Mastering json.dumps in Python: Real-World Applications and How-To Guide
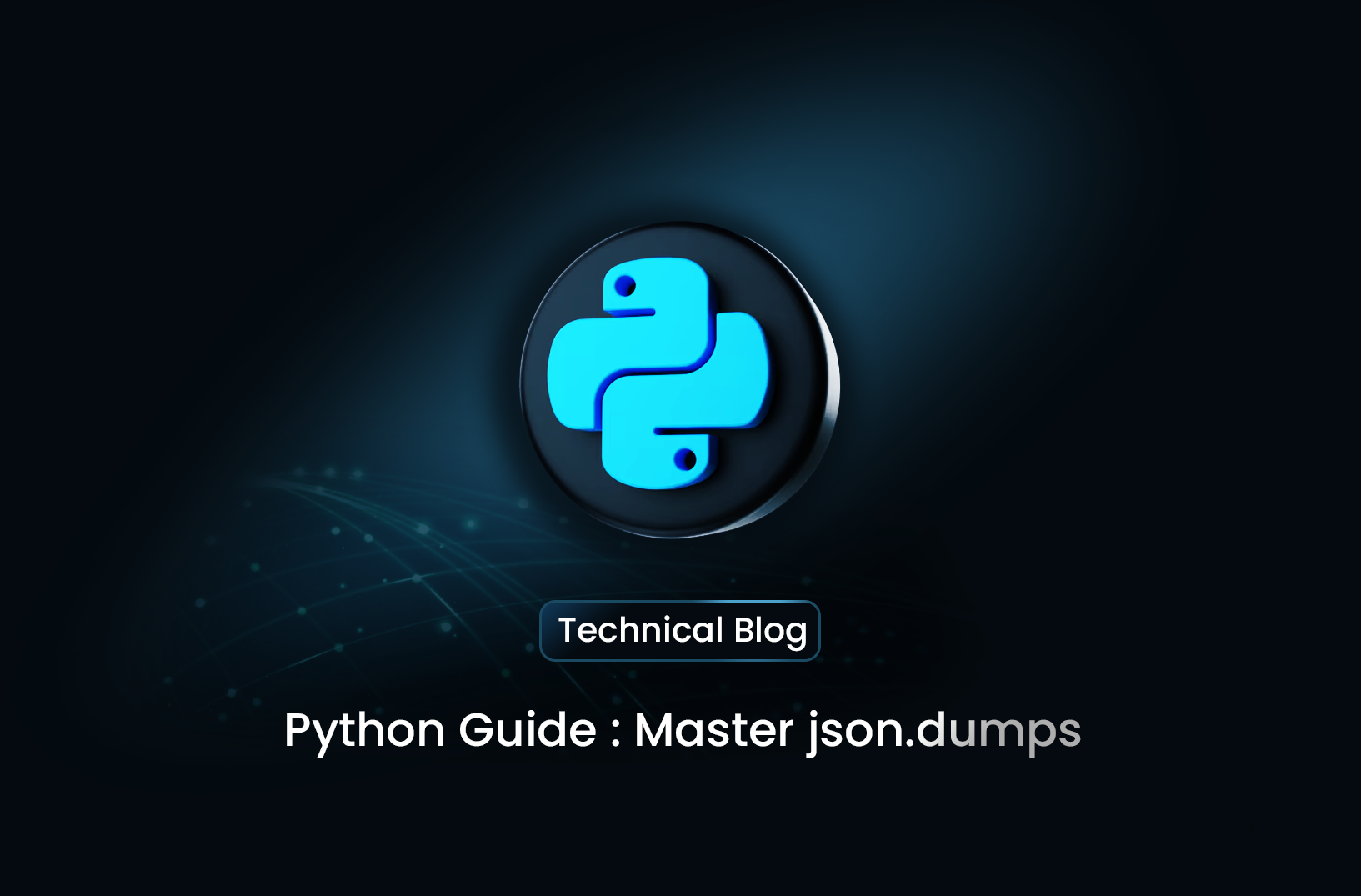
Converting Python objects to JSON strings with json.dumps
is critical in many programming scenarios, such as web APIs, data serialization, and log management. This guide explores not only the technical syntax but also real-world use cases and step-by-step examples to help you make the most of json.dumps
in your projects.
What is json.dumps?
json.dumps() converts Python objects to JSON strings, providing an easy way to serialize data. While JSON is universal for data exchange in web APIs, json.dumps ensures data is encoded in a compact, structured format that applications across languages can process.
Key Use Cases for json.dumps
1. API Response Formatting
In web applications, data is often sent in JSON format to ensure compatibility with various frontend clients. Converting complex data structures like dictionaries or lists to JSON makes it accessible across platforms.
2. Configuration Management
Storing configurations in JSON allows for easy sharing and reuse. Configurations can be stored in JSON format, serialized with json.dumps when saving, and deserialized with json.loads when needed.
3. Logging and Debugging Complex Data
When tracking application events, logging structured JSON can provide insights. JSON-formatted logs are searchable and readable, and tools like Kibana or Splunk can analyze them more effectively.
Step-by-Step Case Studies
Case Study 1: Converting an API Response to JSON
Scenario Imagine an API endpoint that fetches user data from a database and returns it to a frontend application. To make this data readable and accessible, you use json.dumps.
Step-by-Step Guide
- Retrieve Data from Database: Fetch data from the database, stored in a Python dictionary.
- Convert to JSON with json.dumps: Pass the data dictionary to json.dumps.
- Return JSON to Client: Send the JSON-formatted string as an API response.
import json
def fetch_user_data():
# Simulated database response
user_data = {
"id": 1,
"name": "Alice",
"email": "alice@example.com",
"roles": ["admin", "editor"]
}
# Convert to JSON
return json.dumps(user_data)
# Call the function and print the JSON response
api_response = fetch_user_data()
print(api_response)
Output:
{"id": 1, "name": "Alice", "email": "alice@example.com", "roles": ["admin", "editor"]}
Why This Works Sending JSON data allows the client (such as a JavaScript frontend) to parse the response and display user information easily.
Case Study 2: Storing Application Configurations in JSON
Scenario Storing configuration files in JSON allows for easy modifications and integration. This example demonstrates how to convert a configuration dictionary to JSON for storage.
Step-by-Step Guide
- Create Configuration Dictionary: Define configuration settings.
- Convert to JSON for Storage: Use json.dumps to create a JSON string.
- Save to File: Store the JSON string in a file.
import json
# Step 1: Define configuration settings
config = {
"database": {
"host": "localhost",
"port": 5432,
"username": "admin"
},
"logging": {
"level": "INFO",
"format": "%(asctime)s - %(levelname)s - %(message)s"
}
}
# Step 2: Convert to JSON
config_json = json.dumps(config, indent=4)
# Step 3: Write to a file
with open("config.json", "w") as file:
file.write(config_json)
Benefits: Storing configurations as JSON simplifies sharing settings between different environments and enables easy reading by automated scripts.
Case Study 3: Enhanced Logging with JSON Data
Scenario In monitoring or debugging, logging JSON-formatted data allows analysis tools to parse complex information easily. Here’s how to log-structured data with json.dumps.
Step-by-Step Guide
- Define Event Data: Create a dictionary representing the log data.
- Serialize with json.dumps: Convert to JSON for easier readability.
- Log to File: Write the JSON string to a log file.
import json
from datetime import datetime
def log_event(event_type, details):
# Step 1: Define log data
log_data = {
"timestamp": datetime.now().isoformat(),
"event": event_type,
"details": details
}
# Step 2: Serialize to JSON
log_entry = json.dumps(log_data)
# Step 3: Log to file
with open("app.log", "a") as log_file:
log_file.write(log_entry + "\n")
# Example usage
log_event("USER_LOGIN", {"username": "Alice", "status": "success"})
Log Output:
{"timestamp": "2024-10-29T12:45:00", "event": "USER_LOGIN", "details": {"username": "Alice", "status": "success"}}
Why This is Useful Structured JSON logs enable querying based on log fields, making analysis straightforward.
Conclusion
With json.dumps, converting Python objects to JSON is a powerful way to handle data for API responses, configurations, and logging. By understanding use cases and options, you can ensure JSON is used efficiently and effectively across applications.
Table of Contents
Take a Taste of Easy Scraping!
Get started now!
Step up your web scraping
Find more insights here
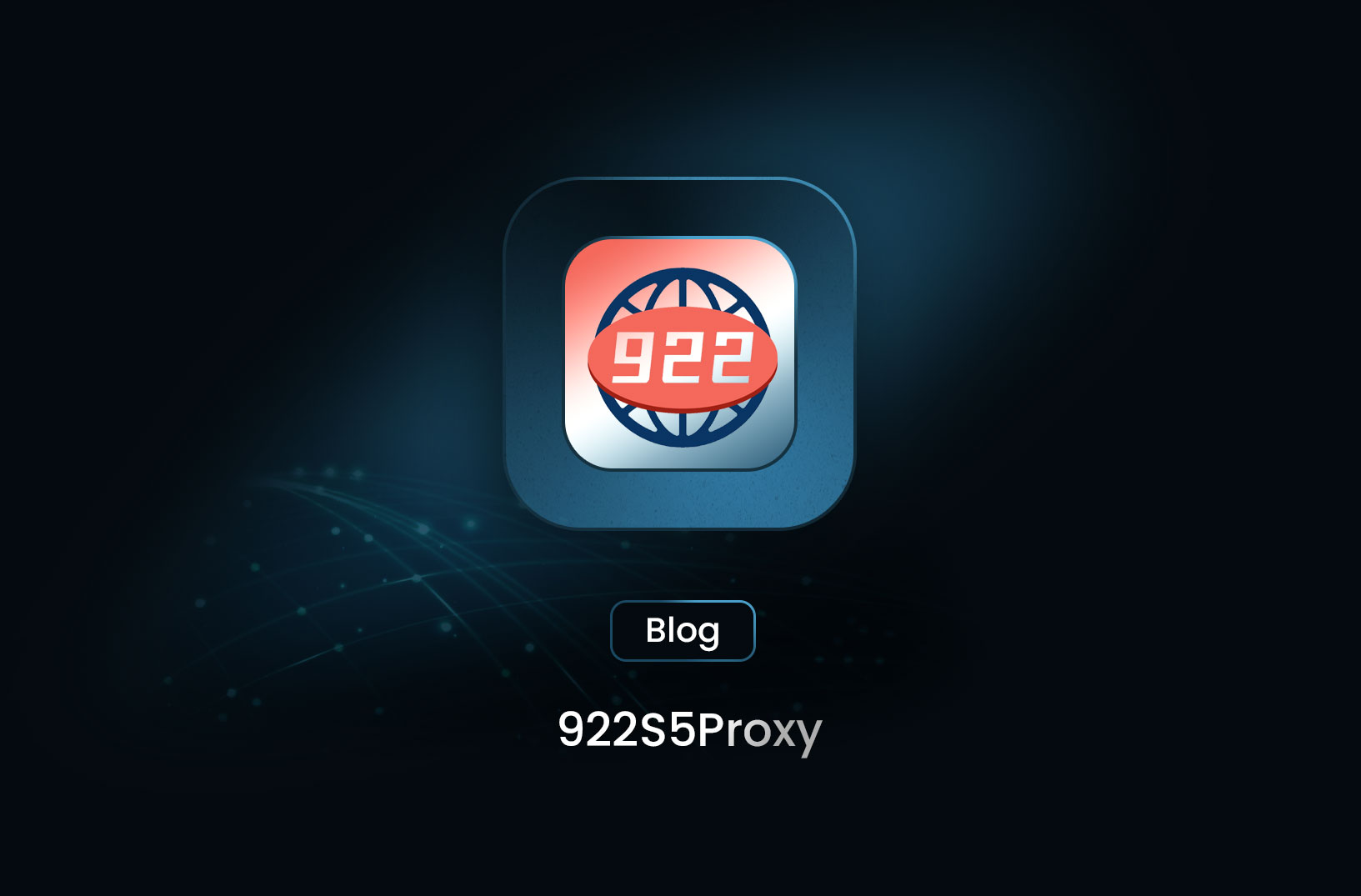
Enhancing Web Scraping Performance with 922S5Proxy
Boost your web scraping success with 922S5Proxy. Learn how its high-speed, anonymous SOCKS5 residential proxies help bypass restrictions, avoid bans, and optimize data extraction efficiency.
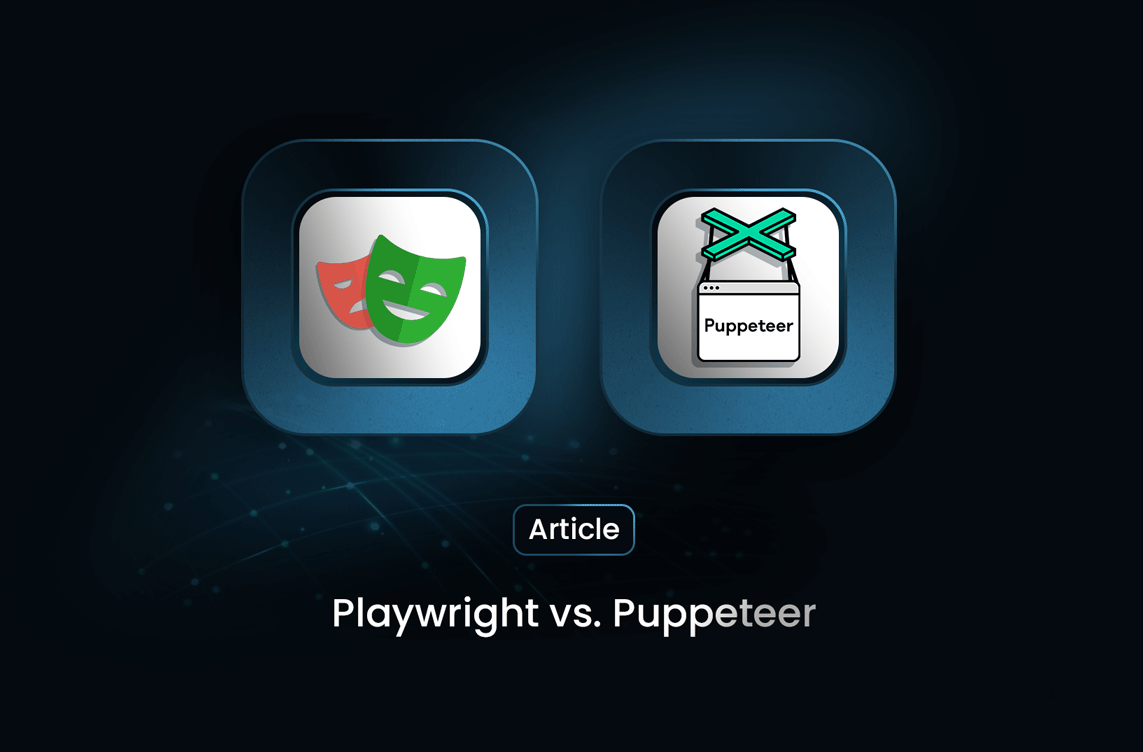
Playwright vs. Puppeteer: What Should I Use?
A detailed comparison of Playwright vs. Puppeteer for browser automation, web scraping, and testing. Learn their key differences, features, performance, and best use cases to choose the right tool for your project.
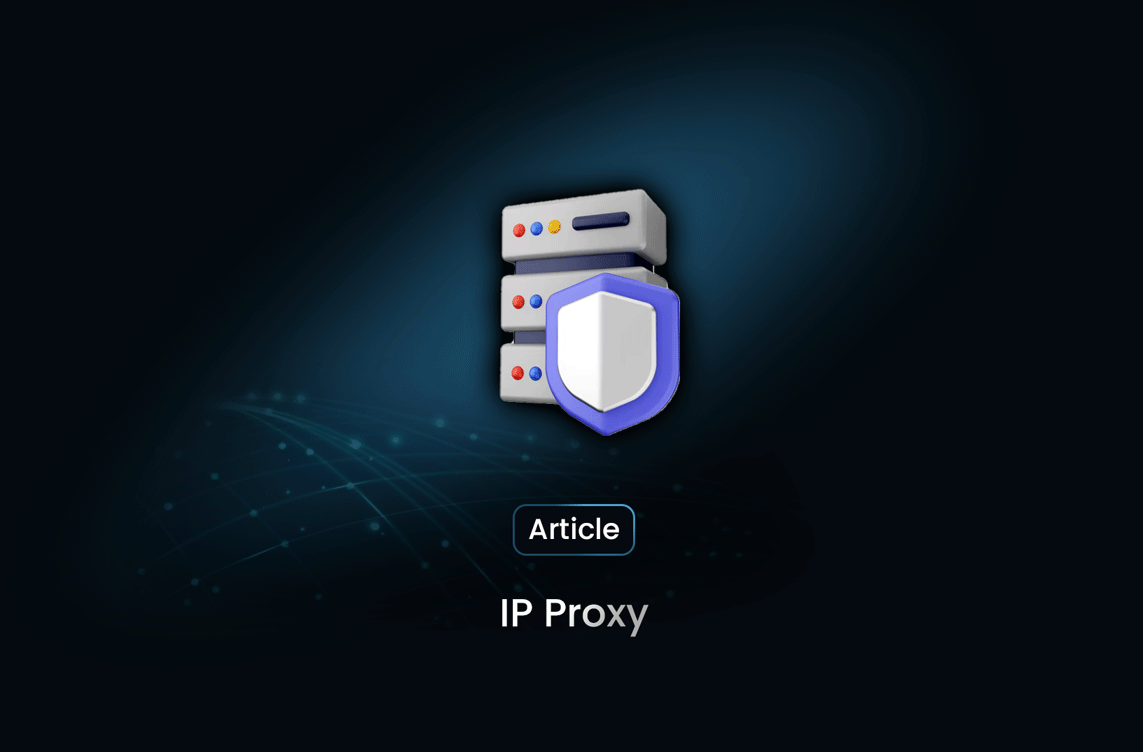
The Commercial Applications of Data Scraping Tools and the Role of IP Proxy Services
Discover how data scraping powers businesses and why IP proxies are essential for seamless, ethical, and efficient data extraction.
@MrScraper_
@MrScraper