How to Use a Python HTML Parser for Web Scraping
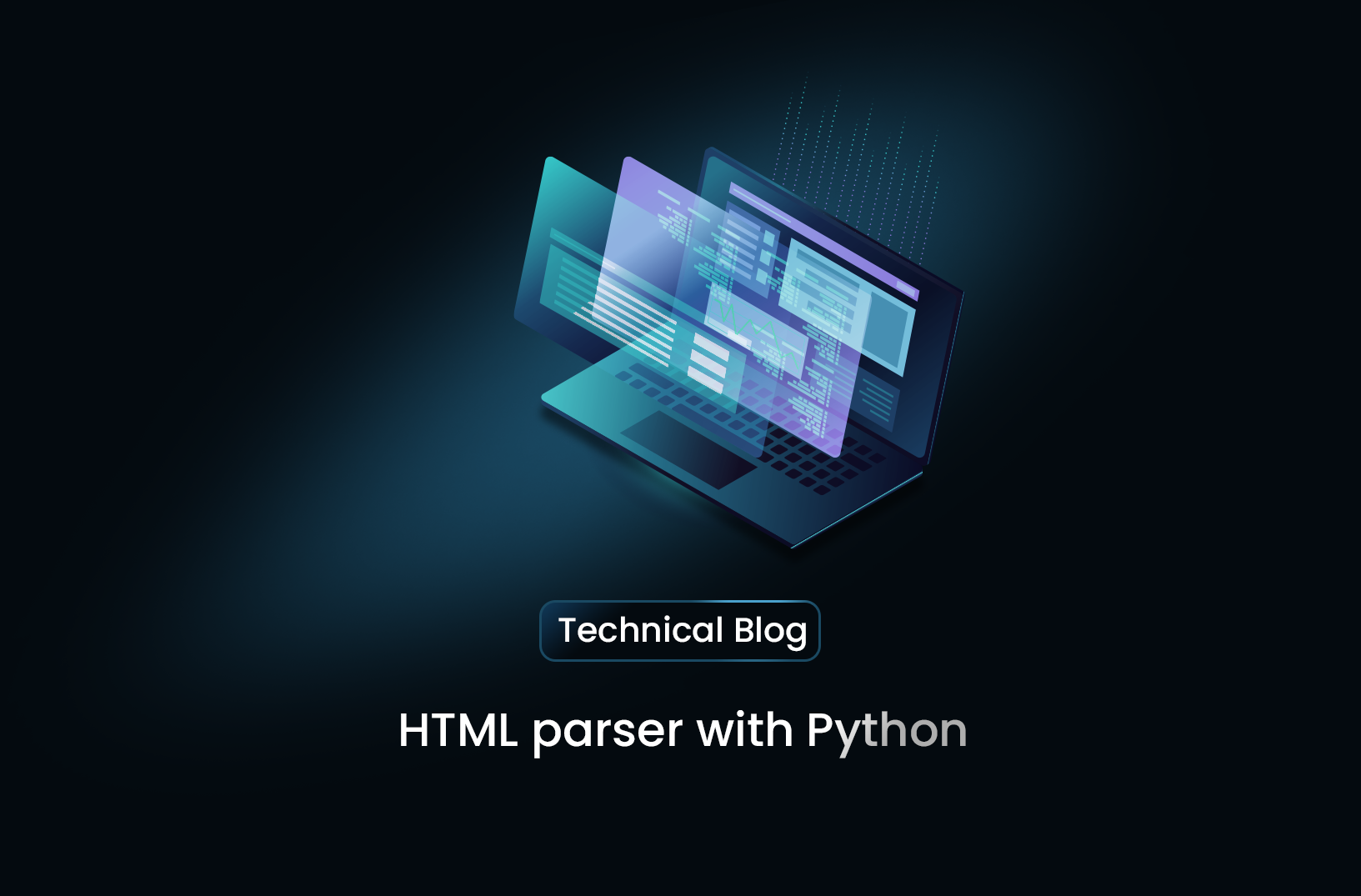
When it comes to web scraping, Python HTML parsers are a crucial tool for extracting data from websites. A Python HTML parser is used to break down and process the raw HTML content of a webpage so that you can retrieve useful information like text, images, links, and more. In this article, we will discuss Python HTML parsers and how to use them. We will also provide a complete code example using the popular BeautifulSoup
library to parse HTML content.
What is a Python HTML Parser?
A Python HTML parser reads and interprets the structure of HTML documents. It traverses the entire HTML tree, identifying elements like headings, paragraphs, images, and links, and allowing you to extract or modify specific data. These parsers make it easy to process web pages for data extraction.
There are various libraries available for Python HTML parsing, with the most popular being:
- BeautifulSoup: A library designed for quick HTML parsing and web scraping.
- lxml: Known for its fast processing and efficient handling of large files.
- html.parser: A built-in Python library that provides basic HTML parsing.
Why Use a Python HTML Parser for Scraping?
The internet is filled with information, but it's often presented in a format that makes it hard to extract data manually. A Python HTML parser allows you to automate this process, making it much easier to collect large amounts of data from websites. This can be especially useful for tasks like:
- Monitoring product prices
- Extracting contact information
- Gathering news articles or blog posts
- Analyzing social media trends
Using a Python HTML parser can streamline the collection and structuring of the data you need.
Installing the Python HTML Parser Library (BeautifulSoup)
For this tutorial, we’ll use BeautifulSoup
as the Python HTML parser. BeautifulSoup is a widely used library because of its simplicity and ability to handle poorly formed HTML.
You can install BeautifulSoup along with the requests
library (which helps in making HTTP requests to fetch web pages) by running:
pip install beautifulsoup4 requests
Writing a Complete Python HTML Parser Code
Let's walk through the steps of writing a Python HTML parser using BeautifulSoup to extract data from a webpage.
1. Importing Required Libraries
First, import the necessary libraries, including requests
for fetching web content and BeautifulSoup
for parsing it:
import requests
from bs4 import BeautifulSoup
2. Fetching the Web Page
Use the requests
library to fetch the raw HTML content of the page:
url = "https://en.wikipedia.org/wiki/Google"
response = requests.get(url)
#Check if the request was successful
if response.status_code == 200:
html_content = response.content
else:
print(f"Failed to retrieve the page. Status code: {response.status_code}")
3. Parsing the HTML
Once you have the HTML content, you can create a BeautifulSoup object to parse it:
soup = BeautifulSoup(html_content, 'html.parser')
The html.parser
is a built-in parser in Python, but you could also use lxml
or html5lib
for better performance with more complex websites.
4. Extracting Data from the HTML
With the parsed HTML, you can now start extracting specific elements, such as the title, headings, paragraphs, and links:
# Extract the title of the page
page_title = soup.title.text
print(f"Page Title: {page_title}")
# Extract all headings (h1 tags)
headings = soup.find_all('h1')
for heading in headings:
print(f"Heading: {heading.text}")
# Extract all paragraphs
paragraphs = soup.find_all('p')
for paragraph in paragraphs:
print(f"Paragraph: {paragraph.text}")
# Extract all links
links = soup.find_all('a')
for link in links:
print(f"Link: {link.get('href')} - Text: {link.text}")
5. Handling Dynamic Content
Some modern websites use JavaScript to dynamically load content after the initial HTML is delivered. Unfortunately, a simple Python HTML parser like BeautifulSoup cannot handle JavaScript. In such cases, you can use Selenium to interact with the webpage and render dynamic content.
Here’s a simple example of how to use Selenium to handle JavaScript content:
pip install selenium
from selenium import webdriver
# Set up the webdriver (for example, using Chrome)
driver = webdriver.Chrome()
# Load the webpage
driver.get('https://en.wikipedia.org/wiki/Google')
# Extract the rendered HTML
html_content = driver.page_source
soup = BeautifulSoup(html_content, 'html.parser')
# Extract data just like before
page_title = soup.title.text
print(f"Page Title: {page_title}")
# Close the browser window
driver.quit()
Why Parsing HTML Can Be Difficult
At first glance, HTML parsing seems straightforward. However, in real-world scraping scenarios, challenges arise:
- Unpredictable Structure: Websites vary in their structure, and even small changes in the HTML can break your parser.
- Anti-Scraping Measures: Many sites employ protections like CAPTCHAs or rate limiting, making it harder to access data.
- Dynamic Content: JavaScript-generated content can make parsing difficult since basic parsers like BeautifulSoup can only handle static HTML.
- Website Blockage: Sites may detect scraping attempts and block your IP, especially if you’re sending frequent requests.
Why MrScraper Is the Right Solution
Building and maintaining a Python HTML parser for every website you scrape can be incredibly challenging. Websites frequently change their structure, and the sheer volume of data to process can overwhelm your resources. MrScraper offers a complete solution for large-scale, hassle-free scraping:
- Scalable Web Scraping: No need to write custom parsers for each site. MrScraper adapts to website changes and scales effortlessly.
- Anti-Scraping Measures Bypassed: With advanced techniques to bypass CAPTCHAs, rate limiting, and other security features, MrScraper mostly can do uninterrupted data collection.
Using a Python HTML parser like BeautifulSoup makes web scraping easy for small-scale projects. However, once you start dealing with complex websites, JavaScript rendering, and large volumes of data, you’ll quickly realize the limitations of building parsers yourself.
If you’re looking for a powerful, scalable, and effortless solution to scrape any website, give MrScraper a try. By letting MrScraper handle the hard parts of web scraping, you can focus on what truly matters—using the data you collect.
Ready to simplify your scraping projects? Sign up for MrScraper today.
Table of Contents
Take a Taste of Easy Scraping!
Get started now!
Step up your web scraping
Find more insights here
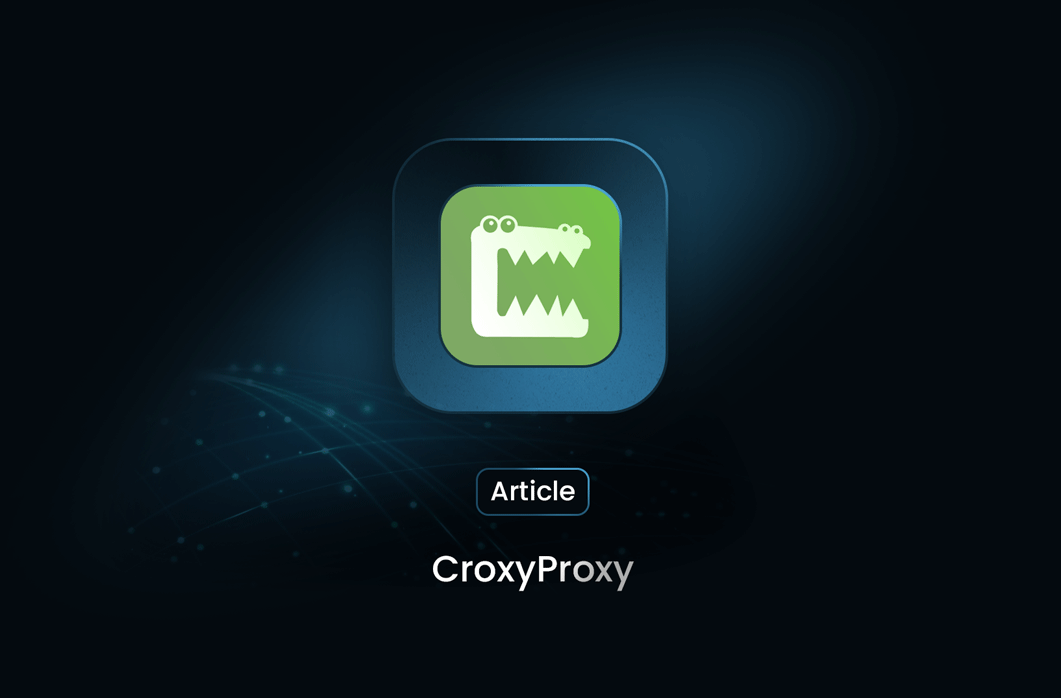
How to Use CroxyProxy: Complete with Usecase
CroxyProxy is a free web proxy service that provides secure and anonymous browsing by acting as an intermediary between the user and the website. This article will explore CroxyProxy, its features, a practical use case, and beginner-friendly steps to get started.
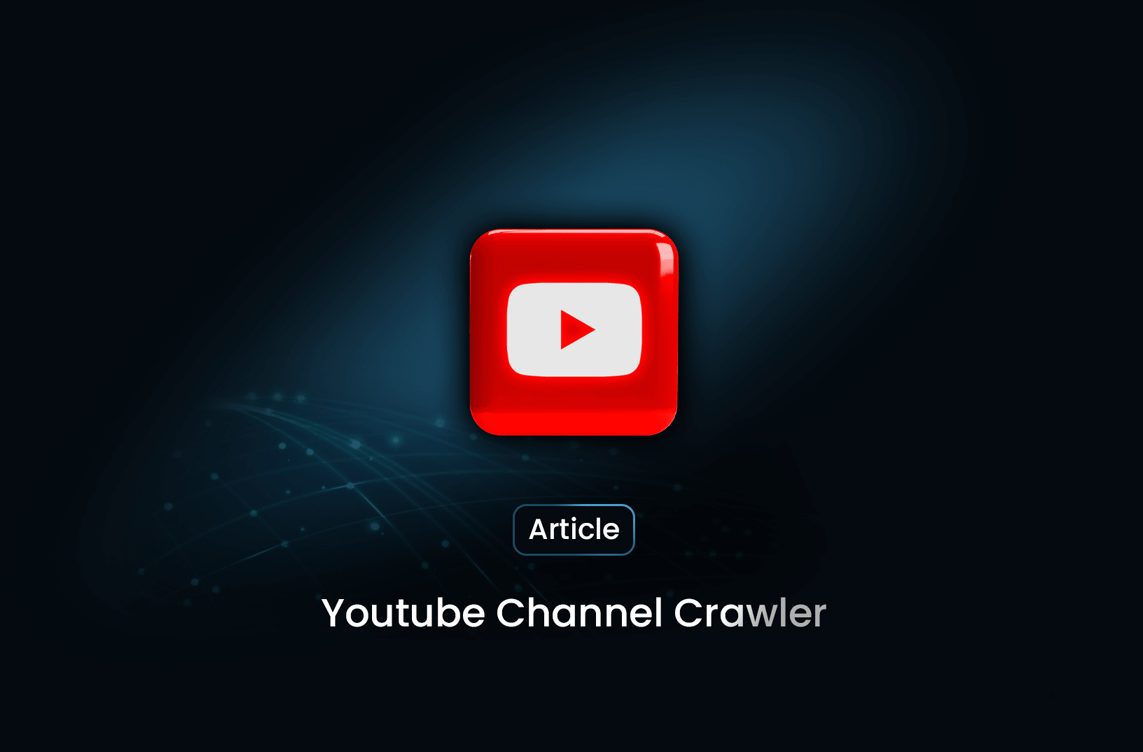
YouTube Channel Crawler
A YouTube channel crawler is a tool that automatically collects data from YouTube channels. It can extract information like video titles, descriptions, upload dates, views, likes, and comments, enabling efficient data analysis or research.
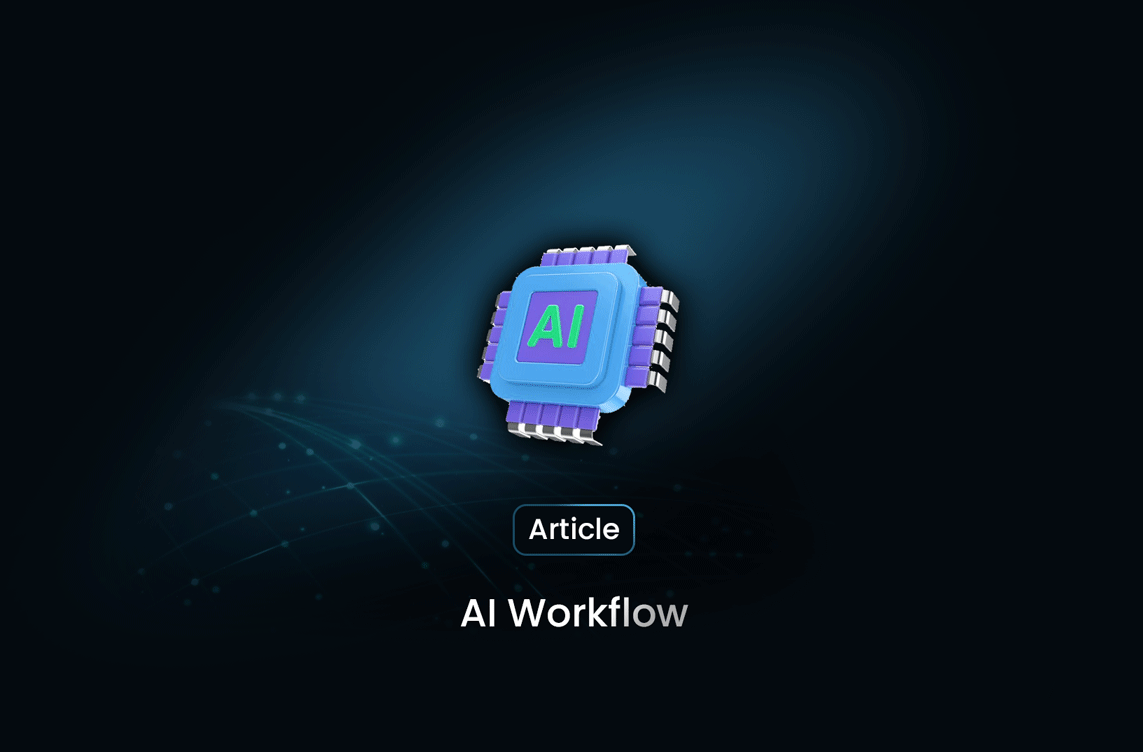
AI Workflow: Automating Customer Support with AI
Artificial Intelligence (AI) workflows are structured processes that guide the development, deployment, and usage of AI systems to solve specific problems or automate tasks. This guide provides a clear understanding of AI workflows, a practical use case, and simple, beginner-friendly steps to implement one.
@MrScraper_
@MrScraper