Understanding "Scroll Down" in Web Scraping
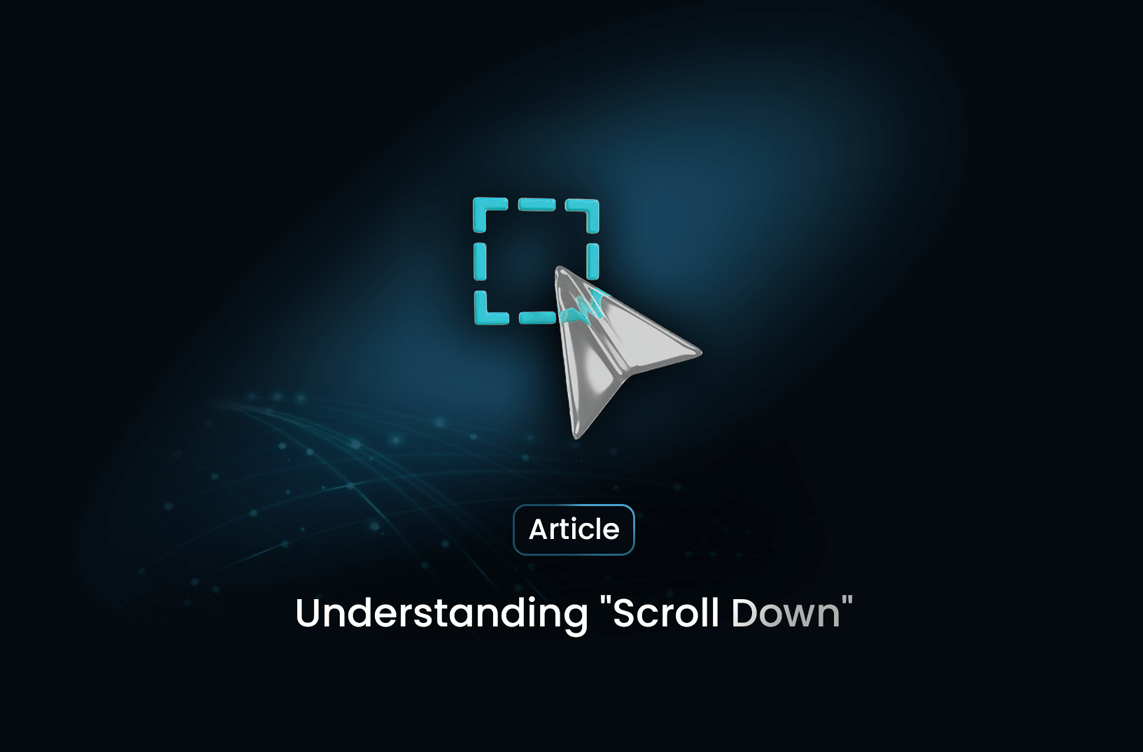
In the realm of web scraping, "scrolling down" means navigating to the bottom of a webpage to load more content dynamically. Many modern websites, like social media platforms or content-heavy sites, use techniques such as infinite scrolling or lazy loading, fetching data only as you scroll. If you're into web scraping, mastering this behavior is key to accessing all the data you need.
Before diving into the details, we’d like to share some good news: MrScraper handles pagination effortlessly, including scrolling down web pages. In this blog, we’ll guide you through how it’s done and share tips to help you scrape scrolling pages like a pro!
Why is Scrolling Down Important in Web Scraping?
When scraping websites with dynamic content, simply fetching the initial HTML of a page may not be enough. By scrolling down, you can:
- Load More Data: Access additional content that isn't loaded until the user interacts with the page.
- Improve Data Collection: Gather a more comprehensive dataset for analysis.
- Mimic User Behavior: Many sites have protections against automated scraping, and mimicking real user actions can help avoid detection.
Implementing Scroll Down in Code
When scraping, you can automate scrolling using libraries such as Selenium or Puppeteer. Below is an example of how to implement scrolling down using Puppeteer:
Example Code: Scrolling Down with Puppeteer
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto('https://example.com'); // Replace with your target URL
// Set the scroll delay
const scrollDelay = 1000; // Time in milliseconds
// Scroll down to the bottom of the page
await autoScroll(page, scrollDelay);
// Capture the page content after scrolling
const content = await page.content();
console.log(content); // Output the content for further processing
await browser.close();
})();
async function autoScroll(page, delay) {
await page.evaluate(async (delay) => {
await new Promise((resolve) => {
let totalHeight = 0;
const distance = 100;
const timer = setInterval(() => {
const scrollHeight = document.body.scrollHeight;
window.scrollBy(0, distance);
totalHeight += distance;
if (totalHeight >= scrollHeight) {
clearInterval(timer);
resolve();
}
}, delay);
});
}, delay);
}
Scraping from Scratch vs. Using MrScraper
Scraping from Scratch
- Time-Consuming: Building a web scraper from the ground up requires significant time investment.
- Complexity: Handling different page structures, managing cookies, sessions, and dealing with CAPTCHAs can be daunting.
- Maintenance: Constant updates and adjustments are needed to adapt to website changes.
Using MrScraper
- Ease of Use: MrScraper simplifies the scraping process with intuitive features and a user-friendly interface.
- Efficiency: Quickly set up scrapers without dealing with low-level code.
- Dynamic Loading: Built-in capabilities to handle scrolling down and dynamically loading content automatically.
- Support: Access to support and documentation tailored for users, helping you troubleshoot issues faster.
While you can certainly create your web scraper from scratch, using MrScraper offers numerous advantages that save you time, effort, and headaches. With built-in features for pagination, including scrolling down, you can focus on extracting valuable data rather than wrestling with code.
For effective and efficient web scraping, choose MrScraper and experience the difference!
Table of Contents
Take a Taste of Easy Scraping!
Get started now!
Step up your web scraping
Find more insights here
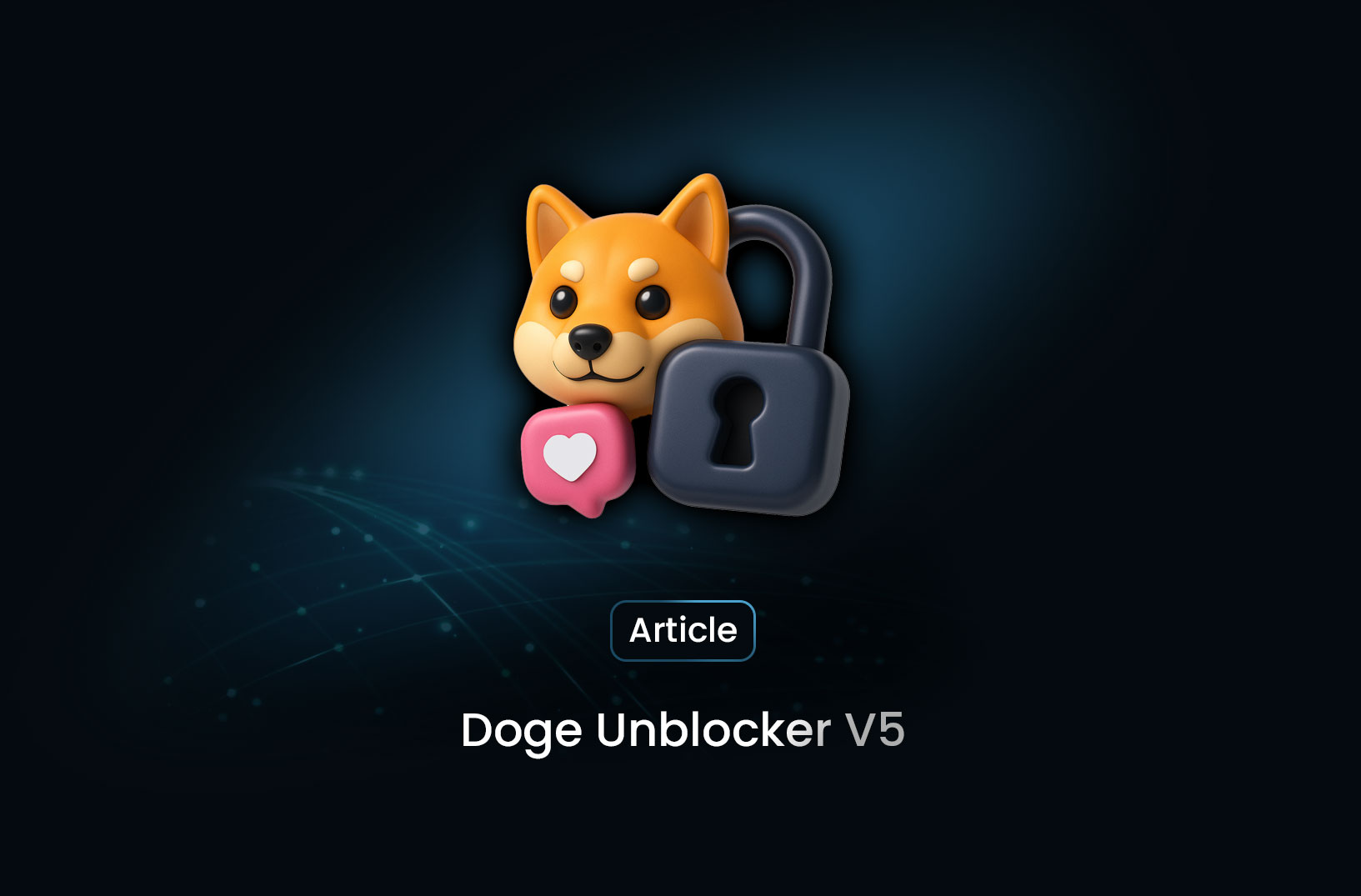
Doge Unblocker V5: Unlock the Web with Speed, Privacy, and Style
Doge Unblocker V5 is an open-source web proxy tool designed to bypass network restrictions and give users access to blocked websites and resources.
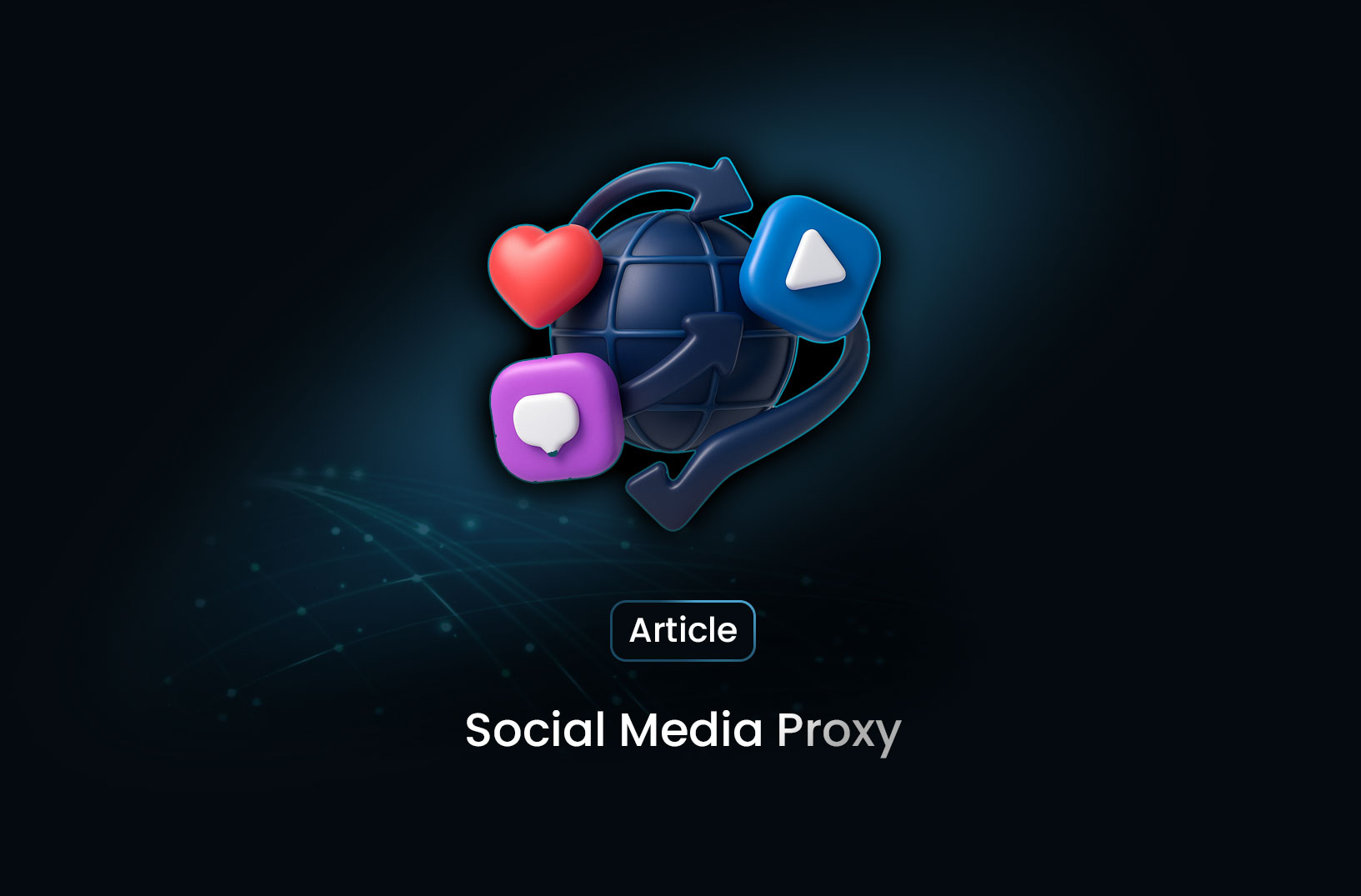
Social Media Proxy: The Easy Way to Manage Multiple Accounts
A social media proxy is an intermediary server that routes your internet connection through a different IP address.
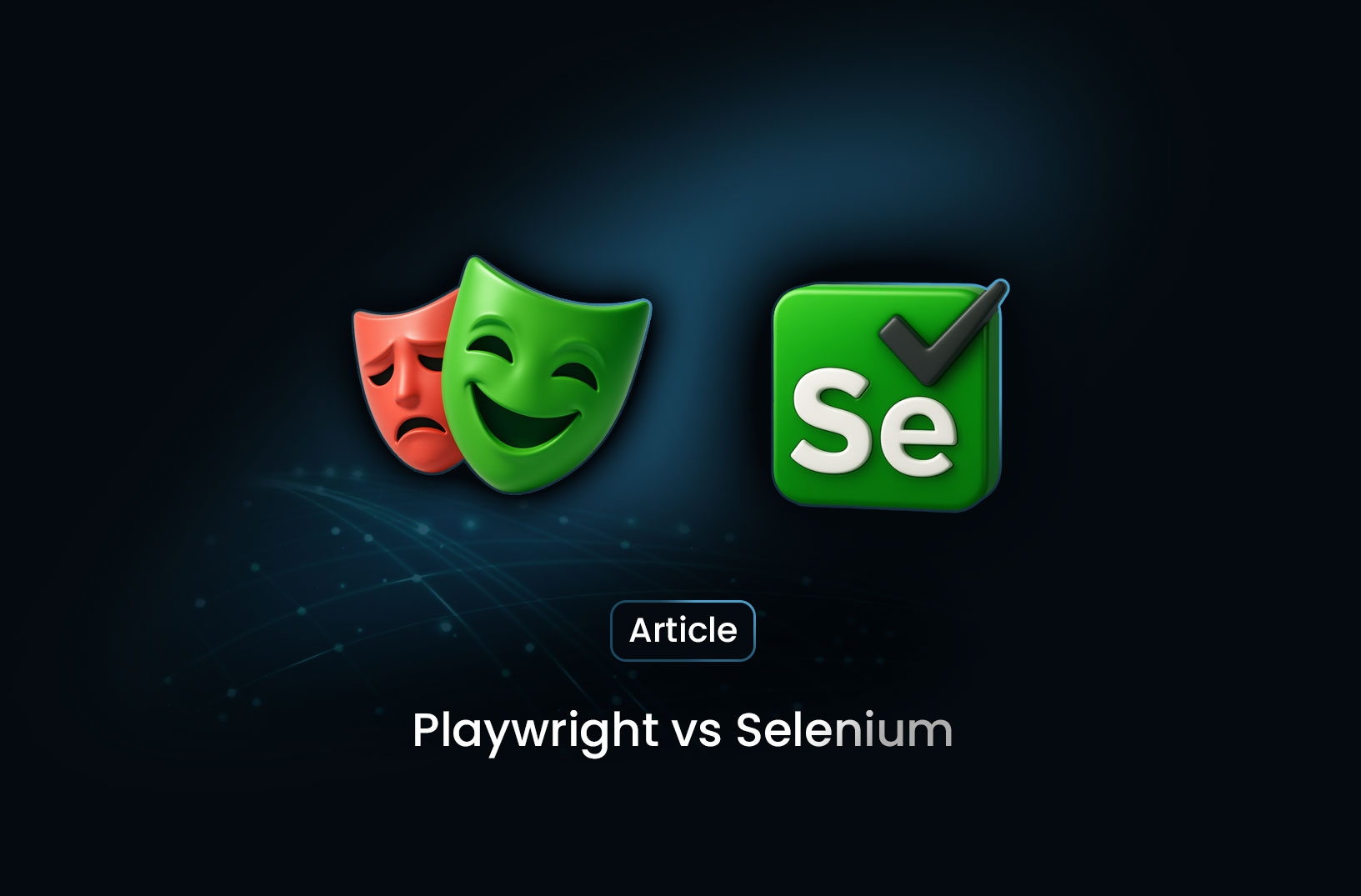
Playwright vs Selenium: Choosing the Right Tool for Web Automation in 2025
Selenium is An open-source framework that has been instrumental in automating web browsers. It supports multiple programming languages and a wide range of browsers, making it a versatile choice for many developers. While Playwright is developed by Microsoft, Playwright is a newer entrant in the automation space. It offers a unified API to automate Chromium, Firefox, and WebKit browsers, emphasizing speed, reliability, and modern web features.
@MrScraper_
@MrScraper