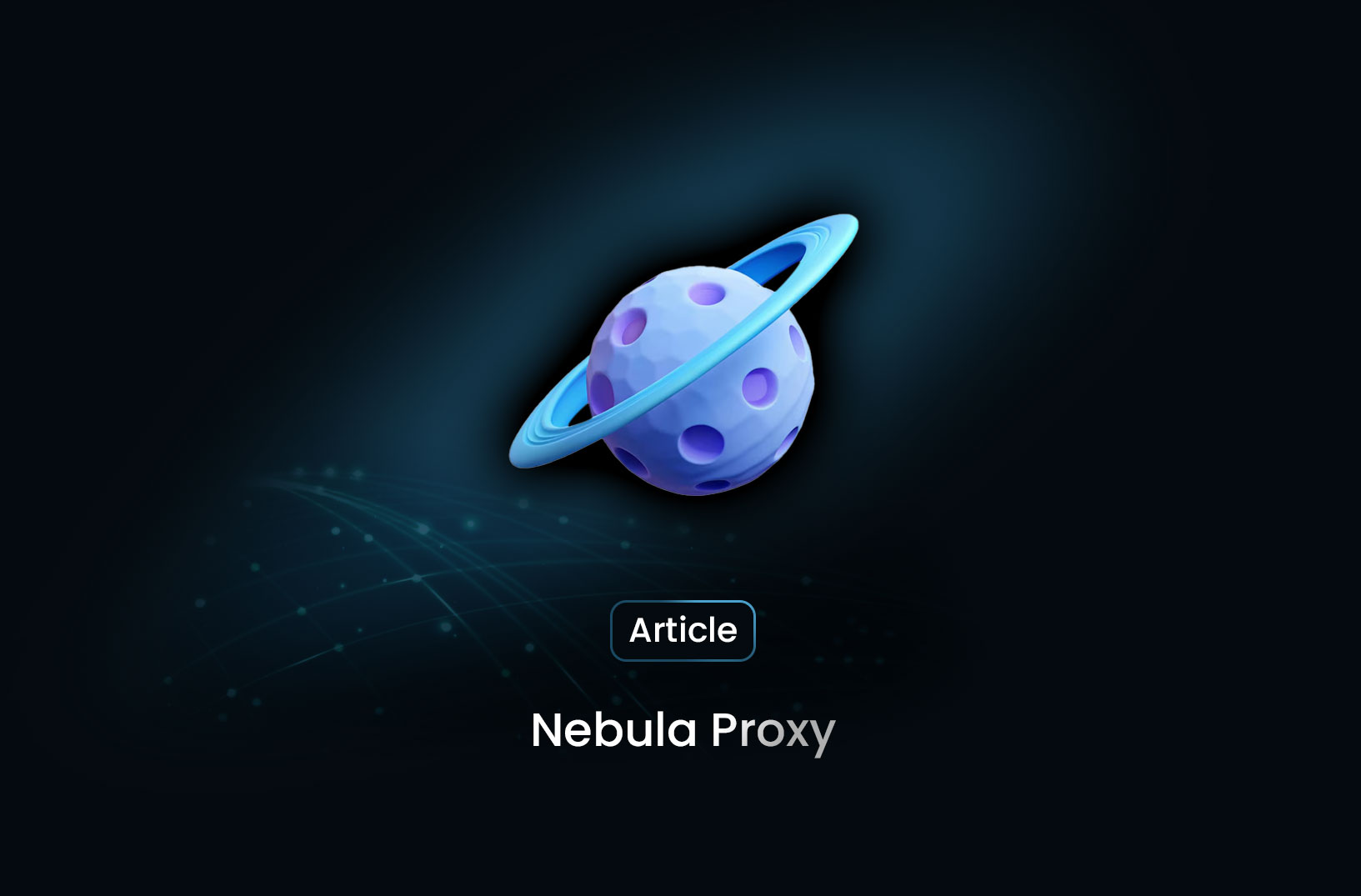
What is Nebula Proxy? A Powerful Tool for Web Scraping, SEO, and Online Privacy
Discover how Nebula Proxy enhances web scraping, SEO monitoring, and online privacy. Learn about its features, use cases, and setup, plus top alternatives for seamless data extraction and secure browsing.
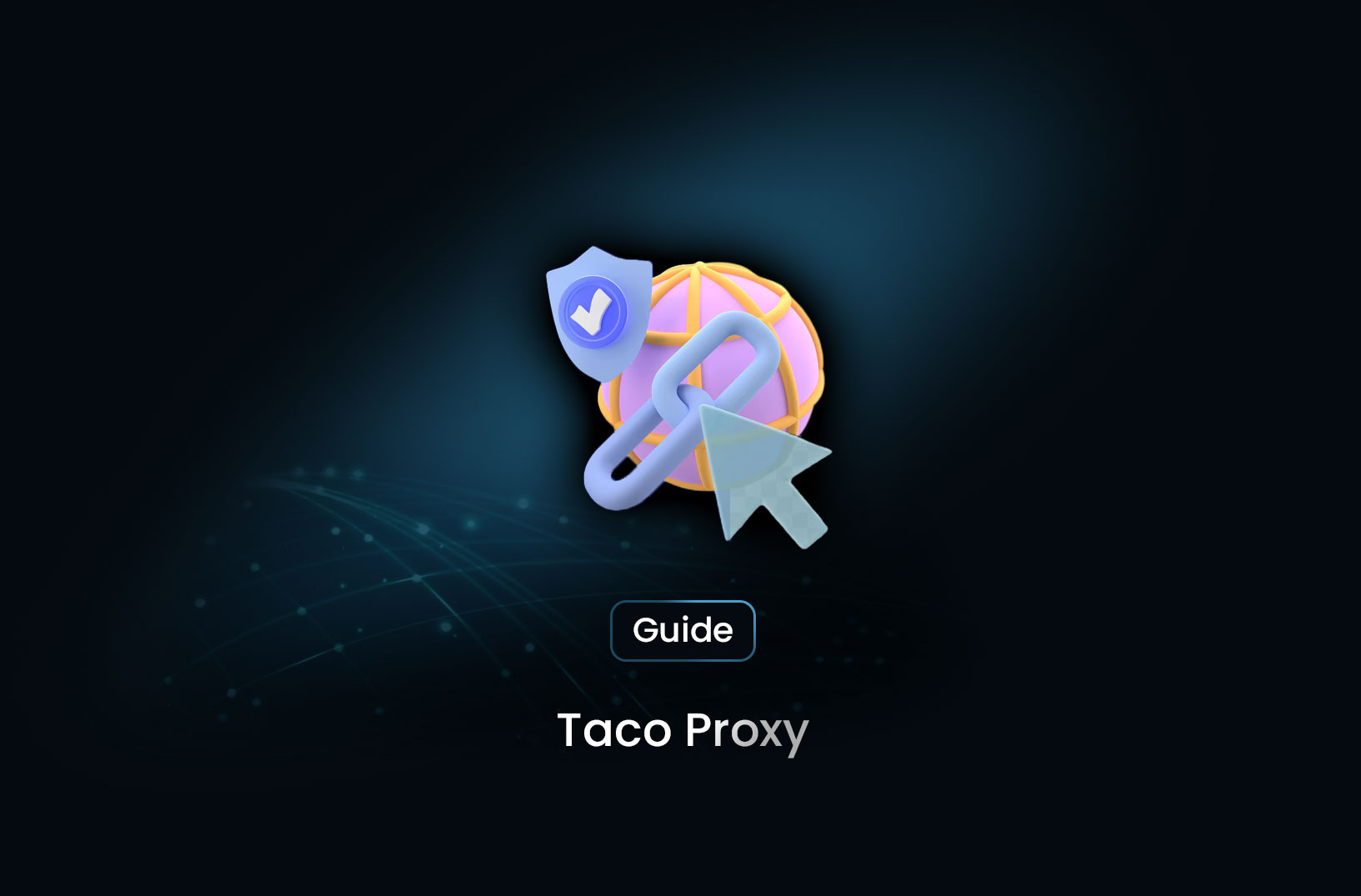
Taco Proxy: Understanding Its Role and Use Cases
Learn what Taco Proxy is, how it works, and its key use cases for web scraping, SEO monitoring, cybersecurity, and bypassing geo-restrictions. Get step-by-step proxy configuration guides for Python, Scrapy, and cURL.
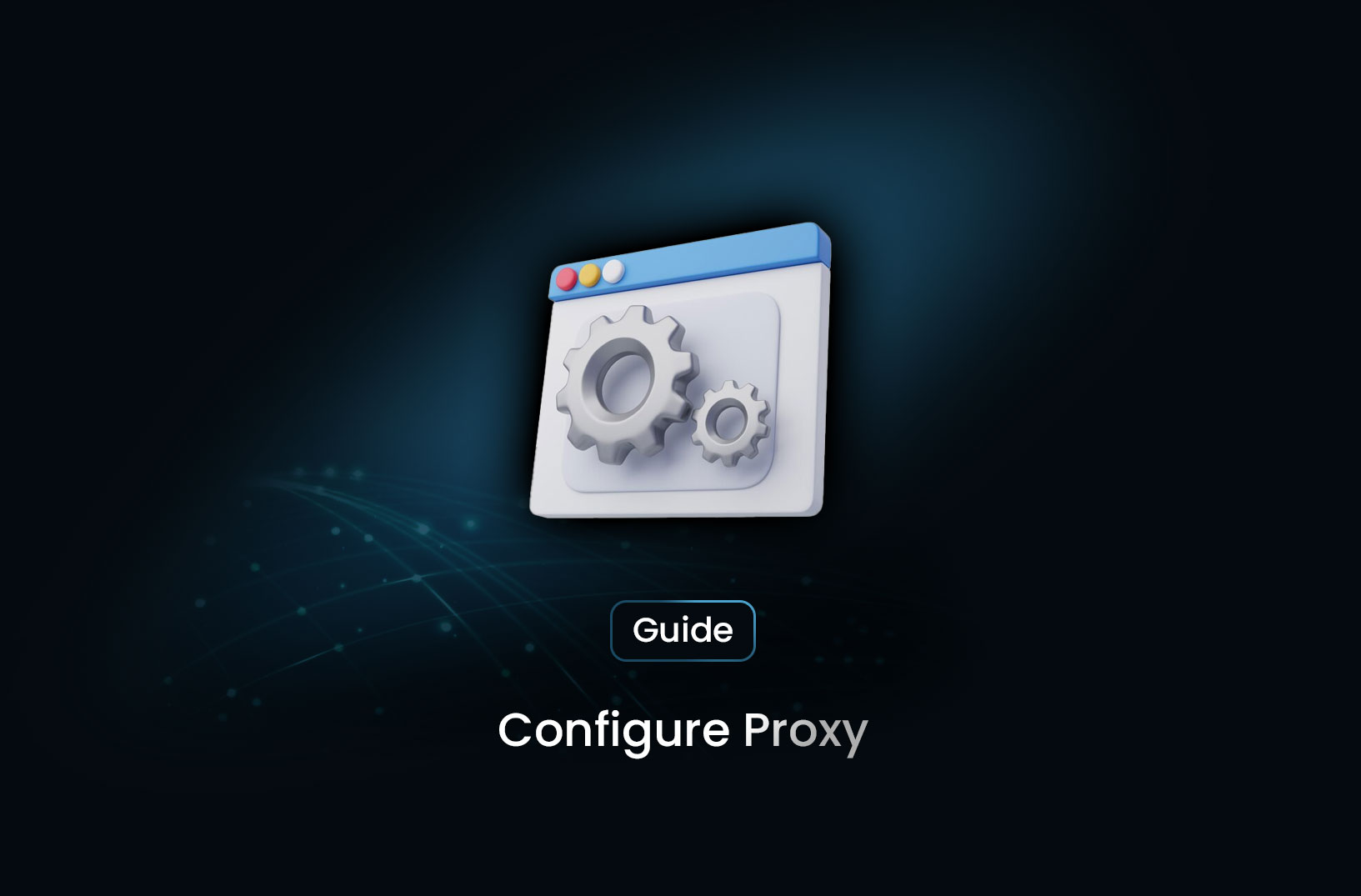
How to Configure Proxy
Learn how to set up a proxy on Windows, macOS, Linux, browsers, and command-line tools like cURL and Python.
@MrScraper_
@MrScraper