The Technical Guide to Google Scraping: Risks, Methods, and Best Practices
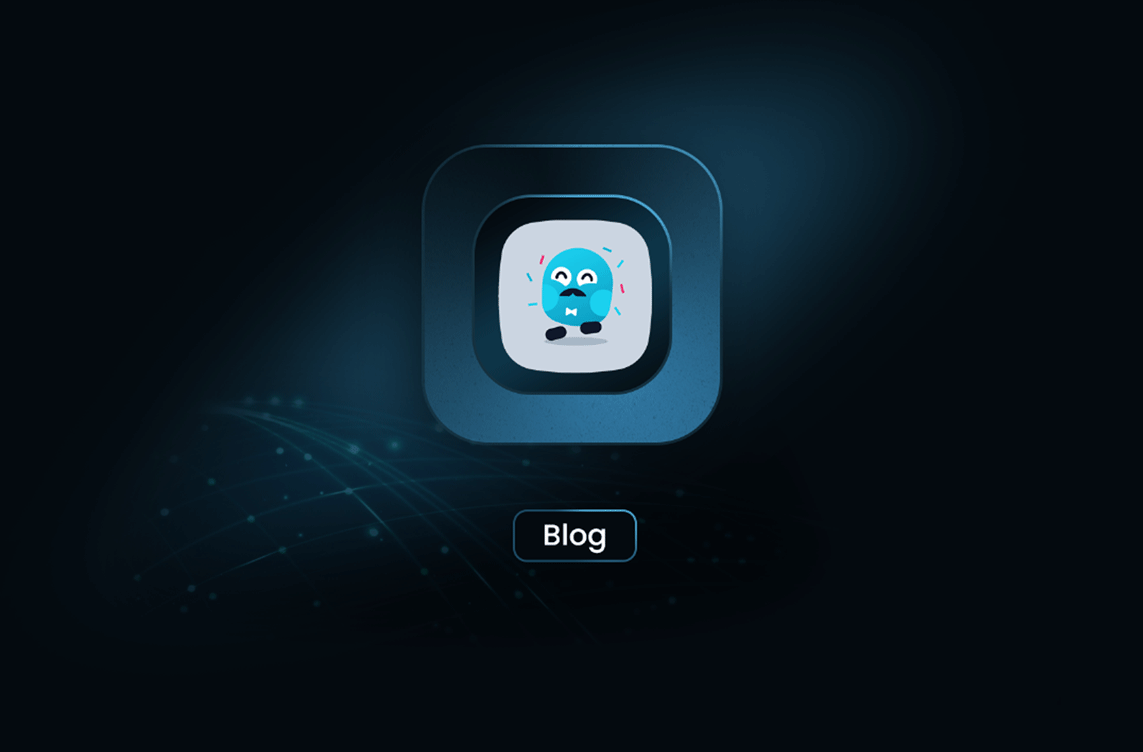
Google scraping involves extracting data from Google’s search results or platforms using automated tools. Common uses include:
- SEO analysis: Collecting SERP data.
- Market research: Tracking trends and competition.
- Data aggregation: Extracting business details from Google Maps.
Scraping Google can violate its terms of service, and it’s important to be aware of the legal implications. An alternative is to use Google’s official APIs.
How Google Scraping Works
Scraping Google involves sending HTTP requests to Google's servers and extracting data from the responses. Here are the key tools and languages:
- Python: Popular libraries like
BeautifulSoup
,Selenium
, orScrapy
. - Node.js: Tools like
Puppeteer
orCheerio
. - Browser Automation: Tools like Selenium and Puppeteer are used to handle JavaScript-heavy pages.
Step-by-Step Guide to Scraping Google
1. Setting up the Environment
You can install necessary libraries using pip:
pip install requests beautifulsoup4 selenium
For Puppeteer (Node.js):
npm install puppeteer
2. Sending Requests to Google
Use Python’s requests library to send an HTTP request with a custom User-Agent:
import requests
from bs4 import BeautifulSoup
headers = {'User-Agent': 'Mozilla/5.0'}
response = requests.get('https://www.google.com/search?q=python', headers=headers)
soup = BeautifulSoup(response.text, 'html.parser')
for result in soup.select('.tF2Cxc'):
title = result.select_one('.DKV0Md').get_text()
link = result.select_one('a')['href']
snippet = result.select_one('.aCOpRe').get_text()
print(title, link, snippet)
3. Parsing Google’s HTML
Use libraries like BeautifulSoup
to parse the returned HTML and extract the information you need:
for result in soup.select('.tF2Cxc'):
title = result.select_one('.DKV0Md').get_text()
link = result.select_one('a')['href']
snippet = result.select_one('.aCOpRe').get_text()
print(f"Title: {title}\nLink: {link}\nSnippet: {snippet}")
4. Avoiding Google’s Blocking Mechanisms
Google has anti-scraping measures like CAPTCHAs and IP blocking. To avoid this:
- Use Proxies: Rotate proxies with services like
ScraperAPI
orBright Data
. - Set Proper Headers: Randomize User-Agent strings, add referrers, and set random intervals between requests.
- Handle CAPTCHAs: Integrate CAPTCHA solving services or use headless browsers.
5. Scraping JavaScript-Heavy Pages with Selenium
To handle dynamic content, you can use Selenium:
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
driver = webdriver.Chrome()
driver.get("https://www.google.com/")
search_box = driver.find_element_by_name('q')
search_box.send_keys('python scraping')
search_box.send_keys(Keys.RETURN)
#Extract results after the page loads
results = driver.find_elements_by_css_selector('div.tF2Cxc')
for result in results:
print(result.text)
driver.quit()
Ethical Considerations and Legal Risks
- Legal Risks: Scraping Google can violate its terms of service, leading to blocked IPs or legal action.
- Ethical Practices: Follow robots.txt, scrape responsibly, and use APIs when available.
Using Google APIs as an Alternative
Instead of scraping, you can use Google’s Custom Search API for compliant data extraction:
import requests
API_KEY = 'your-api-key'
CX = 'your-custom-search-engine-id'
query = 'python scraping'
url = f"https://www.googleapis.com/customsearch/v1?q={query}&key={API_KEY}&cx={CX}"
response = requests.get(url)
data = response.json()
for item in data['items']:
print(item['title'], item['link'])
Best Practices for Web Scraping
- Rate Limiting: Avoid frequent requests to prevent being blocked. Introduce delays between requests.
- Rotating Proxies: Use proxy services to distribute traffic across multiple IP addresses.
- Error Handling: Handle timeouts, 404 errors, and CAPTCHAs effectively in your code.
Bonus Tips: Mrscraper’s Leads Generator API
While traditional Google scraping involves navigating complex challenges like CAPTCHAs, IP blocking, and ever-changing HTML structures, there’s a simpler and more effective solution for businesses looking to extract Google-based data: using an API like Mrscraper’s Leads Generator.
Why choose Mrscraper over manual scraping?
- Simplicity: Instead of writing complex code to scrape Google and deal with IP rotations, CAPTCHAs, and parsing, Mrscraper’s API allows you to retrieve data with just a few API calls. Example request to the Leads Generator endpoint:
curl -X POST "https://api.mrscraper.com/v1/leads/google" \
-H "Content-Type: application/json" \
-H "Authorization: Bearer YOUR_API_KEY" \
-d '{
"query": "business name",
"location": "city, state",
"filters": {"type": "local"}
}'
-
CAPTCHA-Free: Mrscraper handles CAPTCHA challenges behind the scenes, saving you from integrating third-party CAPTCHA solving services.
-
Reliable Data: Scraping Google results manually can lead to incomplete or inaccurate data due to frequent changes in the HTML structure. Mrscraper’s Leads Generator API ensures consistently accurate and well-formatted data.
-
Time-Saving: Building a Google scraping solution requires ongoing maintenance as Google frequently updates its UI and anti-scraping measures. With Mrscraper, you get continuous access to up-to-date data without the need for regular updates to your scraping scripts.
-
Scalable: Whether you need data from a few pages or thousands of records, the Leads Generator API can handle requests at scale, something that traditional scraping struggles with due to rate limits and IP bans.
In Summary:
- Google Scraping: Involves manual coding, proxy management, CAPTCHAs, and risk of blocking.
- Mrscraper API: Provides a streamlined, reliable, and hassle-free way to get structured data without the technical overhead.
For businesses that need quick, reliable, and scalable data from Google, Mrscraper’s Leads Generator is the perfect solution, offering an API that eliminates the headaches of traditional scraping.
Table of Contents
Take a Taste of Easy Scraping!
Get started now!
Step up your web scraping
Find more insights here
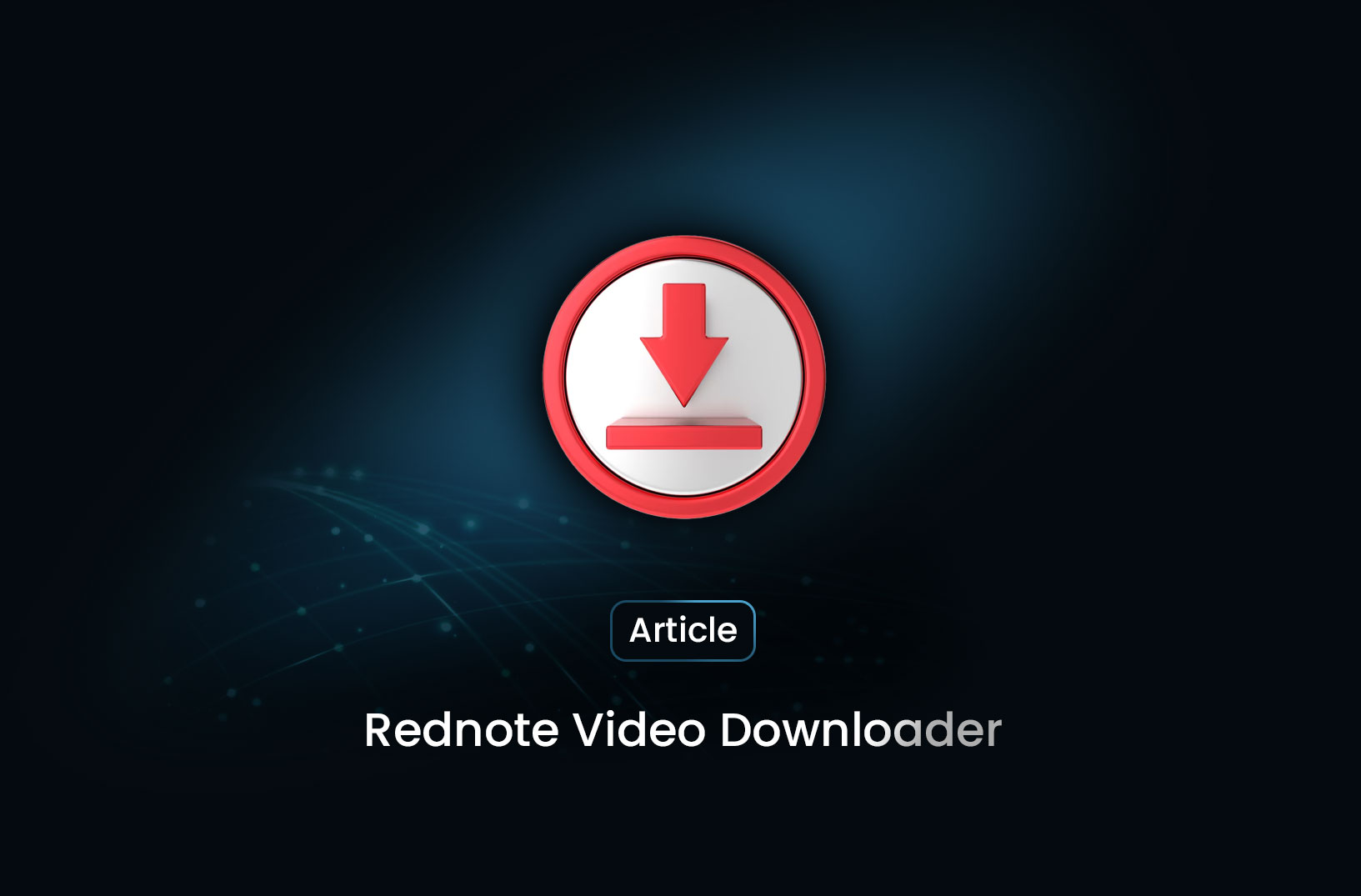
Rednote Video Downloader: All You Need to Know
Discover how to download videos from Rednote (Xiaohongshu) using apps, web tools, or API solutions. Learn how MrScraper offers a powerful and scalable way to scrape Rednote content easily and efficiently.
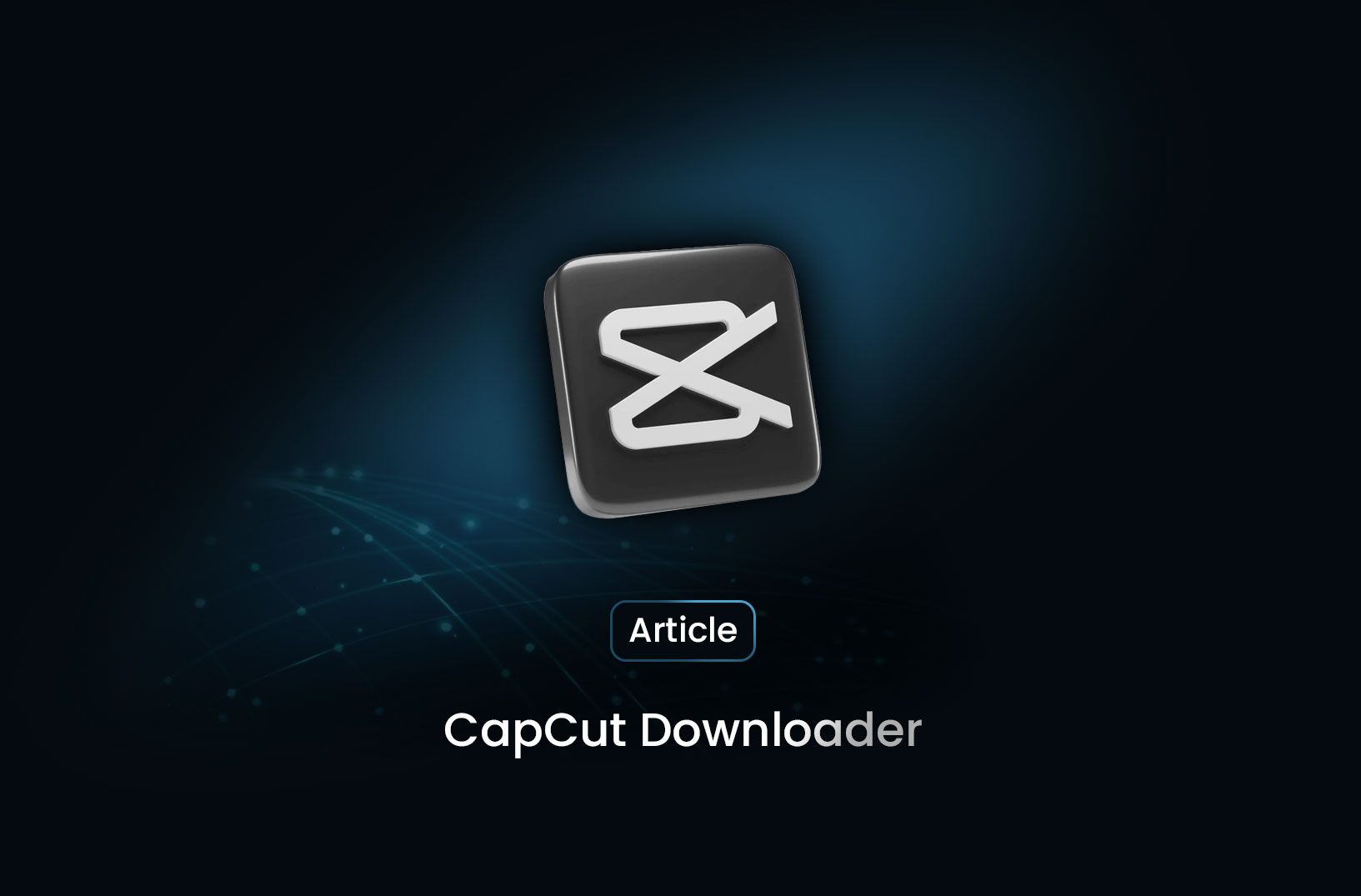
CapCut Downloader: Your Complete Guide
Learn how to download CapCut videos without watermark using built-in tools, web downloaders, or automated APIs. Discover how MrScraper offers a scalable CapCut downloader solution for professionals and businesses.
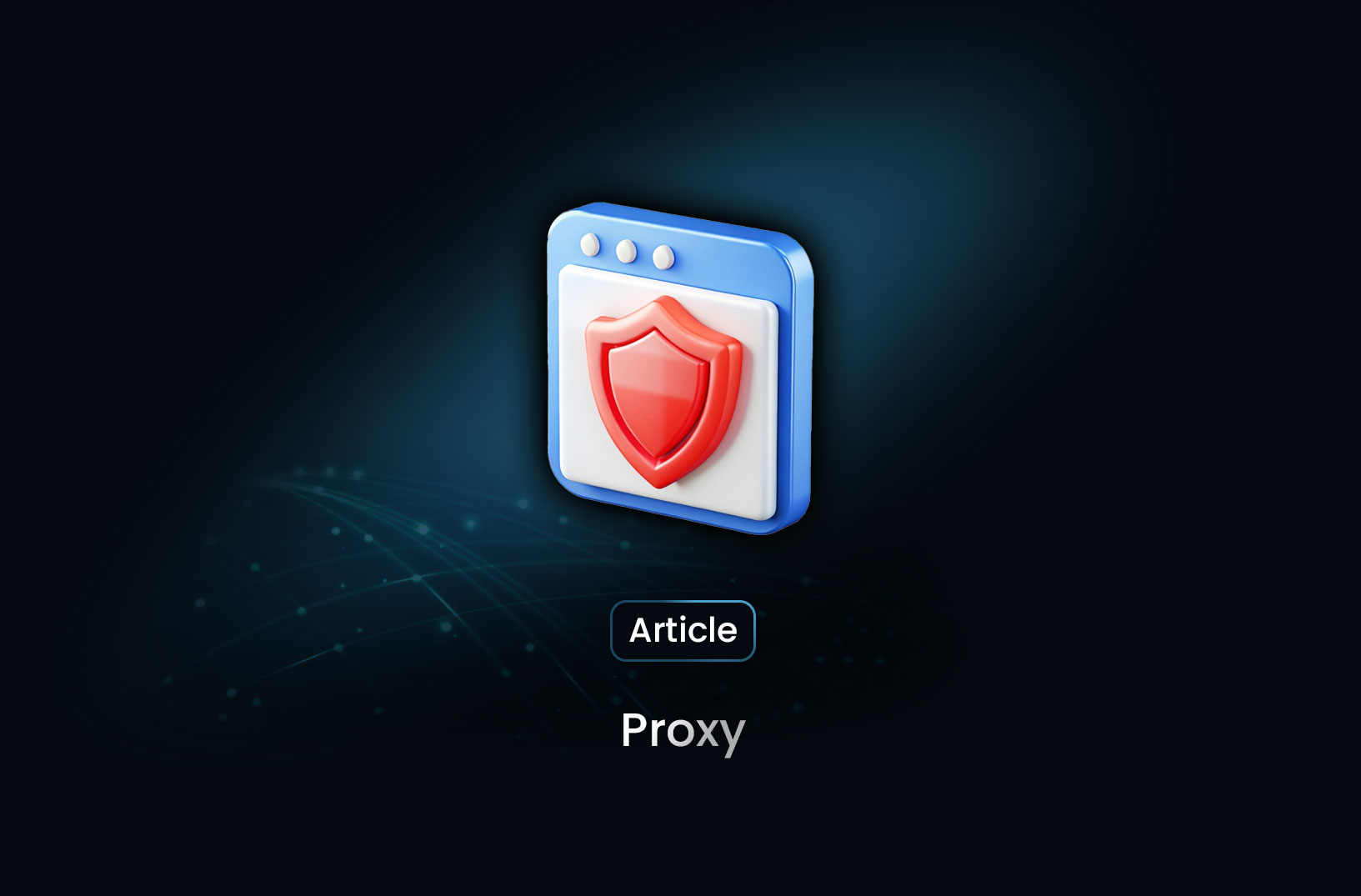
Proxy Meaning: What Are Proxies and Why Do They Matter?
Understand how proxies work, why they’re used, and how they support secure, scalable web scraping.
@MrScraper_
@MrScraper