Mastering reduce() in Python
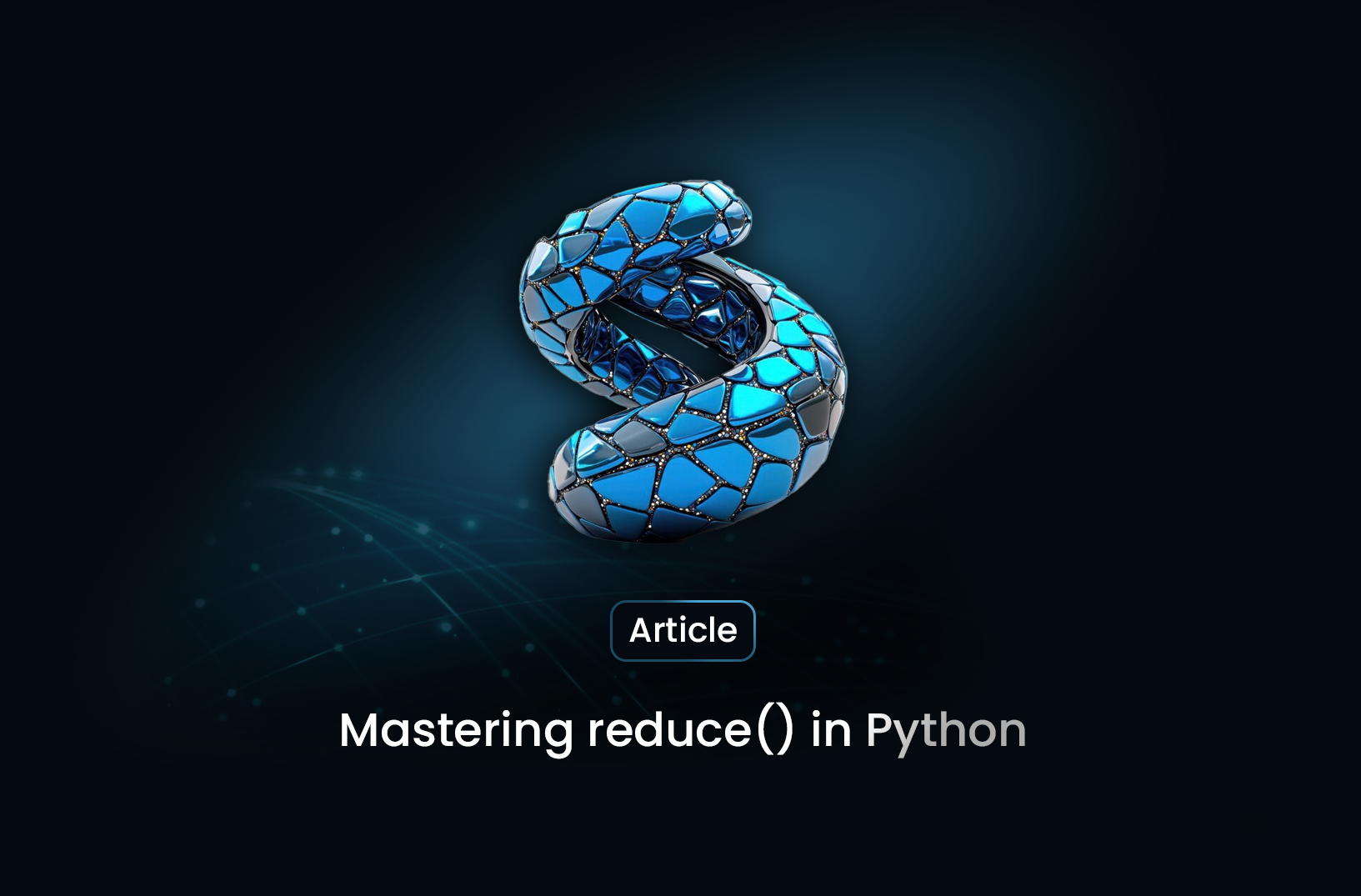
What is reduce in Python?
The reduce function in Python, part of the functools module, applies a two-argument function cumulatively to the elements of an iterable. It reduces the iterable to a single cumulative value, making it a powerful tool for aggregation tasks.!
Syntax
from functools import reduce
result = reduce(function, iterable[, initializer])
- function: A two-argument function to apply to the elements.
- iterable: The data structure to be reduced.
- initializer (optional): A value that is placed before the elements of the iterable in the calculation.
How reduce Works
reduce takes the first two elements of the iterable, applies the function, and then uses the result with the next element, continuing until all elements are processed.
Example Usage:
1. Summing a List
from functools import reduce
numbers = [1, 2, 3, 4]
sum_result = reduce(lambda x, y: x + y, numbers)
print(sum_result) # Output: 10
2. Finding the Product of a List
from functools import reduce
numbers = [1, 2, 3, 4]
product_result = reduce(lambda x, y: x * y, numbers)
print(product_result) # Output: 24
3. Using an Initializer
from functools import reduce
numbers = [1, 2, 3, 4]
sum_with_init = reduce(lambda x, y: x + y, numbers, 10)
print(sum_with_init) # Output: 20
Common Use Cases for reduce
Use Case | Description |
---|---|
Summation | Aggregate all numbers into a total sum. |
Product Calculation | Multiply elements to find the product. |
Custom String Concatenation | Combine a list of strings with custom delimiters. |
Max or Min in Iterables | Custom implementations of finding maximum or minimum with conditions. |
Pros and Cons of reduce
Pros | Cons |
---|---|
Simplifies iterative reduction | Less readable than explicit loops |
Combines logic and operation | May be overkill for simpler use cases |
Works with any iterable | Requires understanding of lambda functions |
Alternatives to reduce
While reduce
is powerful, Python offers alternative, often more readable approaches:
- Loops: Explicit and easy to understand.
-
Built-in Functions: E.g.,
sum()
ormath.prod()
for basic aggregation tasks.
Best Practices for Using reduce
- Readability First: Use reduce when it makes the code clearer and avoid overcomplicating simple tasks.
- Combine with Descriptive Functions: Replace lambdas with named functions for better readability.
Example:
from functools import reduce
def add(x, y):
return x + y
numbers = [1, 2, 3, 4]
result = reduce(add, numbers)
print(result) # Output: 10
Conclusion
The reduce
function is a powerful tool for applying cumulative operations in Python. While it can compactly perform complex operations, always weigh its use against clarity and simplicity in your code. For tasks like summing or multiplying, built-in alternatives like sum()
or math.prod()
might be more appropriate. Use reduce
for advanced use cases requiring custom operations.
Table of Contents
Take a Taste of Easy Scraping!
Get started now!
Step up your web scraping
Find more insights here
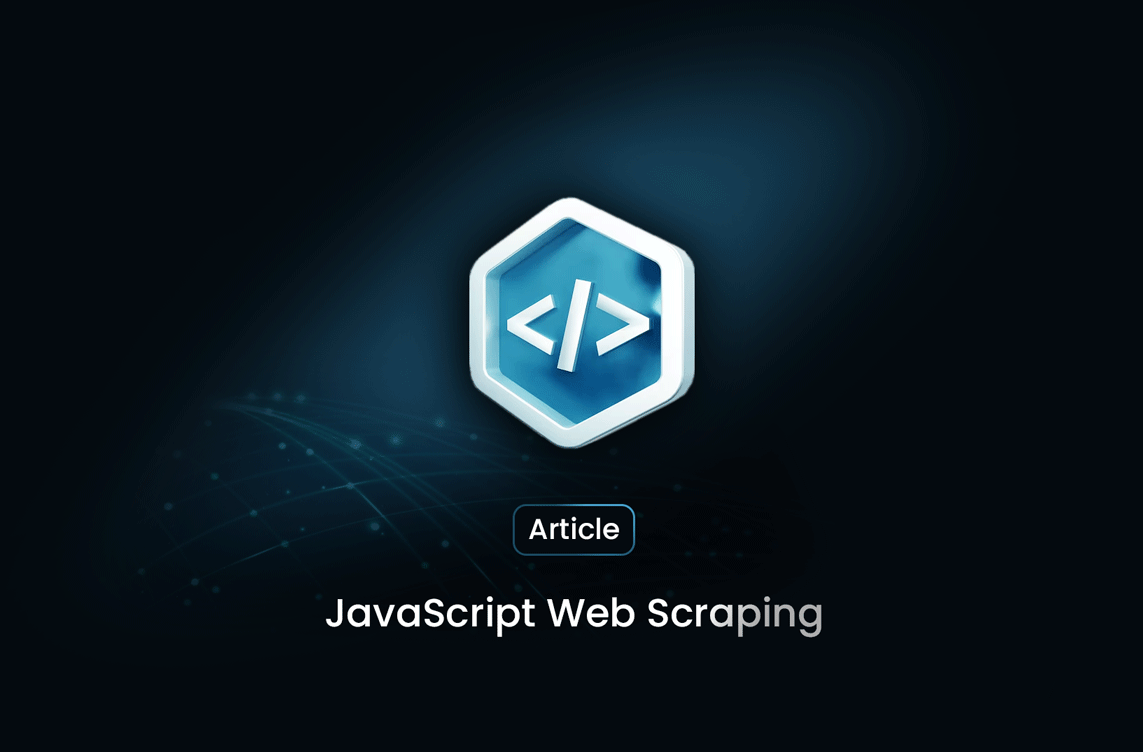
JavaScript Web Scraping
JavaScript is a great choice for web scraping with tools like Puppeteer and Cheerio for both static and dynamic sites. For more complex tasks, like bypassing CAPTCHAs or handling large-scale data, using AI-powered tools like Mrscraper can make the process easier, so you can focus on the data instead of the technical details.
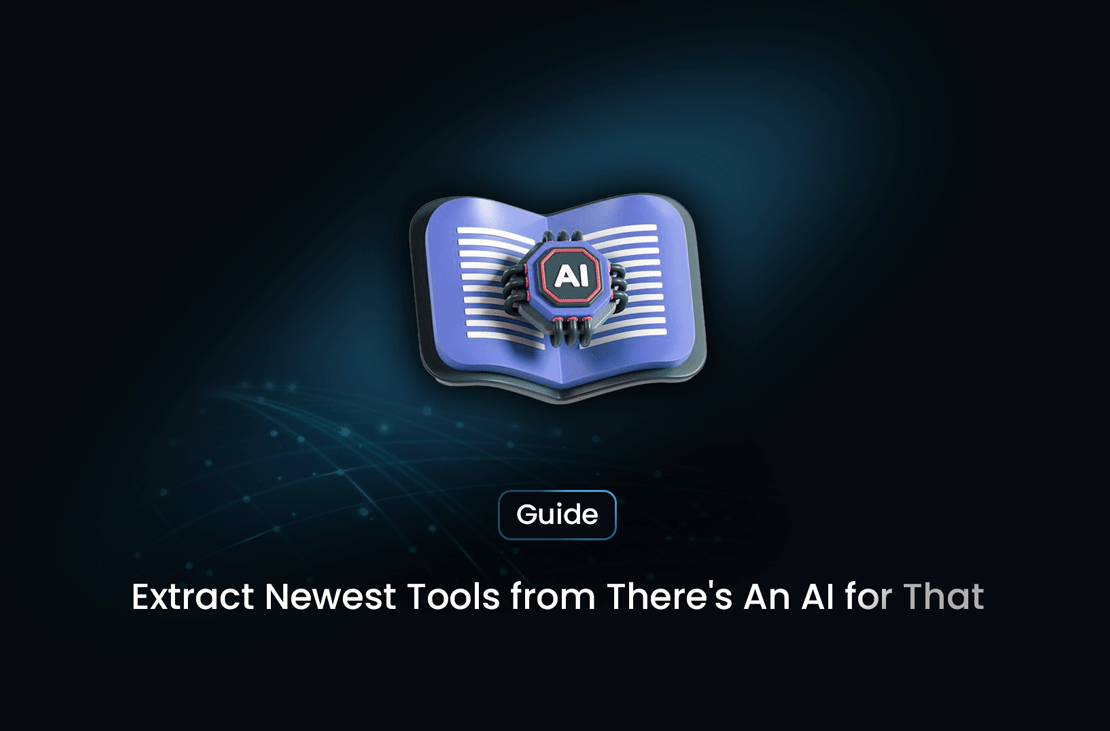
There's an AI for That: Exploring Tools and Extracting Value from AI Directories
"There's An AI For That" is a curated directory of AI tools covering countless categories—from AI chatbots and art generators to complex data analysis tools. It’s essentially a one-stop solution for professionals, developers, and AI enthusiasts looking to find the perfect tool for their needs.
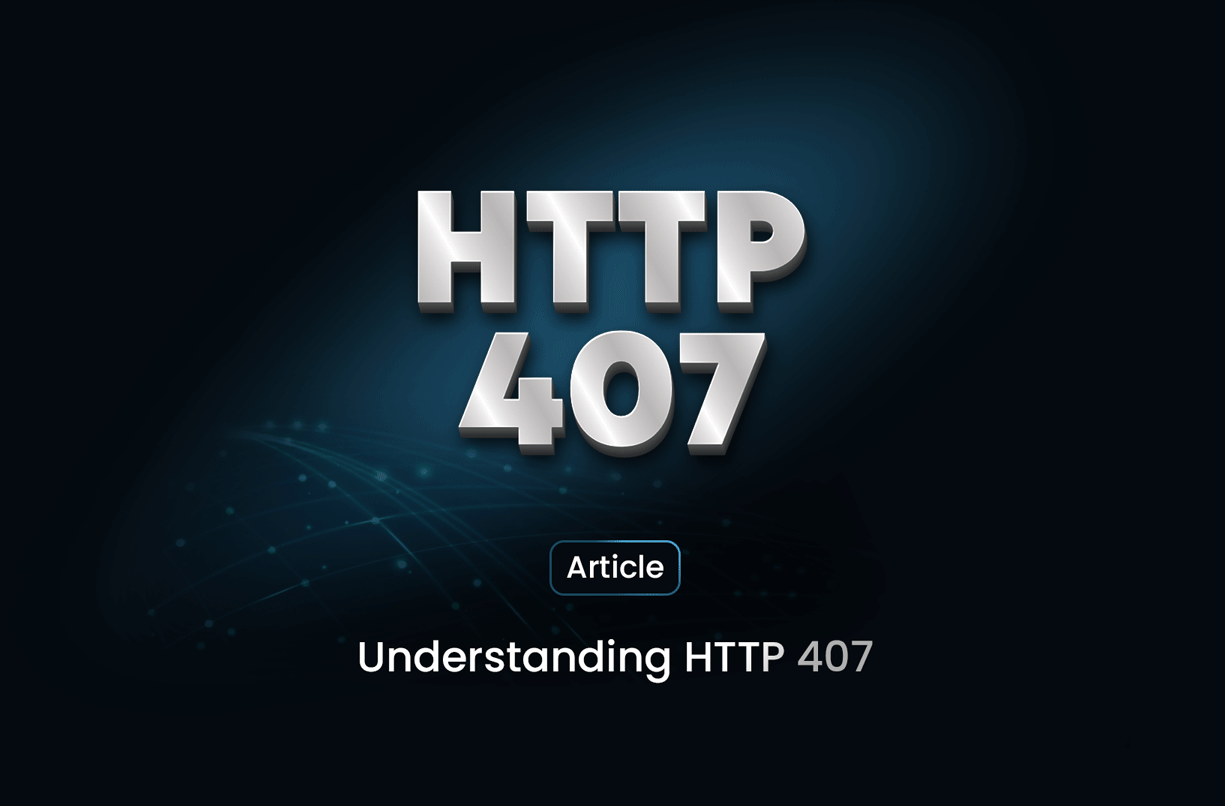
Understanding HTTP 407: Proxy Authentication Required
The HTTP 407 Proxy Authentication Required status code means a proxy server blocked the request due to missing authentication, similar to 401 but specific to proxies.
@MrScraper_
@MrScraper