Facebook Scraper: A Comprehensive Guide to Web Scraping on Facebook
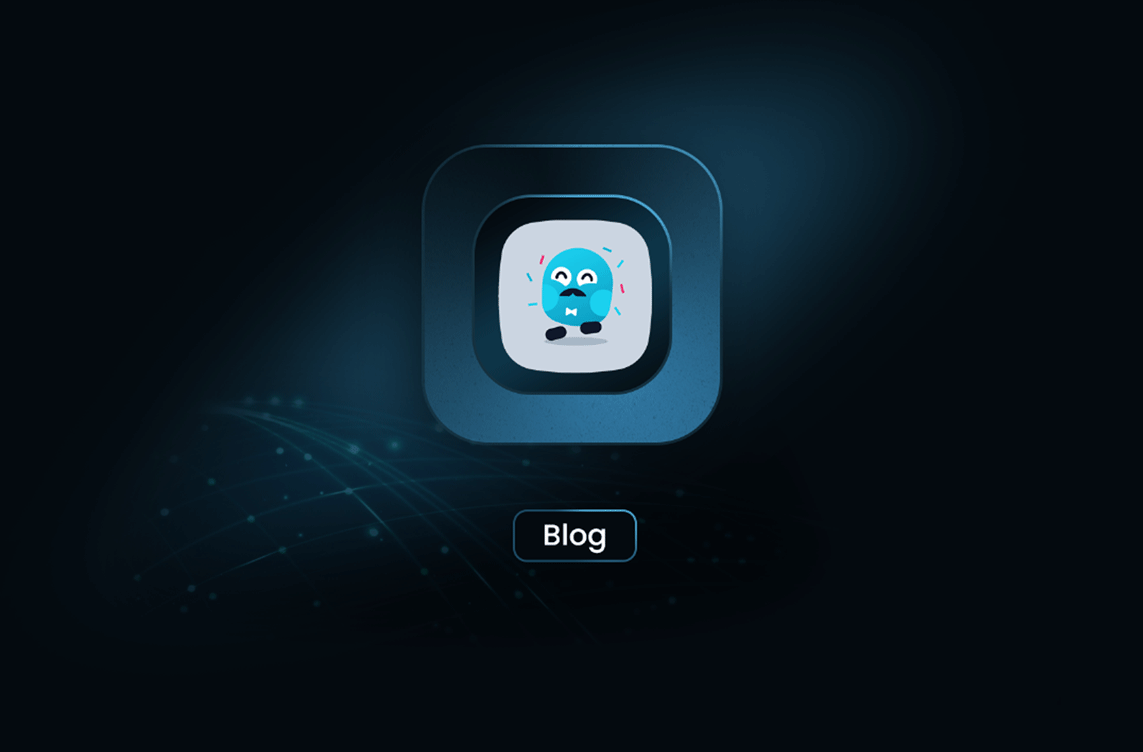
With the increasing reliance on data for decision-making, scraping publicly available information from platforms like Facebook has become a common practice for businesses, marketers, and researchers. Facebook scrapers are tools or scripts designed to extract specific information such as user profiles, posts, likes, comments, and other public data. In this article, we'll dive into the technical details of creating and using a Facebook scraper and compare it with API-based scraping solutions like MrScraper's Facebook Marketplace scraper.
Disclaimer
Before starting, it's essential to emphasize the ethical and legal aspects of web scraping. Facebook’s terms of service prohibit unauthorized scraping of its content, and violations can result in account bans, legal actions, or more severe penalties. Make sure to follow Facebook's policies and scrape data only with proper permissions.
1. Understanding How Facebook Works
Before building a scraper, you need to understand how Facebook serves its content:
- GraphQL and REST API: Facebook primarily uses GraphQL, but some parts still rely on REST APIs for data requests.
- Dynamic Content Loading: Much of Facebook's content is dynamically loaded using JavaScript and AJAX, making scraping HTML challenging without handling these dynamic behaviors.
- Rate Limiting: Facebook has strict rate limits, and any violation might lead to IP blocking or account suspension.
2. Facebook’s Graph API
If you're looking to scrape public Facebook data ethically and within terms, the Graph API is the official and recommended way to do so.
- Access Tokens: All API requests require an access token. You can obtain one by creating a Facebook App and requesting specific permissions.
- Common Data Types: Posts, comments, reactions, pages, groups, and events.
- Rate Limiting and Pagination: The API limits the number of requests per app/user. Implementing pagination and respecting rate limits are crucial for effective scraping.
3. Scraping Without the API: Challenges and Tools
Although the Graph API is the official way to access Facebook data, some users may opt for scraping using tools or scripts: *** Challenges with Direct HTML Scraping**:
- JavaScript-Rendered Content: Tools like BeautifulSoup may struggle to scrape content that is rendered via JavaScript.
- Dynamic Loading: You'll need a solution that can handle asynchronous content loads, such as Selenium or Puppeteer.
- Anti-Scraping Techniques: Facebook implements measures like CAPTCHAs, dynamic user-agent checks, IP blocking, and bot-detection algorithms.
4. Setting Up a Web Scraper
There are several tools and libraries available for building Facebook scrapers. Here’s a basic technical walkthrough using Python:
a. Libraries and Tools
- Selenium: Automates browser interactions, simulates a real user, and handles dynamic content.
- BeautifulSoup: Parses static HTML.
- Puppeteer: A Node.js-based tool for headless Chrome scraping, useful for handling complex dynamic content.
b. Code Example with Selenium (Python)
Here’s an example that shows how to scrape public Facebook posts using Selenium.
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
import time
#Setup WebDriver (Chrome in this case)
driver = webdriver.Chrome(executable_path='/path/to/chromedriver')
#Open Facebook
driver.get("https://www.facebook.com")
#Login (if necessary)
email_input = driver.find_element_by_id("email")
password_input = driver.find_element_by_id("pass")
email_input.send_keys("your-email")
password_input.send_keys("your-password")
password_input.send_keys(Keys.RETURN)
time.sleep(3) # wait for login
#Navigate to a specific page or profile
driver.get("https://www.facebook.com/someprofile")
time.sleep(5)
#Scrape content (for example, post text)
posts = driver.find_elements_by_xpath("//div[@data-testid='post_message']")
for post in posts:
print(post.text)
#Close browser
driver.quit()
5. Handling JavaScript and Dynamic Content with Puppeteer
If you're dealing with heavy dynamic content rendering, Puppeteer can be a better alternative than Selenium. Puppeteer runs a headless browser and enables control over navigation, interaction, and scraping of JS-based content.
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch({ headless: true });
const page = await browser.newPage();
await page.goto('https://www.facebook.com');
// Perform login
await page.type('#email', 'your-email');
await page.type('#pass', 'your-password');
await page.click('button[name="login"]');
await page.waitForNavigation();
// Go to a Facebook page or profile
await page.goto('https://www.facebook.com/someprofile');
// Extract post content
const posts = await page.evaluate(() => {
let postElements = document.querySelectorAll('div[data-testid="post_message"]');
let postTexts = [];
postElements.forEach(post => postTexts.push(post.innerText));
return postTexts;
});
console.log(posts);
await browser.close();
})();
6. Avoiding Detection and Bypassing Scraping Blocks
Facebook actively implements anti-bot and anti-scraping measures. Here are some ways to avoid detection:
- Rotating Proxies: To avoid getting IP-blocked, use services that provide rotating proxies.
- Randomized User Agents: Mimic different browsers and devices by randomly switching user agents.
- Headless Detection: Modify browser settings in Selenium or Puppeteer to make it harder for Facebook to detect you’re using a bot (e.g., disable headless mode, tweak headers).
7. Rate Limiting and Best Practices
To ensure that your scraper runs smoothly and avoids detection:
- Respect Rate Limits: Implement pauses between requests to avoid raising red flags.
- Error Handling: Handle cases where content is unavailable or the account is logged out.
- IP Rotation: Use services like ScraperAPI or Bright Data to rotate proxies.
8. Data Storage and Management
Once the data is scraped, you’ll need to store it efficiently for analysis:
- Relational Databases (MySQL, PostgreSQL): Good for structured, normalized data.
- NoSQL Databases (MongoDB): Useful for unstructured or semi-structured data like JSON objects from posts or comments.
- Data Pipelines: Set up data pipelines with ETL (Extract, Transform, Load) processes to clean and store the data for downstream usage.
Comparison with MrScraper’s Facebook Marketplace API
In contrast to custom scraping techniques, MrScraper's API for Facebook Marketplace offers a structured and efficient solution for scraping Facebook data. Below is a summary of how MrScraper simplifies the scraping process:
- Endpoint-Based Scraping: MrScraper provides ready-to-use endpoints that allow you to easily create and run scrapers without worrying about the technical challenges of setting up bots, handling dynamic content, or evading anti-scraping measures.
Facebook Marketplace Scraper: The /api-reference/endpoint/facebook-marketplace
endpoint is specifically designed for scraping data from Facebook Marketplace. It requires the user to specify a scraper task that runs automatically and captures marketplace listings such as product titles, descriptions, prices, locations, and seller information.
For example, here's an API request to create and run a Facebook Marketplace scraper:
POST https://api.mrscraper.com/facebook-marketplace/scraper
Content-Type: application/json
Authorization: Bearer YOUR_API_KEY
{
"search_term": "laptop",
"location": "New York",
"radius": 50,
"max_results": 100
}
- Automation and Error Handling: MrScraper handles the heavy lifting by managing retries, error handling, and proxy management, so users don’t need to write extensive code to deal with CAPTCHAs or blocked requests.
- API Response: The scraped data is returned in a structured JSON format, which makes it easy to integrate directly into databases or other applications for further analysis.
Feature | Custom Web Scraping (Selenium/Puppeteer) | MrScraper API (Facebook Marketplace) |
---|---|---|
Setup | Requires custom code and browser automation | Pre-built API, no setup needed |
Dynamic Content Handling | Requires JavaScript handling libraries (e.g., Puppeteer) | Built-in handling of dynamic content |
Error Handling | Requires manual retries and CAPTCHA handling | Automatic retries, error handling, and proxy rotation |
Rate Limiting | Must be manually managed | Managed by the API |
Data Structure | Raw HTML or JSON | Structured JSON output |
Ease of Use | Requires technical expertise | Simple API integration |
Scraping Scope | Any data you can access via Facebook | Limited to predefined endpoints (e.g., Marketplace) |
Conclusion
While custom web scraping using tools like Selenium or Puppeteer offers flexibility, it comes with a lot of technical challenges, including dealing with dynamic content, CAPTCHA avoidance, and rate limits. MrScraper's Facebook Marketplace API, on the other hand, provides a simpler, more reliable, and legally safer way to extract structured data from Facebook, particularly the Marketplace.
For those looking for a straightforward solution to extract data without getting involved in the complexities of web scraping, MrScraper's API is a great alternative. However, if your scraping needs go beyond what the API offers (such as scraping user profiles, comments, or posts), you may still need to opt for custom scraping approaches.
Table of Contents
Take a Taste of Easy Scraping!
Get started now!
Step up your web scraping
Find more insights here
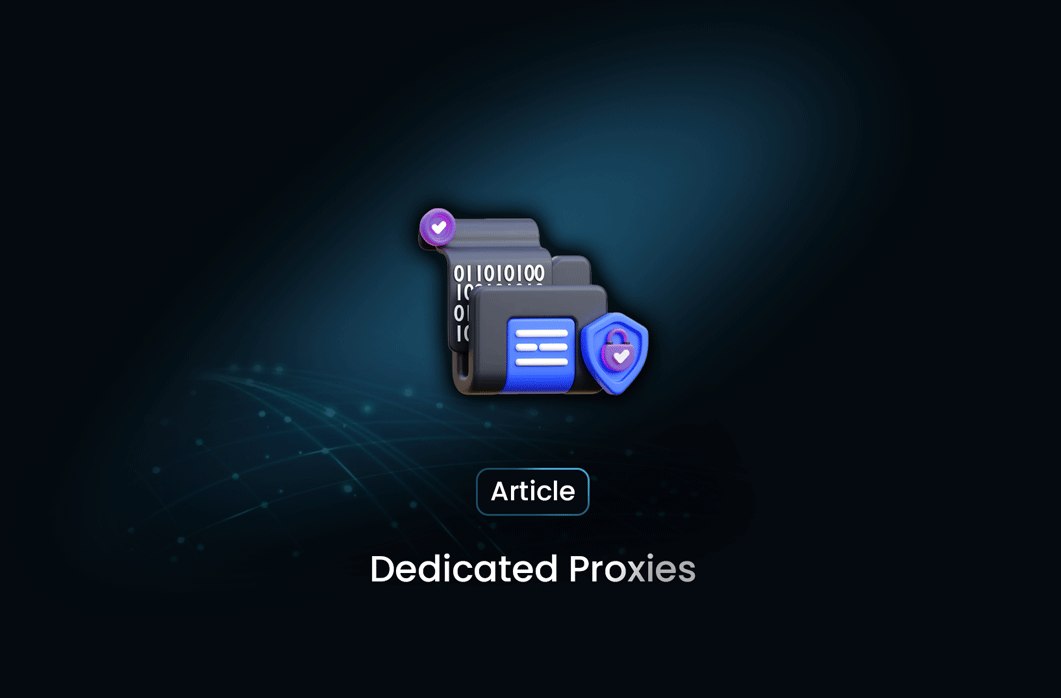
Dedicated Proxies: Benefits, Use Cases, and Setup
A dedicated proxy is an IP address exclusively assigned to a single user or entity. Unlike shared proxies, where multiple users share the same IP address, dedicated proxies ensure that only one user can utilize the proxy, offering enhanced speed, security, and anonymity.
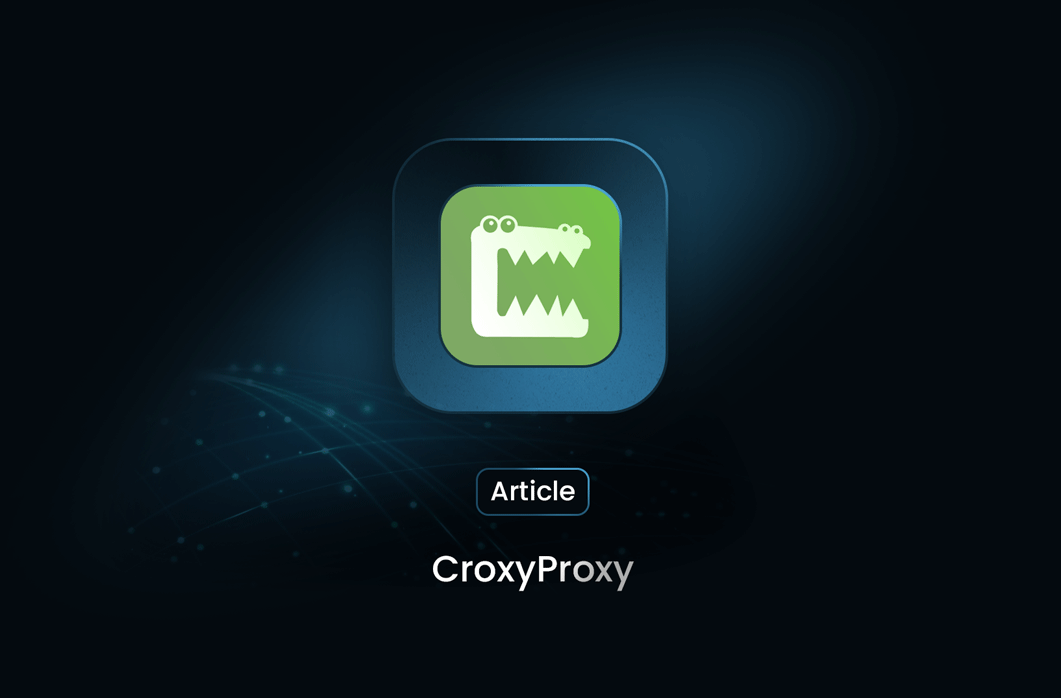
How to Use CroxyProxy: Complete with Usecase
CroxyProxy is a free web proxy service that provides secure and anonymous browsing by acting as an intermediary between the user and the website. This article will explore CroxyProxy, its features, a practical use case, and beginner-friendly steps to get started.
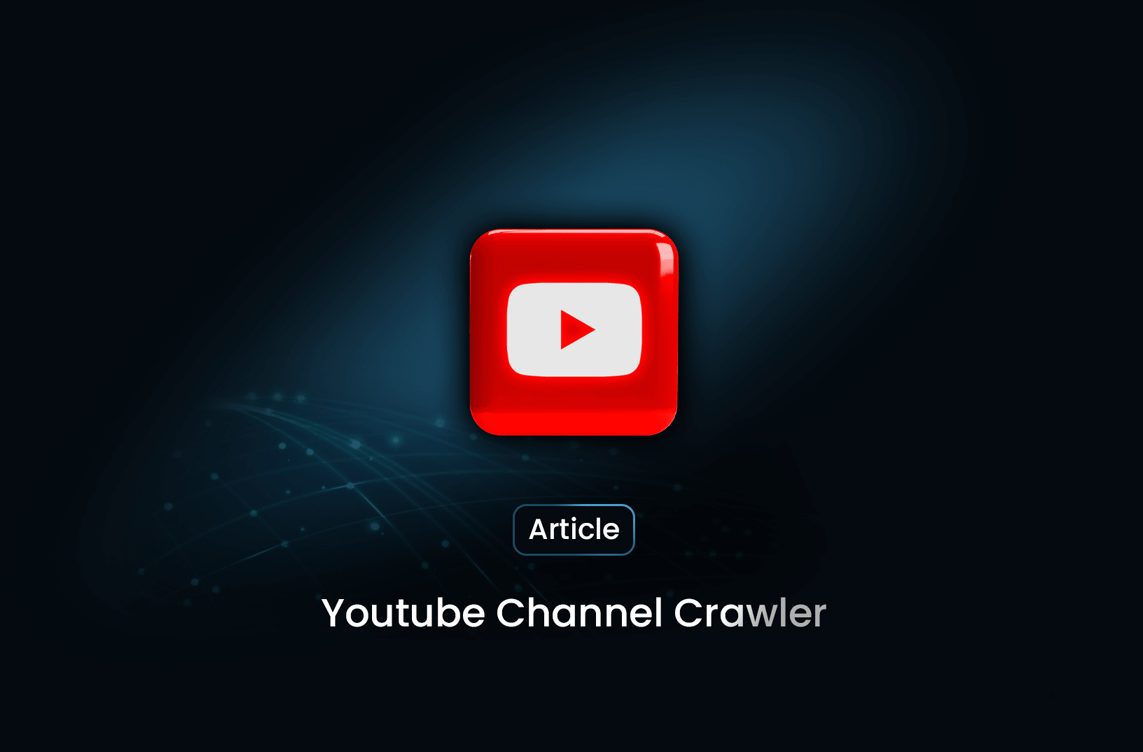
YouTube Channel Crawler
A YouTube channel crawler is a tool that automatically collects data from YouTube channels. It can extract information like video titles, descriptions, upload dates, views, likes, and comments, enabling efficient data analysis or research.
@MrScraper_
@MrScraper