Parsing XML with Python: A Comprehensive Guide
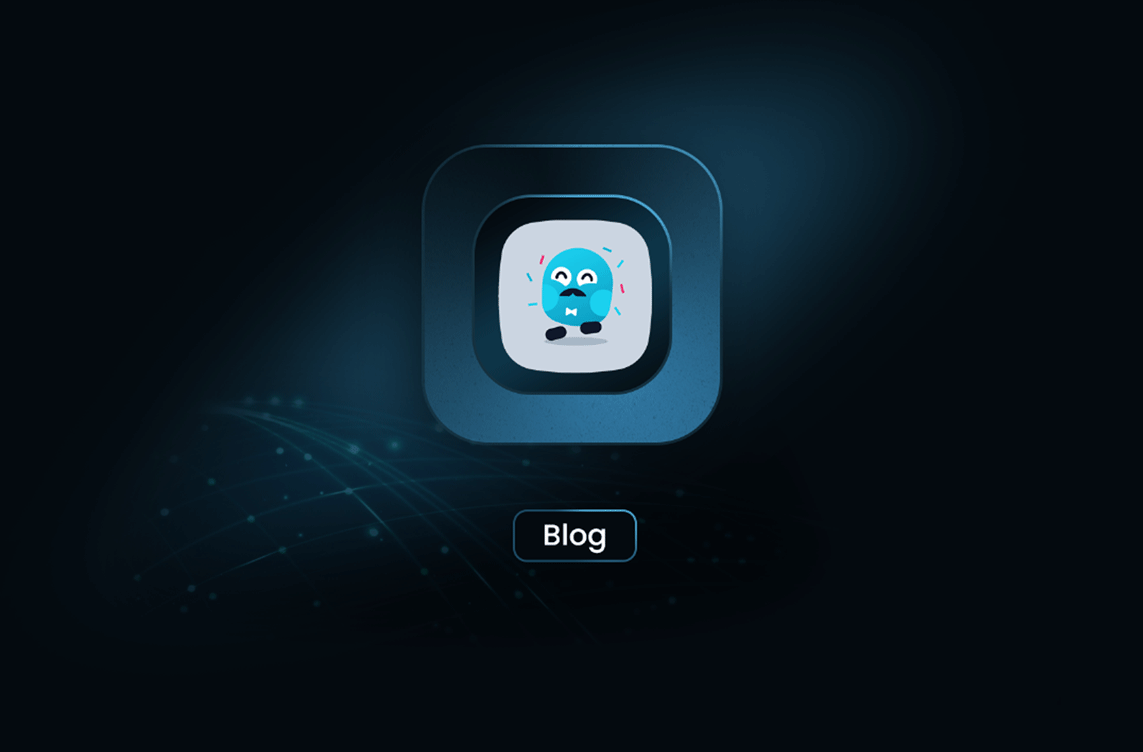
XML, or Extensible Markup Language, is a standardized format for structuring data. When it comes to web scraping, the ability to parse XML documents efficiently is crucial. Python, with its rich ecosystem of libraries, provides robust tools for this task. In this tutorial, we'll delve into the world of XML parsing using Python and explore the powerful
lxml
library.
Why Choose lxml for XML Parsing?
- Speed: lxml is known for its exceptional performance, making it an excellent choice for large XML documents.
- Flexibility: It supports various XML standards and offers advanced features like XPath and XSLT.
- Pythonic API: lxml provides a Pythonic API, making it easy to learn and use.
A Step-by-Step Tutorial
Let's consider a simple XML file containing book information:
XML
<bookstore>
<book category="COOKING">
<title lang="en">Everyday Italian</title>
<author>Giada De Laurentiis</author>
<year>2005</year>
<price>30.00</price>
</book>
</bookstore>
To parse this XML file using lxml, follow these steps:
1. Install lxml:
pip install lxml`
2. Import the library:
import lxml.etree as ET`
3. Parse the XML:
Python
tree = ET.parse('books.xml')
root = tree.getroot()
4. Iterate over elements:
Python
for book in root.iter('book'):
title = book.find('title').text
author = book.find('author').text
print(f"Title: {title}, Author: {author}")
Using XPath for More Complex Queries
XPath is a powerful language for selecting nodes in an XML document. Here's an example of using XPath to find all books with the category "MARKETING":
Python
for book in root.xpath('//book[@category="MARKETING"]'):
# ...
Tips and Tricks
- Large XML Files: For large XML files, consider using iterparse to process the document incrementally.
- Error Handling: Implement robust error handling to handle unexpected data or parsing errors.
- Performance Optimization: Explore techniques like caching and profiling to optimize your parsing code.
Conclusion
Parsing XML with Python is a straightforward task when using the right tools. lxml provides a powerful and efficient way to work with XML data. By understanding the basics of XML and leveraging the features of lxml, you can effectively extract information from XML documents for various applications.
Table of Contents
Take a Taste of Easy Scraping!
Get started now!
Step up your web scraping
Find more insights here
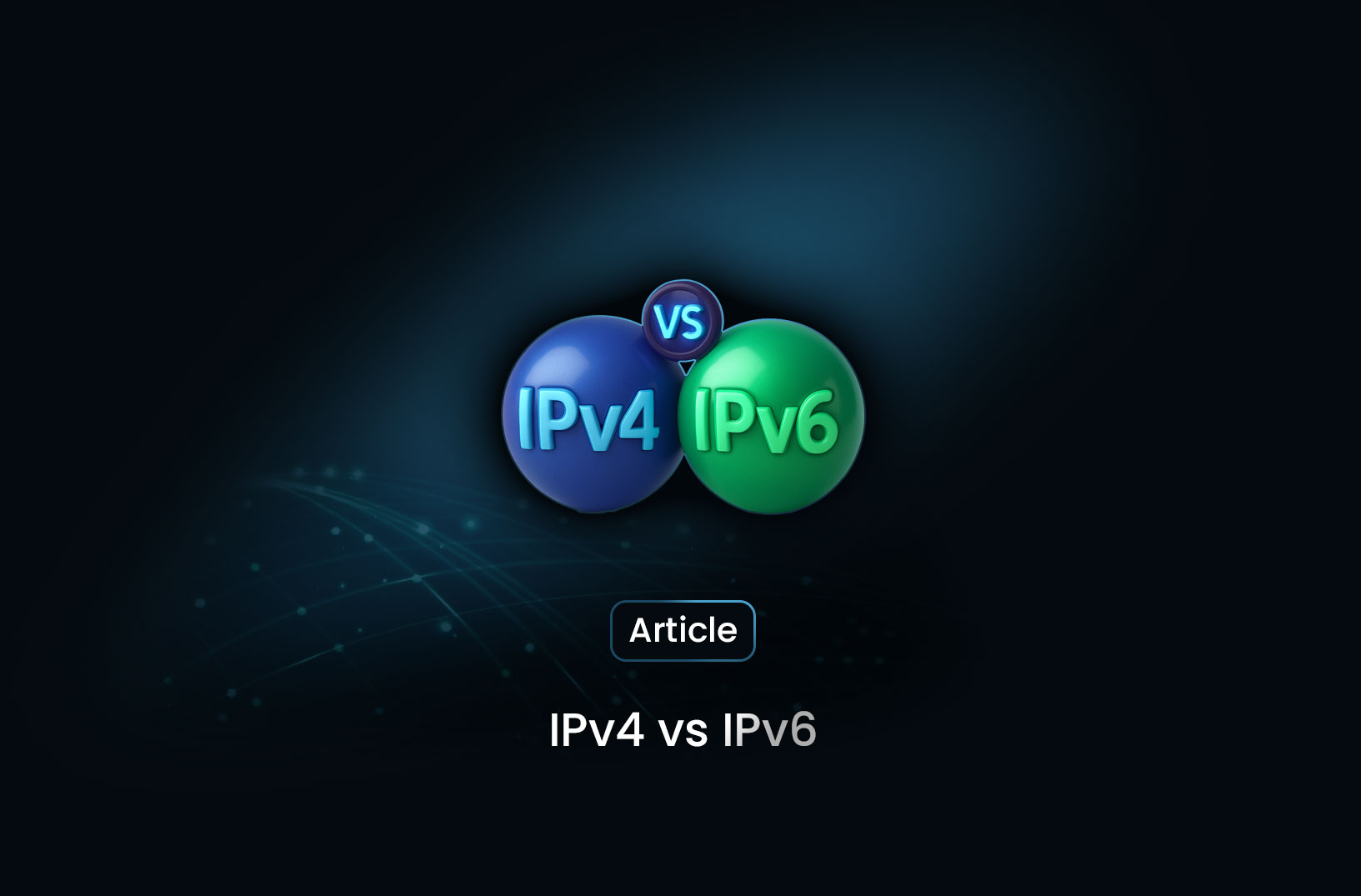
IPv4 vs IPv6 for Beginners: Simple Comparison
IPv4 (Internet Protocol version 4) is the most widely used internet protocol today. It uses a 32-bit numeric address system, which looks like 192.168.1.1.
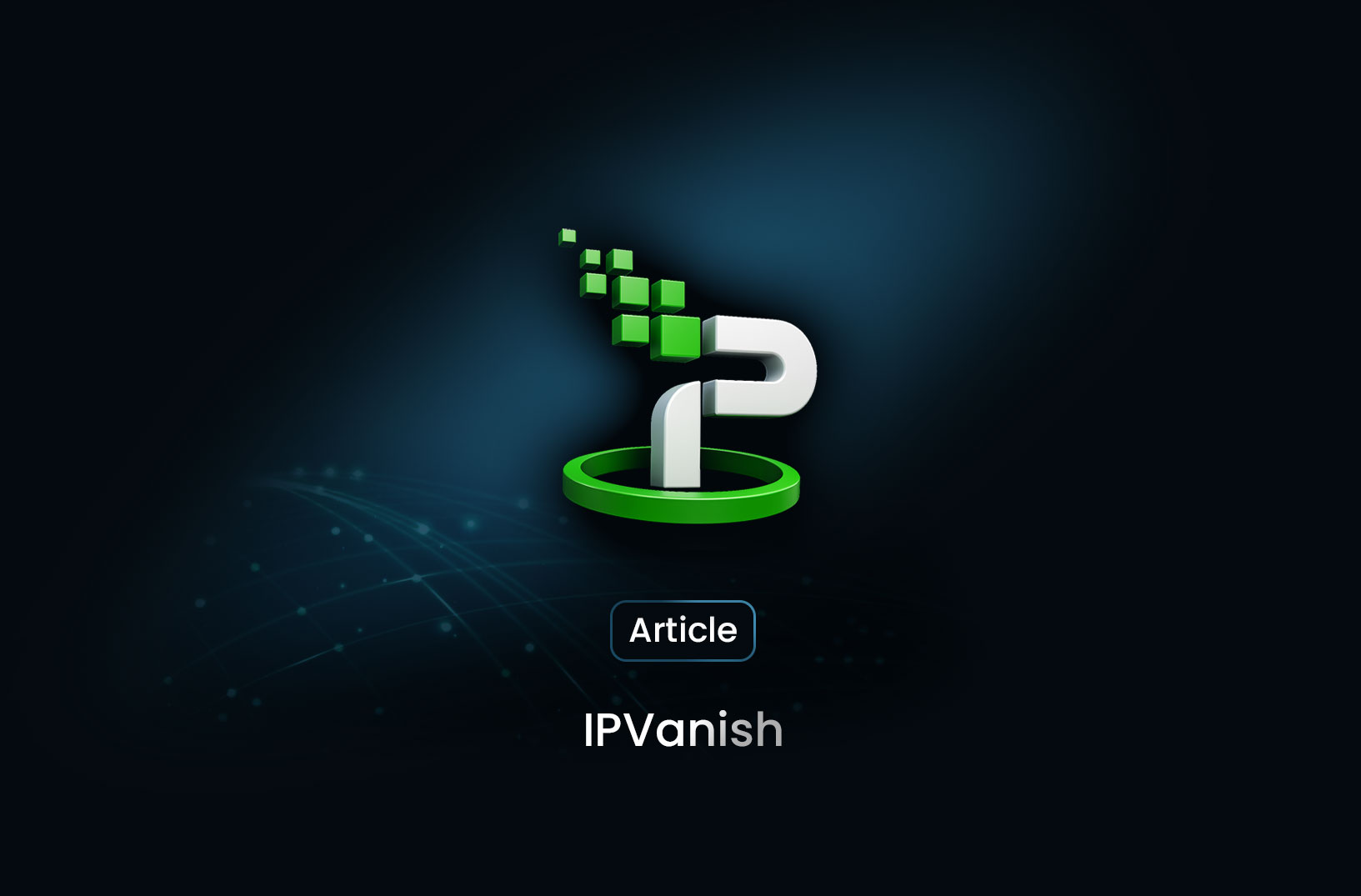
How to Log In to IPVanish: Step-by-Step Tutorial (2025)
IPVanish is a popular VPN provider founded in 2012. It offers apps for Windows, macOS, Android, iOS, Fire TV, and even manual setup for routers and NAS devices.
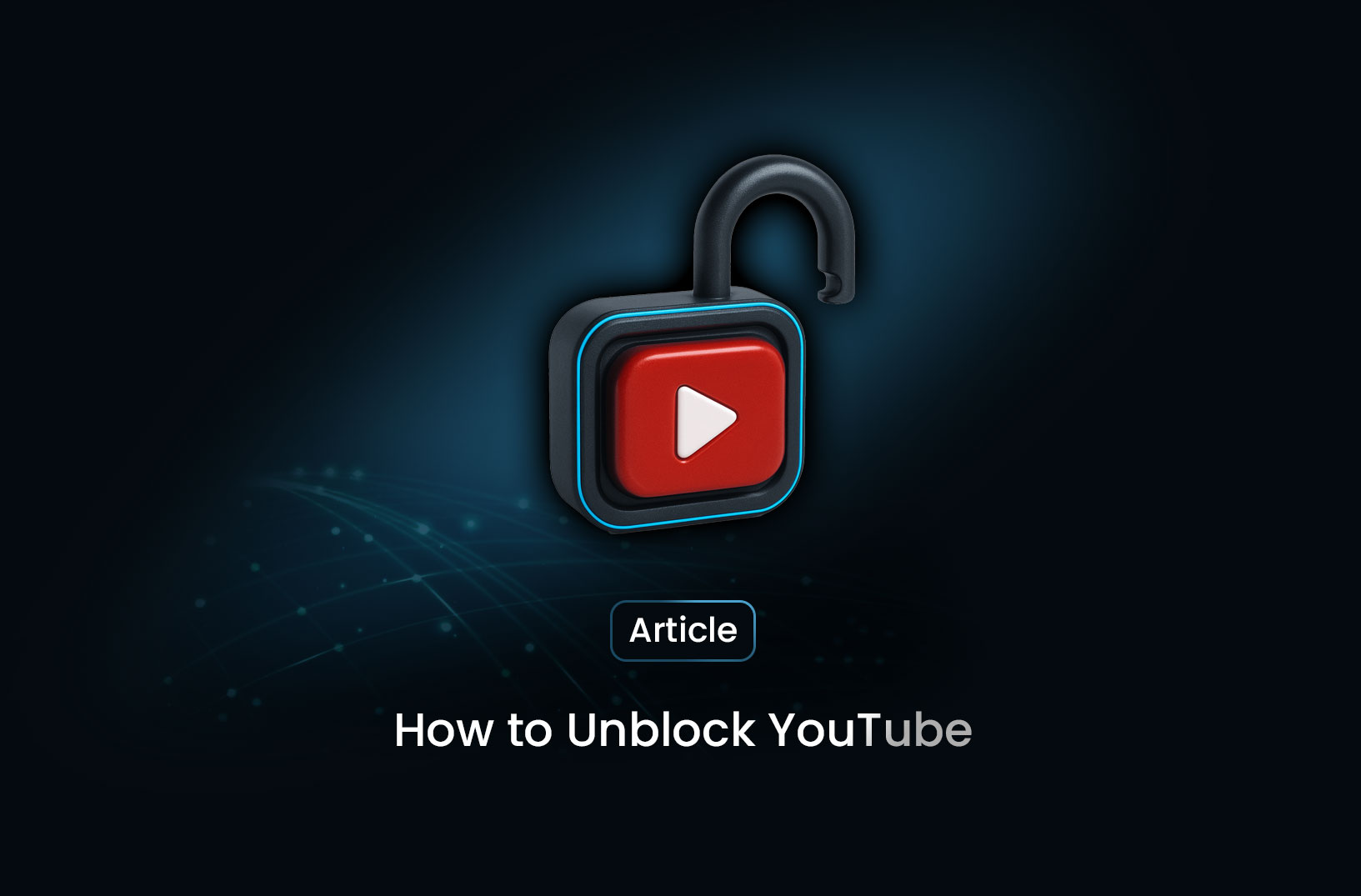
How to Unblock YouTube: Practical Methods and Tools
Learn how to unblock YouTube using VPNs, proxies, DNS changes, or MrScraper’s tool to access YouTube search data even on restricted networks.
@MrScraper_
@MrScraper