How to Parse XML with Python: A Beginner-Friendly Guide
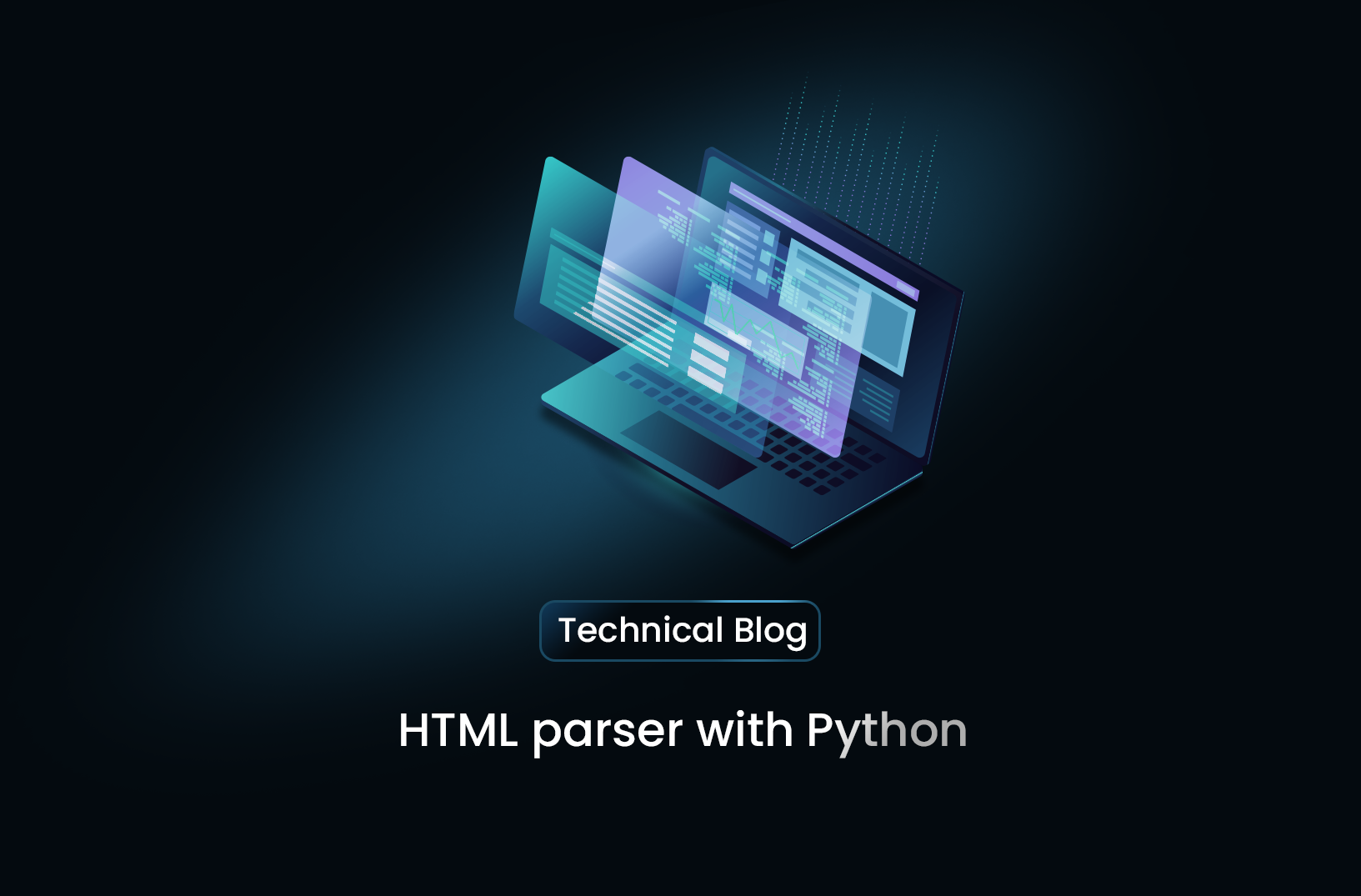
Extensible Markup Language (XML) is widely used for structuring data, especially in web services and data exchange. If you're working with XML in Python, knowing how to parse it efficiently is essential. Python provides multiple libraries for handling XML, with lxml
being one of the most powerful and efficient choices.
In this guide, we’ll explore how to parse XML using Python, focusing on lxml
for its speed, flexibility, and ease of use.
Why Use lxml
for XML Parsing?
Python offers several XML parsing libraries, such as xml.etree.ElementTree
and BeautifulSoup
. However, lxml
stands out due to:
-
Performance – Built on C libraries, it is significantly faster than built-in parsers.
-
XPath & XSLT Support – Allows advanced querying and transformation of XML data.
-
Robust Error Handling – Provides better validation and error messaging.
-
Easy Integration – Works seamlessly with web scraping libraries like
requests
andScrapy
.
Installing lxml
Before diving into XML parsing, install lxml
using pip:
pip install lxml
Parsing an XML File with lxml
Let's parse a sample XML document that contains book details:
Sample XML File (books.xml
)
<bookstore>
<book category="COOKING">
<title lang="en">Everyday Italian</title>
<author>Giada De Laurentiis</author>
<year>2005</year>
<price>30.00</price>
</book>
</bookstore>
Loading and Parsing XML in Python
from lxml import etree
# Load the XML file
tree = etree.parse("books.xml")
root = tree.getroot()
# Access elements
for book in root.iter("book"):
title = book.find("title").text
author = book.find("author").text
print(f"Title: {title}, Author: {author}")
Explanation:
etree.parse("books.xml")
loads the XML document..getroot()
retrieves the root element (<bookstore>
)..iter("book")
loops through all<book>
elements..find("title").text
extracts text inside<title>
.
Advanced XML Parsing with XPath
XPath is a powerful way to navigate and extract data from XML. Here’s how you can use XPath queries with lxml
:
# Find all book titles
book_titles = root.xpath("//book/title/text()")
print("Book Titles:", book_titles)
//book/title/text()
retrieves the text of all<title>
elements.
Filtering by Attributes
To find books under a specific category, use:
cooking_books = root.xpath('//book[@category="COOKING"]/title/text()')
print("Cooking Books:", cooking_books)
//book[@category="COOKING"]
selects books with the attributecategory="COOKING"
.
Handling Large XML Files with Iterative Parsing
For large XML files, use iterparse()
to process elements without loading the entire file into memory:
for event, element in etree.iterparse("books.xml", tag="book"):
title = element.find("title").text
print("Title:", title)
element.clear() # Free memory
iterparse()
processes elements one at a time, reducing memory usage.
Error Handling in XML Parsing
Handle parsing errors using try-except
:
try:
tree = etree.parse("invalid.xml")
except etree.XMLSyntaxError as e:
print("XML Parsing Error:", e)
- This prevents crashes when encountering malformed XML.
Parsing XML from a URL
If your XML data is online, use requests
with lxml
:
import requests
from lxml import etree
url = "https://example.com/data.xml"
response = requests.get(url)
root = etree.fromstring(response.content)
fromstring()
parses raw XML content from the response.
XML Parsing vs. JSON Parsing: When to Use What?
Feature | XML | JSON |
---|---|---|
Readability | Human & Machine | Mostly Machine |
Data Storage | Hierarchical | Key-Value Pairs |
Parsing Libraries | lxml , xml.etree |
json |
Web Services | Used in REST & SOAP APIs | Mostly REST APIs |
-
Use XML when working with structured hierarchical data or interacting with legacy systems.
-
Use JSON when dealing with modern web APIs for better readability and flexibility.
Conclusion
Python provides several libraries for XML parsing, with lxml
being the most efficient and feature-rich. Whether you are processing small XML files or handling large datasets, mastering XML parsing is crucial for web scraping, data extraction, and API integration.
Key Takeaways:
lxml
is the best choice for performance and advanced XML features.- Use XPath for precise XML data extraction.
- For large files, iterparse() reduces memory usage.
- Proper error handling ensures robust parsing.
Start implementing XML parsing today and streamline your data processing tasks!
Table of Contents
Take a Taste of Easy Scraping!
Get started now!
Step up your web scraping
Find more insights here
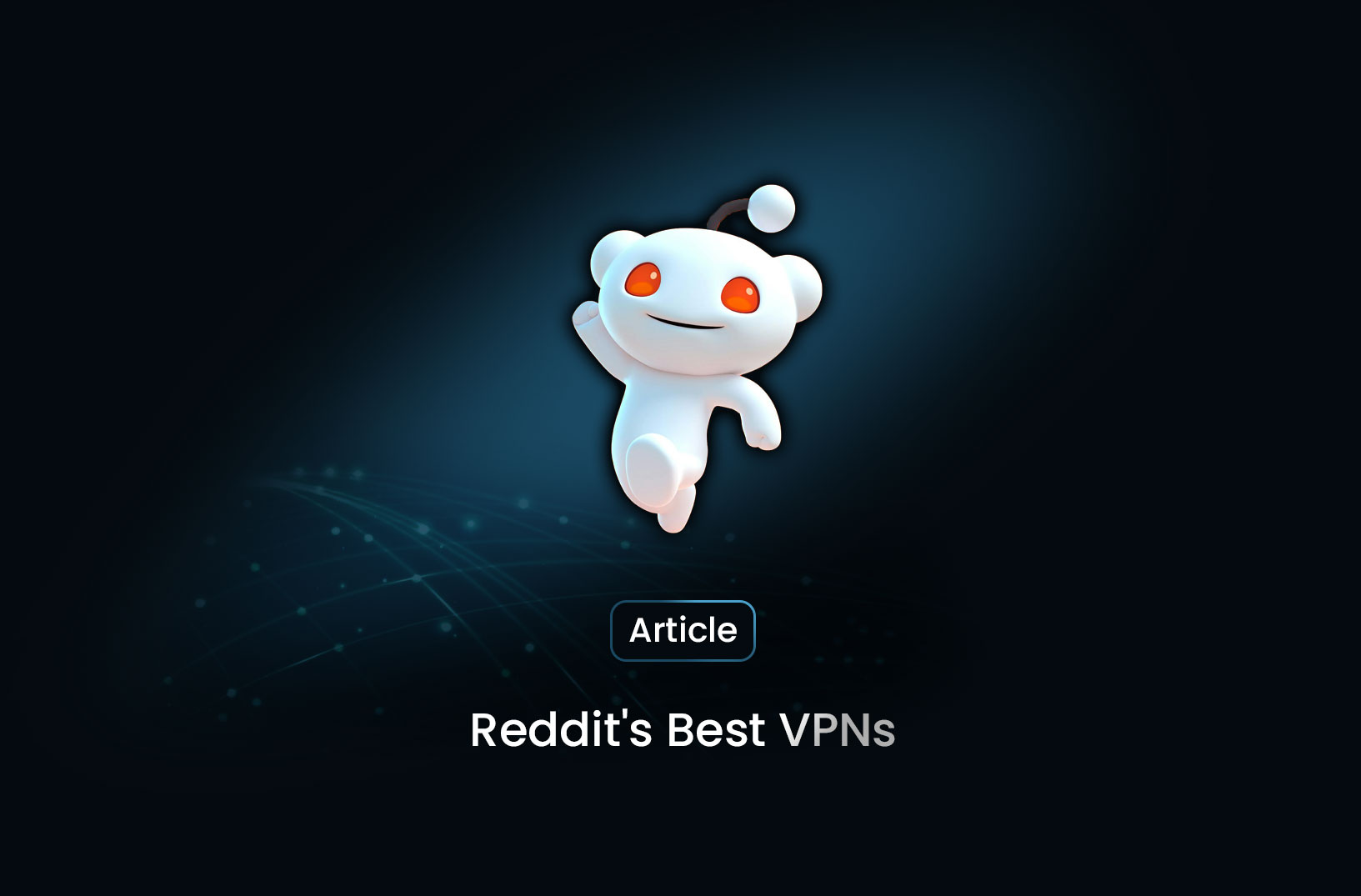
Reddit's Best VPNs in 2025: What Real Users Recommend
Discover the best VPNs according to Reddit users in 2025. Compare top-rated services like NordVPN, Surfshark, and ProtonVPN based on real user feedback, privacy, speed, and scraping compatibility. Learn what VPN Redditors trust most—and why.
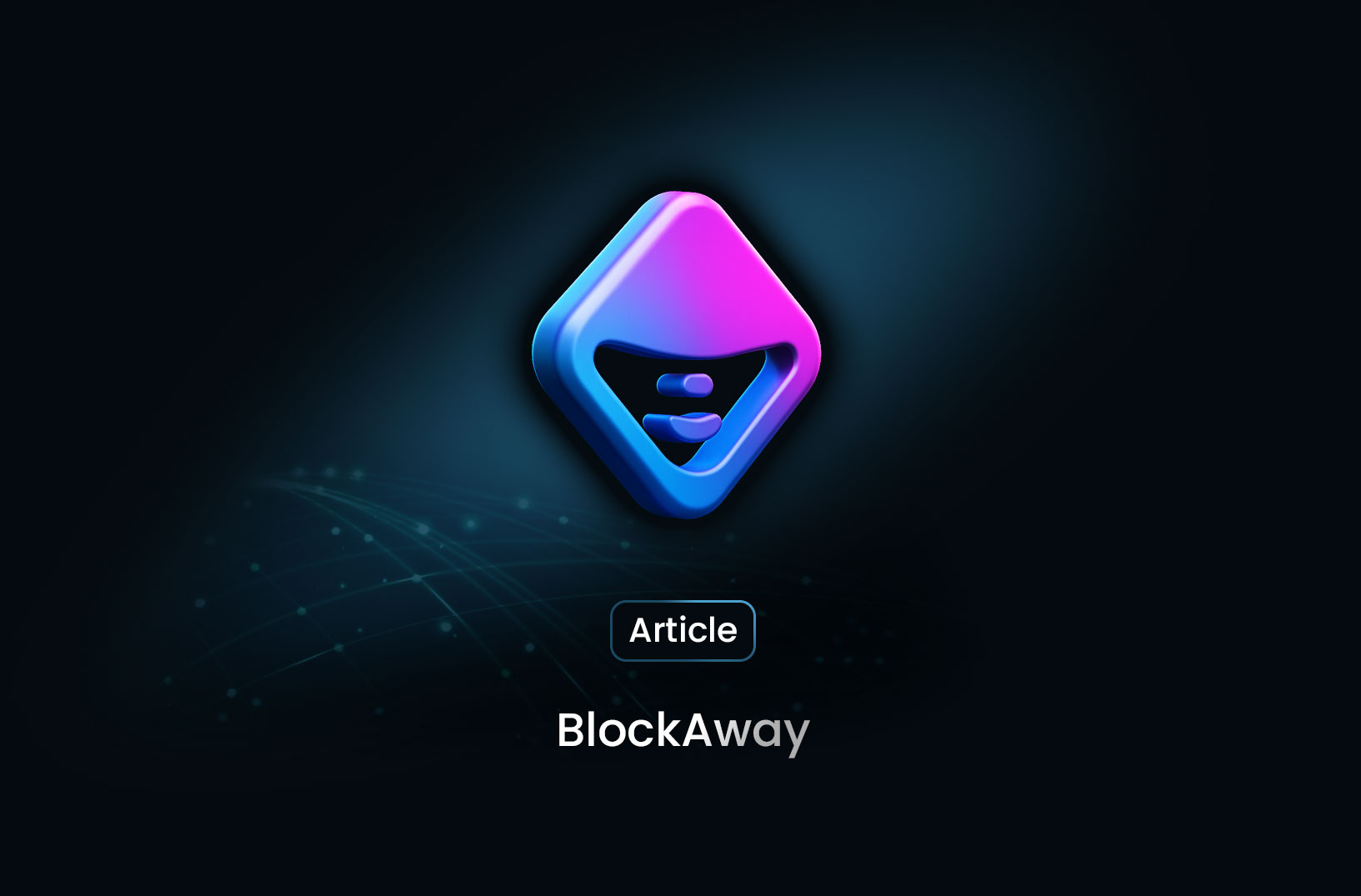
BlockAway: Is It Worth Using in 2025?
BlockAway is a web proxy, not a VPN. It works entirely through your browser. You type in the website you want to visit, and BlockAway fetches the content for you, masking your IP and bypassing restrictions in the process.
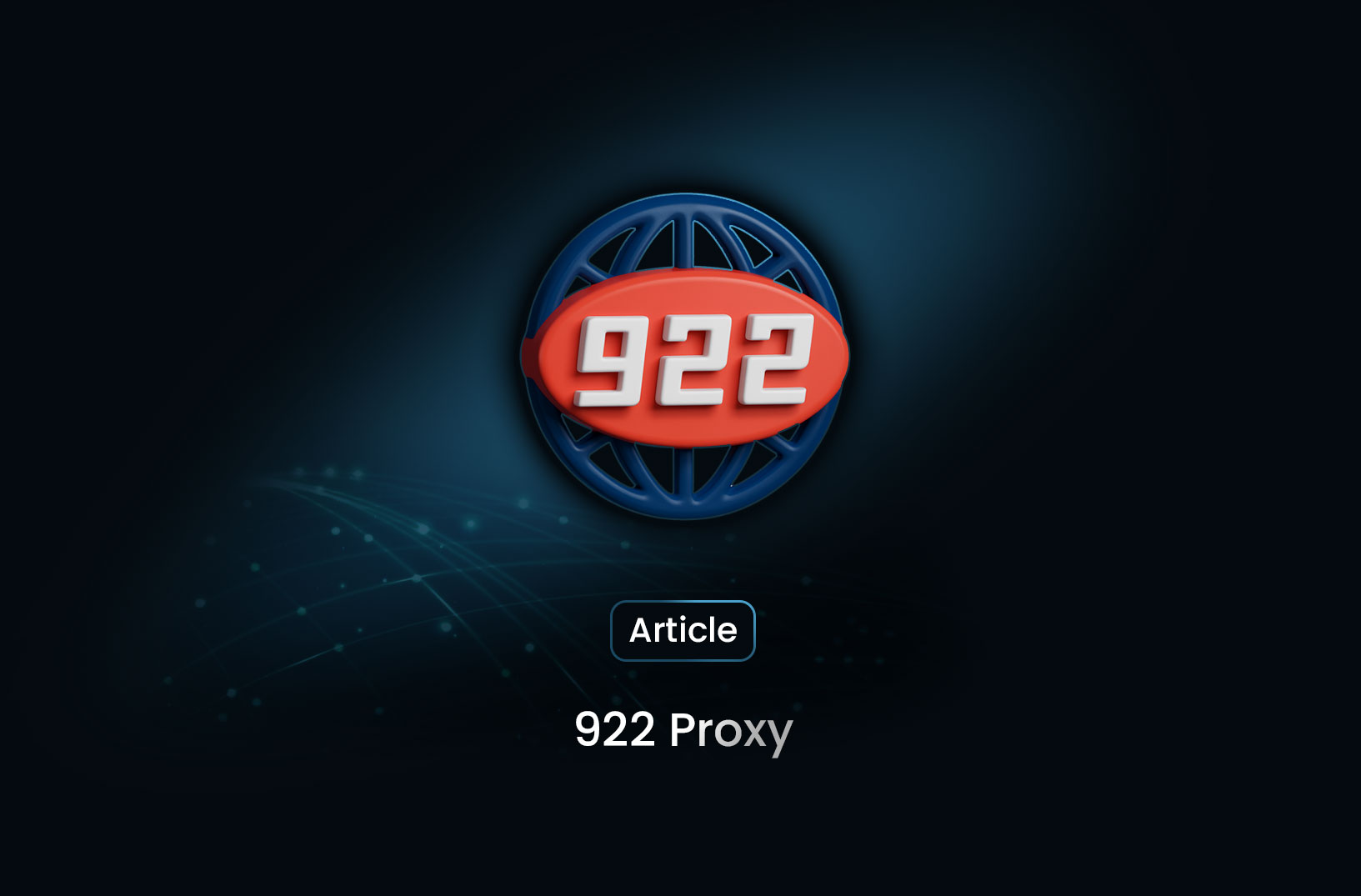
922 Proxy: High-Performance Proxies for Scalable Web Scraping
Discover how 922 Proxy supports scalable web scraping with residential, ISP, and rotating IPs. Learn how MrScraper uses 922 Proxy to extract data from ShopeeFood, GrabFood, and more.
@MrScraper_
@MrScraper