Mastering the Curl Converter: Transforming cURL Commands into Code Snippets for MrScraper API
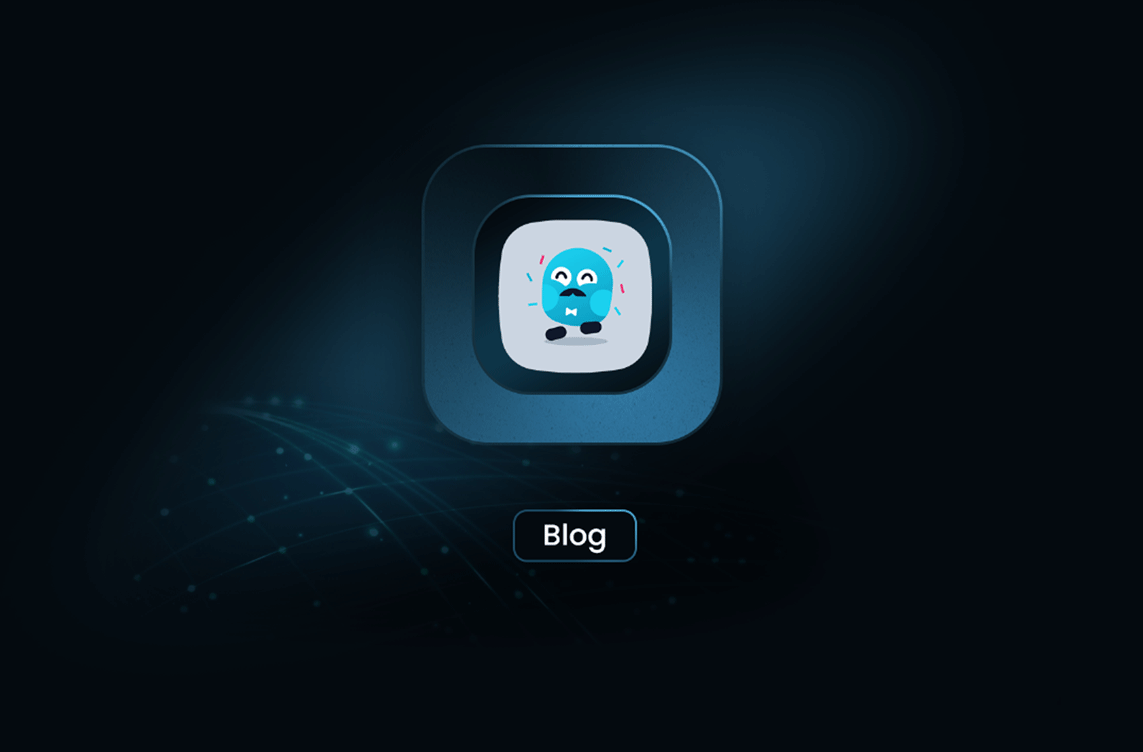
In the realm of web development and API integration, cURL (Client URL) commands play a pivotal role in making HTTP requests to interact with servers and APIs. However, transforming these commands into code snippets compatible with various programming languages can be a tedious process. Enter the curl converter—a handy tool designed to automate this transformation, making your development process smoother and more efficient. In this blog post, we’ll explore what a curl converter is, how to use it effectively for MrScraper API, and provide practical examples to illustrate its benefits.
What is cURL?
cURL is a command-line tool used to send and receive data to and from servers using various protocols, including HTTP, HTTPS, FTP, and more. It's widely used by developers to test APIs, download files, and even automate tasks. A typical cURL command looks like this:
curl -X GET "https://api.example.com/data" -H "Authorization: Bearer YOUR_API_KEY"
While cURL commands are powerful, translating them into code that can be integrated into your application can be challenging. This is where a curl converter comes into play.
What is a Curl Converter?
A curl converter is an online or local tool that helps developers convert cURL commands into code snippets in various programming languages, such as Python, JavaScript, PHP, Ruby, and others. This conversion streamlines the development process by eliminating the need to manually write the HTTP request code, reducing the likelihood of errors.
How to Use a Curl Converter
Using a curl converter is straightforward. Here’s a step-by-step guide to converting a cURL command into your desired programming language:
- Write Your cURL Command: Start by constructing the cURL command you want to convert. Example cURL command for the MrScraper API:
curl --request POST \
--url https://app.mrscraper.com/api/scrapers/leads-generator/twitter/create-and-run \
--header 'Authorization: Bearer <ACCESS_TOKEN>' \
--header 'Content-Type: application/json' \
--data '{
"name": "Twitter - MrScraper",
"keywords": "MrScraper",
"sentiment_type": "positive",
"expected_data": 100
}'
- Access a Curl Converter: Visit a curl converter website, such as curl.trillworks.com or any similar tool.
- Paste Your Command: Paste your cURL command into the input field provided by the curl converter.
- Select Your Language: Choose the programming language you want the cURL command to be converted into.
- Copy the Output: The curl converter will generate the corresponding code snippet. Copy this output to integrate into your project.
Example Conversion
Let’s illustrate how a curl converter works by converting a cURL command for the MrScraper API into a few programming languages.
Original cURL Command
curl --request POST \
--url https://app.mrscraper.com/api/scrapers/leads-generator/twitter/create-and-run \
--header 'Authorization: Bearer <ACCESS_TOKEN>' \
--header 'Content-Type: application/json' \
--data '{
"name": "Twitter - MrScraper",
"keywords": "MrScraper",
"sentiment_type": "positive",
"expected_data": 100
}'
Expected Result
Upon sending this request, you would receive a response like:
{
"message": "Scraping queued successfully",
"scraper": {
"id": 101,
"name": "Twitter - MrScraper",
"url": [
"Default"
],
"urls": [
"Default"
],
"scheduled": false,
"schedule": null,
"created_at": "2024-09-20T06:45:47.000000Z",
"updated_at": "2024-09-20T06:45:47.000000Z"
},
"results": [
{
"scraping_run_id": 1115,
"user_id": 26,
"scraper_name": "Twitter - MrScraper",
"scrapped_url": "Default",
"scraper_id": 101,
"status": "running",
"created_at": "2024-09-20T06:45:47.000000Z",
"updated_at": "2024-09-20T06:45:47.000000Z",
"id": 1917
}
]
}
Converted to Python (using requests
library)
import requests
url = "https://app.mrscraper.com/api/scrapers/leads-generator/twitter/create-and-run"
headers = {
"Authorization": "Bearer <ACCESS_TOKEN>",
"Content-Type": "application/json"
}
data = {
"name": "Twitter - MrScraper",
"keywords": "MrScraper",
"sentiment_type": "positive",
"expected_data": 100
}
response = requests.post(url, headers=headers, json=data)
print(response.json())
Converted to JavaScript (using fetch
)
const url = "https://app.mrscraper.com/api/scrapers/leads-generator/twitter/create-and-run";
const headers = {
"Authorization": "Bearer <ACCESS_TOKEN>",
"Content-Type": "application/json"
};
const data = {
"name": "Twitter - MrScraper",
"keywords": "MrScraper",
"sentiment_type": "positive",
"expected_data": 100
};
fetch(url, {
method: "POST",
headers: headers,
body: JSON.stringify(data)
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
Converted to PHP (using cURL
functions)
$url = "https://app.mrscraper.com/api/scrapers/leads-generator/twitter/create-and-run";
$headers = [
"Authorization: Bearer <ACCESS_TOKEN>",
"Content-Type: application/json"
];
$data = json_encode([
"name" => "Twitter - MrScraper",
"keywords" => "MrScraper",
"sentiment_type" => "positive",
"expected_data" => 100
]);
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, $data);
$response = curl_exec($ch);
curl_close($ch);
echo $response;
Benefits of Using a Curl Converter
- Time-Saving: Quickly convert cURL commands into code snippets without manually writing the request code, speeding up your development process.
- Error Reduction: Minimize the chances of errors in syntax when translating cURL commands to code manually.
- Multiple Language Support: A good curl converter supports various programming languages, making it versatile for developers working in different environments.
- Learning Tool: For beginners, a curl converter can serve as an educational resource to understand how cURL commands translate to different programming languages.
Incorporating APIs into your applications can significantly enhance functionality, and cURL is a powerful tool for testing and interacting with these APIs. A curl converter simplifies the process of transforming cURL commands into code snippets, saving time and reducing errors. If you want to explore more APIs, feel free to visit docs.mrscraper.com for comprehensive documentation.
Whether you’re a seasoned developer or a beginner, leveraging a curl converter can streamline your workflow and make your coding experience more efficient. If you haven't tried using a curl converter yet, give it a go! It may just become an indispensable tool in your development toolkit. Happy coding!
Table of Contents
Take a Taste of Easy Scraping!
Get started now!
Step up your web scraping
Find more insights here
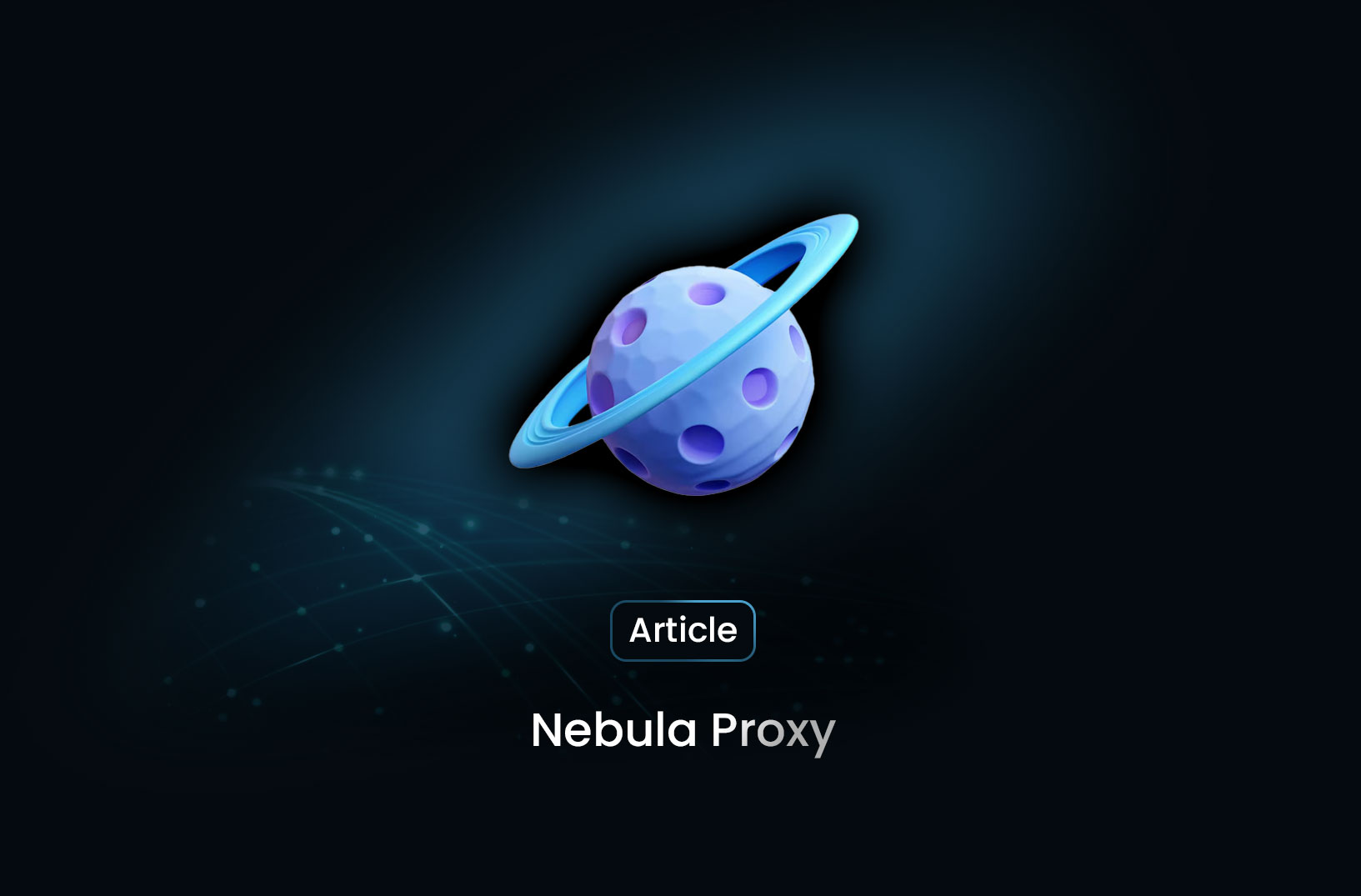
What is Nebula Proxy? A Powerful Tool for Web Scraping, SEO, and Online Privacy
Discover how Nebula Proxy enhances web scraping, SEO monitoring, and online privacy. Learn about its features, use cases, and setup, plus top alternatives for seamless data extraction and secure browsing.
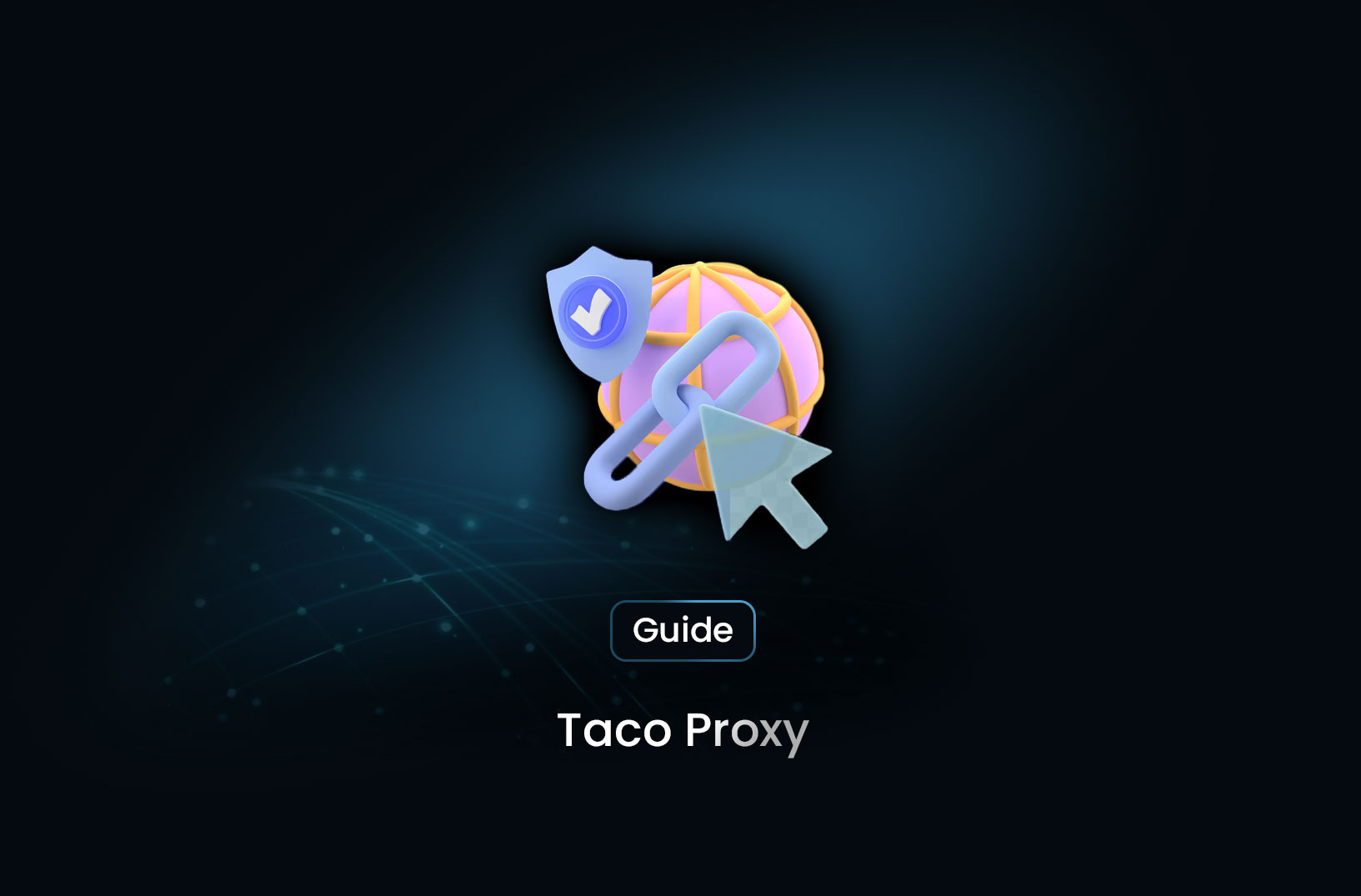
Taco Proxy: Understanding Its Role and Use Cases
Learn what Taco Proxy is, how it works, and its key use cases for web scraping, SEO monitoring, cybersecurity, and bypassing geo-restrictions. Get step-by-step proxy configuration guides for Python, Scrapy, and cURL.
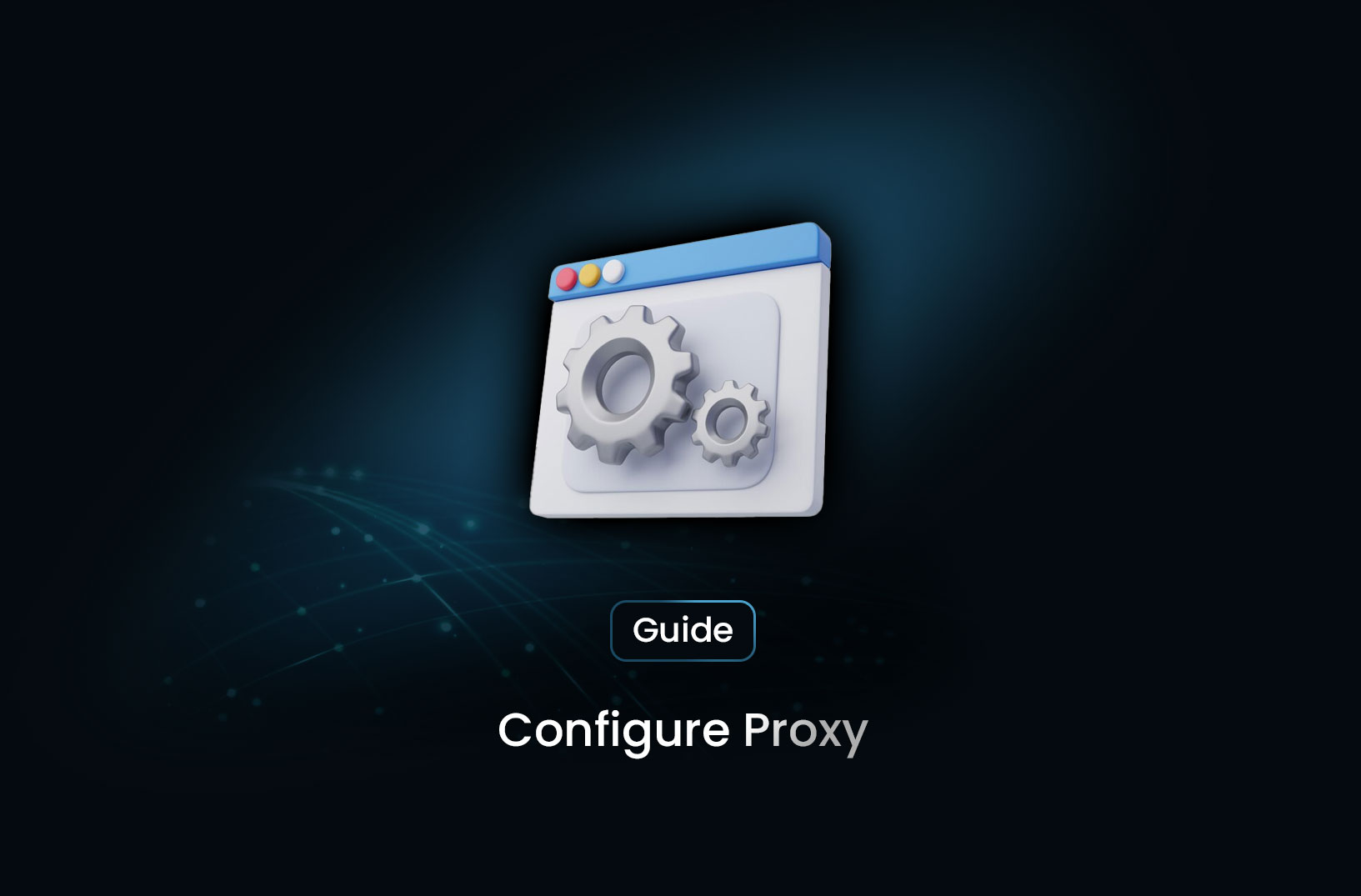
How to Configure Proxy
Learn how to set up a proxy on Windows, macOS, Linux, browsers, and command-line tools like cURL and Python.
@MrScraper_
@MrScraper