Instant Data Scraping Techniques: A Next.js Guide
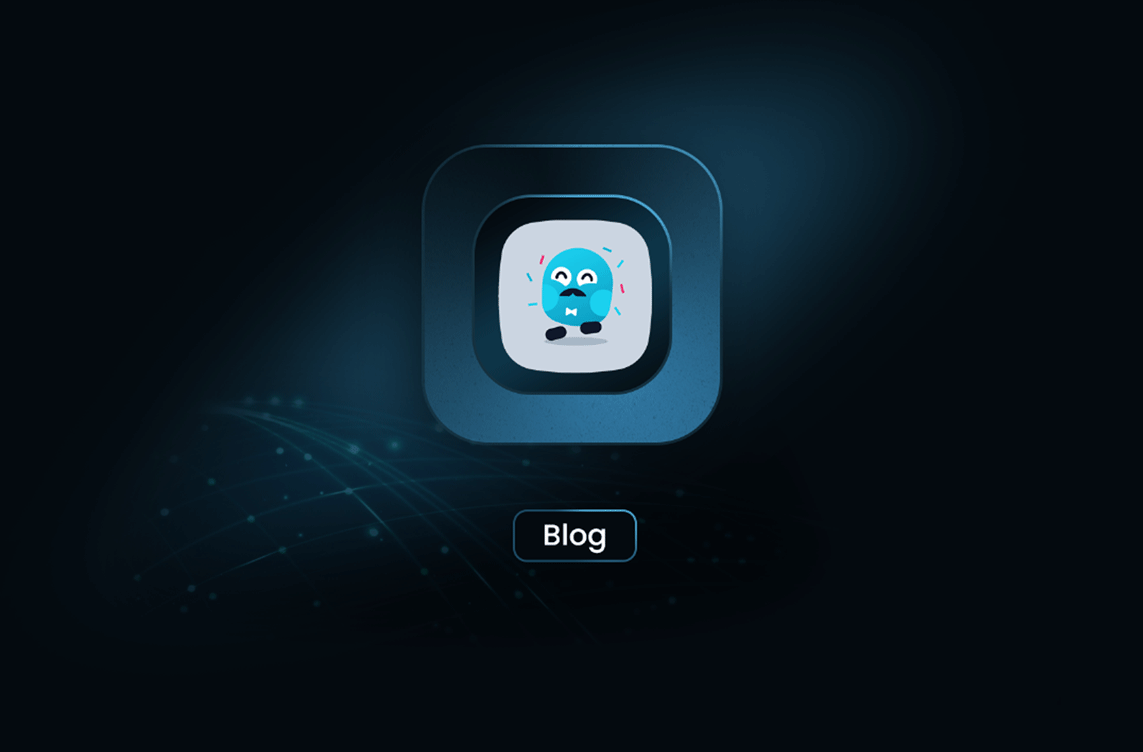
Data scraping, the process of extracting data from websites or APIs, has become an essential tool for businesses and developers alike. Instant data scraping, in particular, allows for real-time data acquisition, making it invaluable for applications requiring up-to-date information. In this blog post, we'll delve into various data scraping techniques and provide Next.js code examples to illustrate their implementation.
Web Scraping
Web scraping involves extracting data directly from HTML or XML content. Essentially, it allows you to gather structured or semi-structured data from web pages that don’t necessarily provide it through an API. While it's a versatile approach, it can be more complex due to dynamic content and potential website changes. Below is an example of a Next.js application to perform a web scraping task.
Example using Next.js:
import axios from 'axios';
import cheerio from 'cheerio';
async function scrapeProductData(url) {
try {
const response = await axios.get(url);
const $ = cheerio.load(response.data);
const title = $('h1.product-title').text();
const price = $('.product-price').text();
const description = $('.product-description').text();
return { title, price, description };
} catch (error) {
console.error('Error scraping:', error);
}
}
In this Next.js example, we’ll scrape product data from a hypothetical e-commerce website, focusing on title, price
, and description
using axios
for making HTTP requests and cheerio
to parse and traverse the HTML document. Here is a breakdown of the simple scraper example:
-
The axios.get(url) function is used to send a request to a given URL and retrieve the webpage's HTML content.
-
Once the webpage content is retrieved, the HTML is loaded into Cheerio for parsing. Cheerio allows us to select and extract specific elements using CSS selectors. Here, we can target and select specific parts of the HTML page by referring to their CSS classes or tags, for example:
- The title is extracted by selecting an
<h1>
tag with a class ofproduct-title
. - The price is captured from an element with the class
product-price
. - The description is pulled from an element with the class
product-description
.
- Once the required data is extracted, it is formatted into a Javascript Object. This object can then be used in different ways—such as displaying the data on a website, saving it into a database, or processing it further in your application.
API Scraping
API scraping is a more efficient and direct method for retrieving data, as it leverages APIs provided by websites or services. APIs are designed specifically to share data, offering structured information in formats like JSON or XML. Unlike web scraping, API scraping avoids the complexities of parsing HTML and reduces the risk of scraping errors due to website changes.
API scraping is ideal for situations where the data is available through an API because it is more reliable and often faster than scraping HTML content. In addition, APIs are typically designed to handle requests for data in real time, offering better scalability when working with large volumes of requests.
Example using Next.js:
async function fetchWeatherData(city) {
try {
const apiKey = 'YOUR_API_KEY';
const url = `https://api.openweathermap.org/data/2.5/weather?q=${city}&appid=${apiKey}`; const response = await axios.get(url); const data = response.data;
return data; }
catch (error) { console.error('Error fetching weather data:',
error);
}
}
In this example, we’re using Axios to make an HTTP request to the OpenWeatherMap API to retrieve real-time weather data for a given city. The request uses an API key for authentication, which is typical for most APIs that require user registration and an API key for access.Here is a breakdown of the simple scraper example:
-
Similar to web scraping, we use Axios to handle HTTP requests. Here, instead of retrieving raw HTML, we are accessing structured data in the form of JSON, which is easier to work with and doesn’t require complex parsing.
-
As opposed to web scraping, APIs often require authentication through an API key. Users need to register the API key and use it to access the data.
-
Once the request is sent, the API returns a response containing the weather data. Axios automatically parses this response as a JavaScript object, making it easy to work with.
A Comparison
Instant data scraping is a valuable technique for extracting real-time information. By understanding the various approaches and following best practices, developers can effectively leverage web scraping and API scraping to build powerful applications. Each of these techniques—Web Scraping and API Scraping—has its own strengths and use cases.
- Web scraping is ideal when dealing with websites that don’t offer structured APIs but can be slower and prone to breaking changes on the site.
- API scraping is the most efficient when available, offering structured data in real-time.
With Next.js, you can easily implement any of these scraping methods within API routes, giving you the power to build real-time data scraping solutions quickly and efficiently. However, there’s always a downside in building your own scraper app. Here is a few challenges worth considering in utilizing NextJS to build your own scraper:
- Maintenance: Websites and APIs change frequently, requiring constant updates to your scraper.
- Scalability: Handling large-scale scraping requires robust infrastructure and error handling.
- Compliance: Respect website terms of service and avoid scraping ethically sensitive data.
Introducing MrScraper: Your Instant Data Scraping Powerhouse
Building and maintaining your own scraper can be a challenging and time-consuming process. That's where MrScraper comes in—our instant data scraping solution designed for speed, efficiency, and ease of use. With a comprehensive suite of features, MrScraper simplifies your data extraction tasks, so you can focus on what matters most.
Key Features of MrScraper:
- Industry-leading Lead Generator: MrScraper's lead generation engine is designed to scrape and gather high-quality leads with enriched data for targeted marketing campaigns.
- AI-Powered Scraping: Harness the power of AI to scrape even the most complex websites. Our own ScrapeGPT AI enables you to scrape with only a prompt and zero coding requirements at all.
- User-Friendly Interface: Enjoy a simple point-and-click interface that makes data extraction quick and hassle-free, even for non-technical users.
- Scalable Infrastructure: Whether you're scraping a small dataset or handling large-scale projects, MrScraper’s infrastructure can scale to meet your needs without compromising performance.
Focus on Your Core Business, Let MrScraper Handle the Scraping
MrScraper frees you from the complexities of building and maintaining your own scraper. With its powerful features and user-friendly interface, you can focus on what matters most – your core business objectives.
Ready to experience the power of MrScraper? Visit our websiteto learn more and start your free trial today!
Table of Contents
Take a Taste of Easy Scraping!
Get started now!
Step up your web scraping
Find more insights here
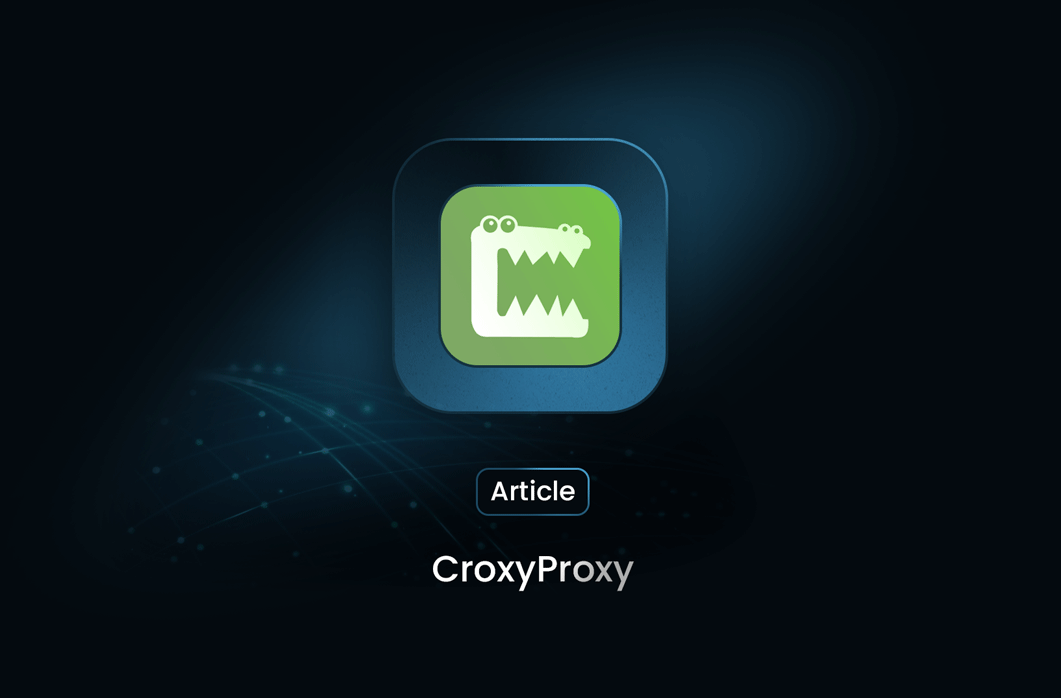
How to Use CroxyProxy: Complete with Usecase
CroxyProxy is a free web proxy service that provides secure and anonymous browsing by acting as an intermediary between the user and the website. This article will explore CroxyProxy, its features, a practical use case, and beginner-friendly steps to get started.
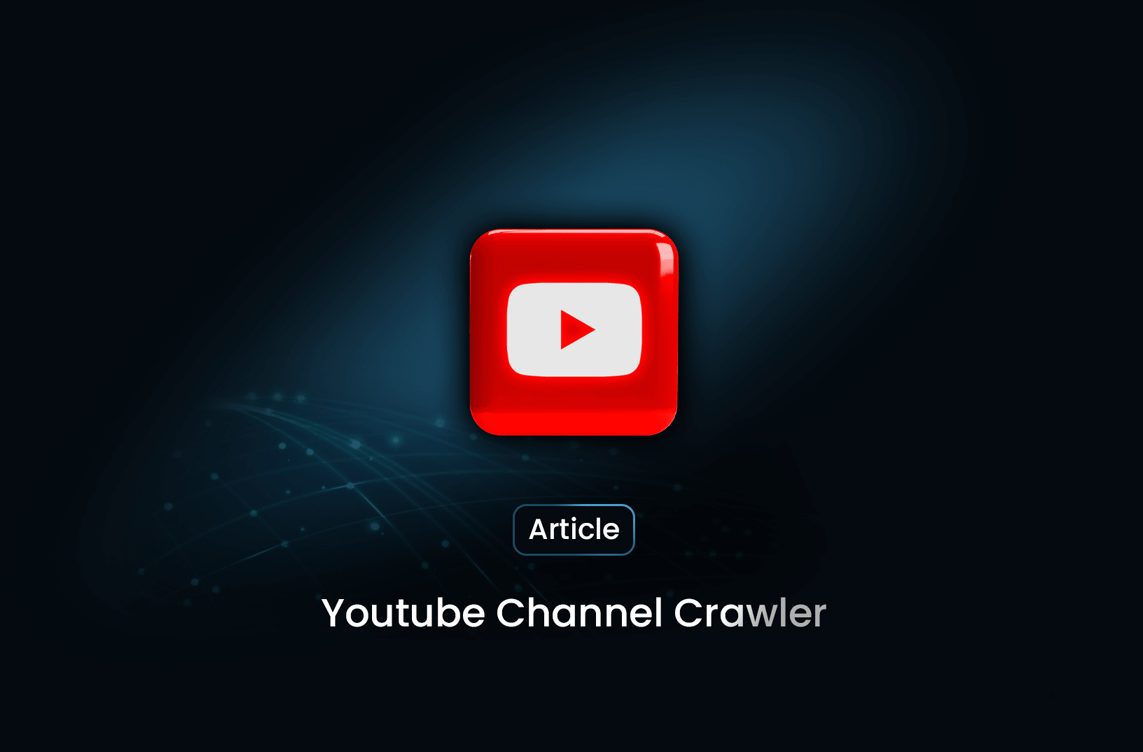
YouTube Channel Crawler
A YouTube channel crawler is a tool that automatically collects data from YouTube channels. It can extract information like video titles, descriptions, upload dates, views, likes, and comments, enabling efficient data analysis or research.
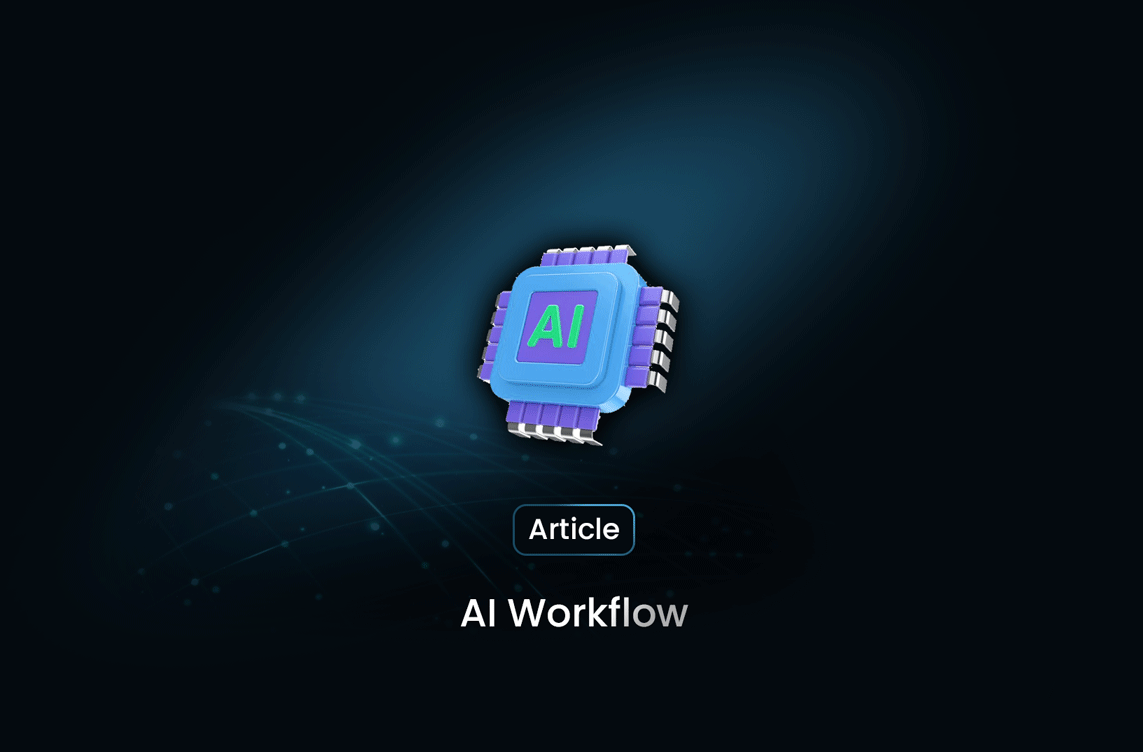
AI Workflow: Automating Customer Support with AI
Artificial Intelligence (AI) workflows are structured processes that guide the development, deployment, and usage of AI systems to solve specific problems or automate tasks. This guide provides a clear understanding of AI workflows, a practical use case, and simple, beginner-friendly steps to implement one.
@MrScraper_
@MrScraper