JavaScript Web Scraping
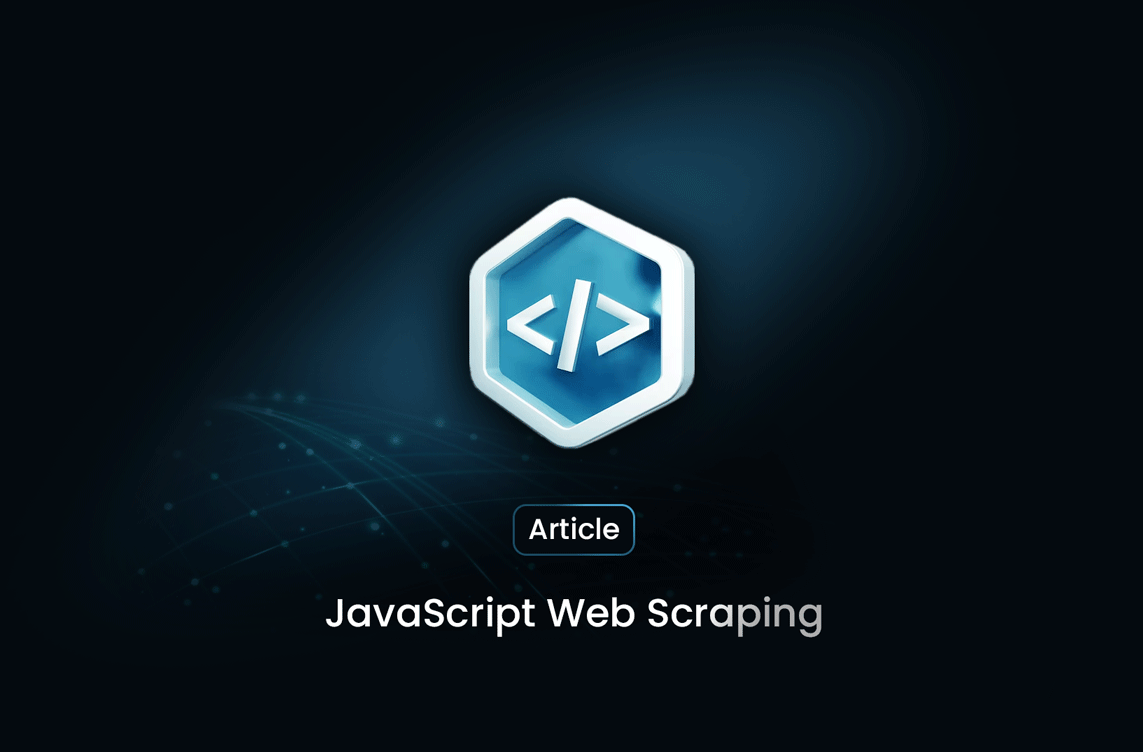
Web scraping is an essential tool for collecting data from websites, and JavaScript offers powerful libraries and techniques to accomplish this. This guide explores how to perform web scraping using JavaScript, the tools you need, and best practices.
What Is Web Scraping?
Web scraping is the process of extracting data from websites. With JavaScript, this often involves interacting with webpage elements, fetching data, and parsing it to extract meaningful information.
Why Use JavaScript for Web Scraping?
JavaScript is widely used for web development, making it an excellent choice for scraping web pages with dynamic content rendered by JavaScript. Here's why it’s beneficial:
- Native DOM Manipulation: Direct access to the Document Object Model (DOM) for precise data extraction.
- Event Simulation: Simulate user interactions like clicks and form submissions.
- Dynamic Rendering: Handle Single Page Applications (SPAs) efficiently.
Tools for JavaScript Web Scraping
Here are popular JavaScript tools and libraries for web scraping:
Tool | Description | Use Case |
---|---|---|
Puppeteer | A headless browser automation library for Chrome. | Scraping SPAs and taking screenshots. |
Cheerio | A lightweight library for parsing and manipulating HTML, similar to jQuery. | Scraping static websites. |
Playwright | A multi-browser library for headless browser automation. | Scraping with multi-browser compatibility. |
Axios | A promise-based HTTP client for making network requests. | Fetching APIs or web pages. |
Node.js | A JavaScript runtime for building scalable scraping scripts. | Backend scraping setups. |
Step-by-Step: Scraping with Puppeteer
Below is an example of how to scrape data using Puppeteer:
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
// Navigate to the website
await page.goto('https://example.com');
// Scrape data
const data = await page.evaluate(() => {
return Array.from(document.querySelectorAll('h1')).map(el => el.textContent);
});
console.log(data);
// Close the browser
await browser.close();
})();
Explanation:
- Launch Puppeteer: Starts a headless browser instance.
- Navigate to Website: Opens the desired webpage.
- Extract Data: Uses the
page.evaluate()
function to extract elements from the DOM. - Output Results: Logs the scraped data to the console.
Best Practices for JavaScript Web Scraping
- Respect Robots.txt: Always adhere to a website’s robots.txt rules to avoid violating terms of service.
- Add Delays: Avoid overwhelming servers with frequent requests by adding delays between actions.
- Handle CAPTCHAs: Use libraries like AntiCaptcha or 2Captcha to bypass CAPTCHAs if required.
- Use Proxies: Rotate proxies to prevent IP bans during extensive scraping.
- Avoid Personal Data: Ensure compliance with data privacy regulations like GDPR.
Use Cases for JavaScript Web Scraping
Use Case | Example |
---|---|
E-commerce Pricing | Scrape product prices from competitor websites. |
SEO Analysis | Extract meta tags and keywords for optimization. |
Content Aggregation | Collect blog posts or news articles. |
Social Media Data | Gather public profiles or posts for analysis. |
Conclusion
JavaScript is a powerful and flexible choice for web scraping, with libraries like Puppeteer and Cheerio enabling scraping from both static and dynamic websites. However, navigating more complex tasks, such as bypassing CAPTCHAs or managing large-scale data extraction, often requires complementary tools.
By pairing your JavaScript projects with solutions like Mrscraper, you can simplify these challenges. Its AI-driven approach streamlines the scraping process, letting you focus more on analyzing and utilizing the data rather than managing intricate setups. Whether you're an experienced developer or just starting, the right combination of tools can make web scraping more efficient and effective.
Table of Contents
Take a Taste of Easy Scraping!
Get started now!
Step up your web scraping
Find more insights here
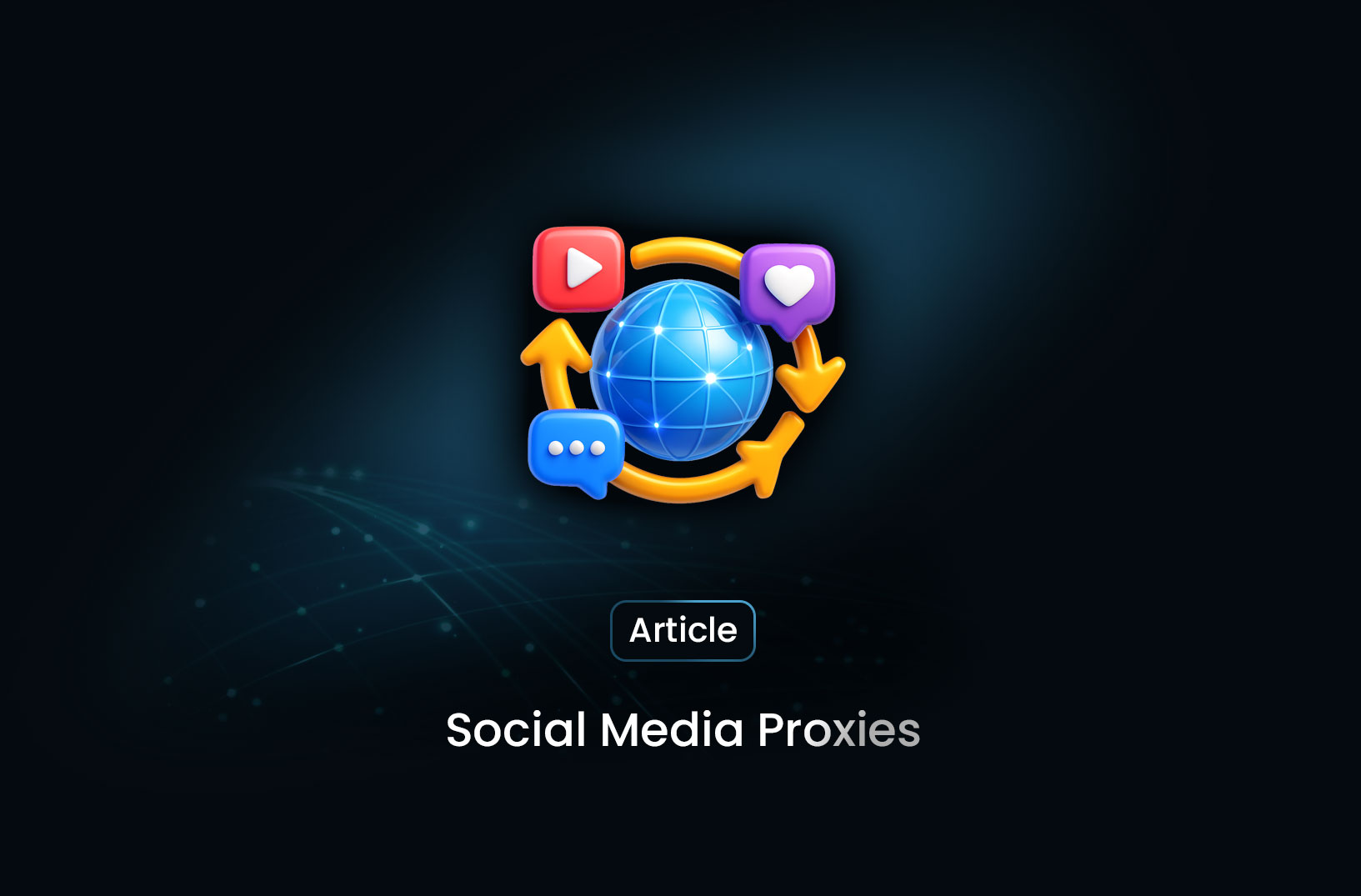
Social Media Proxies: Boost Privacy and Bypass Geo-Restrictions
Social media proxies are a cornerstone of modern online operations—be it account management, scraping, or ad campaigns.
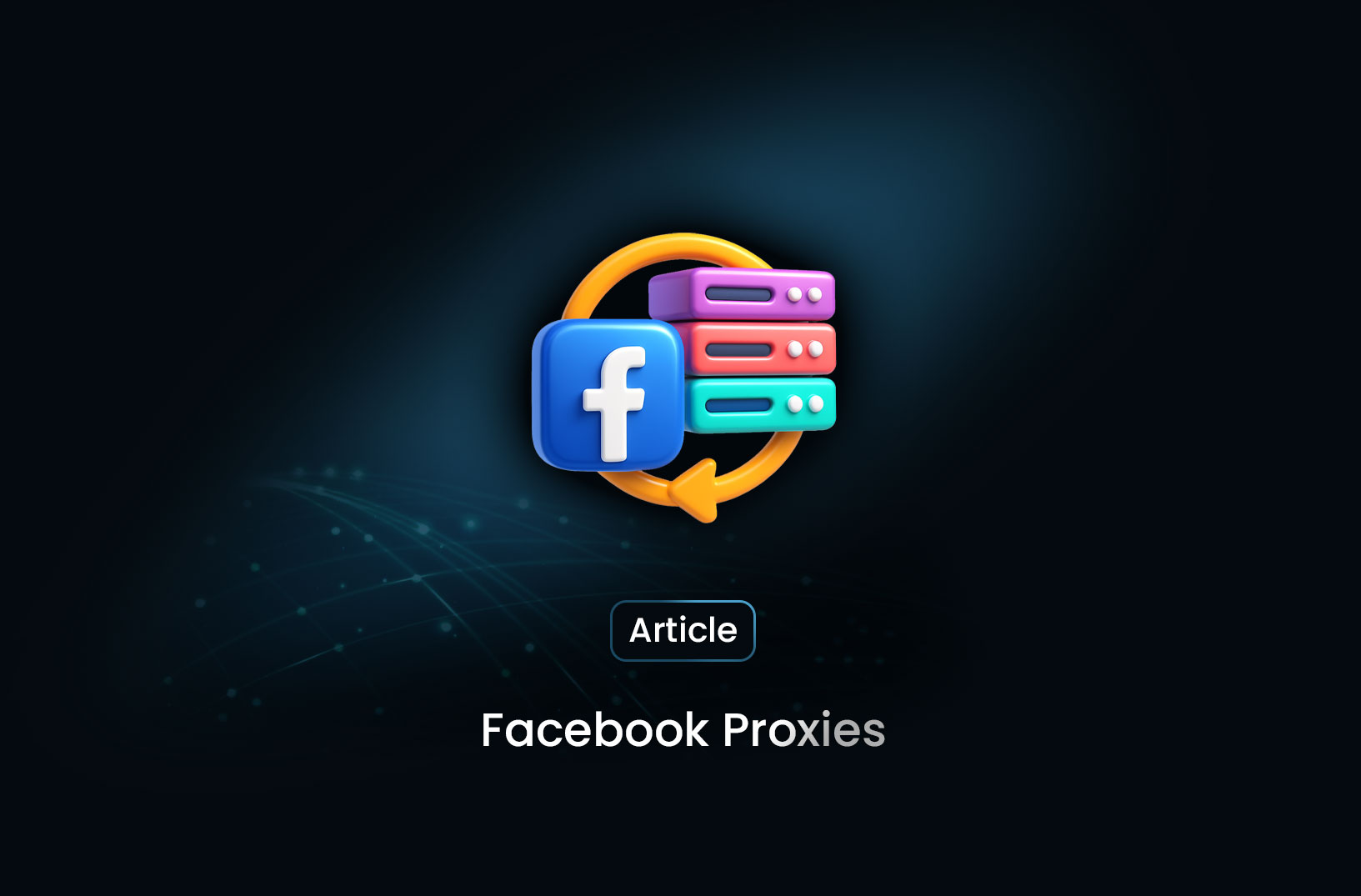
Best Facebook Proxies for Ads, Scraping, and Privacy in 2025
Facebook proxy routes your device’s Facebook traffic through an intermediary server. Instead of connecting directly, you use the proxy server to access Facebook, masking your real IP and location.
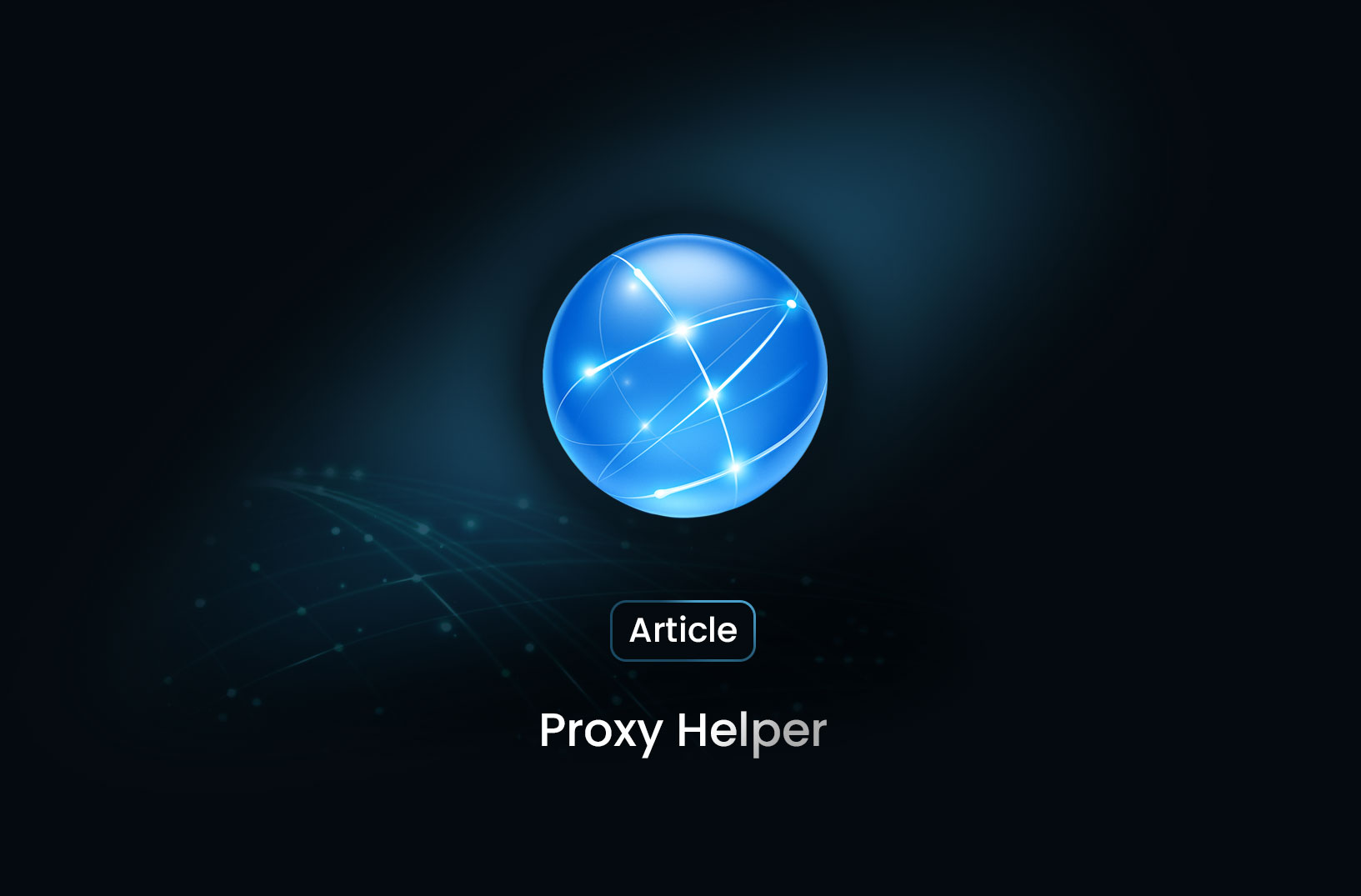
Proxy Helper: Your Chrome Extension for Browser-Level Proxy Control
Proxy Helper is a Chrome extension that allows users to configure and switch proxies directly within the browser, without touching system-wide settings.
@MrScraper_
@MrScraper