What are Residential Proxies?
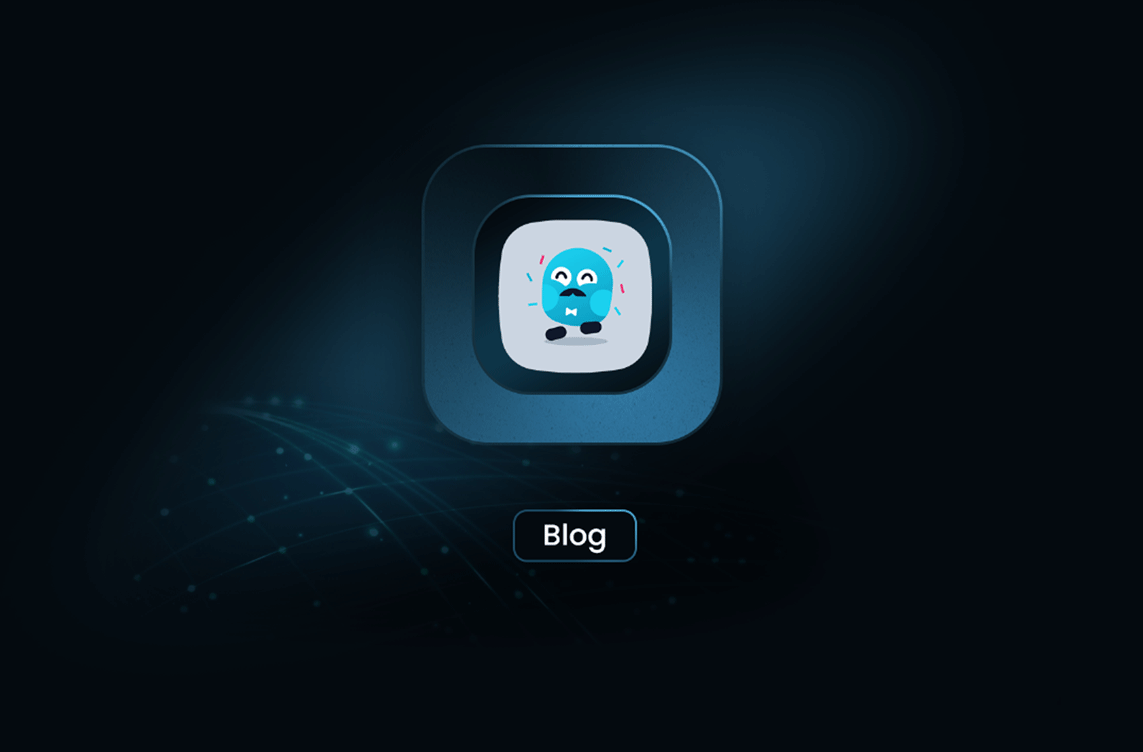
Scraping the web may be one of the most troublesome hurdles you face when you are scraping web pages as they might block you. This is because the website notices dubious activity, for example, repeating requests from the same IP address in a period of time shorter than expected. To counter this practice, developers are often to use residential proxies.
Residential proxies are Internet Service Provider (ISP) that offers a homeowner an IP addresses. These IPs are pegged as authentic because they are assigned to actual addresses, thus outsmarting the blocking attempts of site owners more than datacenter proxies.
Residential proxies are beneficial for web scraping because:
- Less likely to be blocked: Since residential proxies look like regular users’ IP addresses, websites are less likely to block them.
- Higher anonymity: It is harder for websites to detect and blacklist residential IPs.
- Geolocation flexibility: Residential proxies can be selected based on the region, helping you scrape content restricted by geography.
If you have a list of residential proxies and you want to use them manually in your scraping code, here’s how you can do it in JavaScript using a library like Puppeteer for browser automation:
const puppeteer = require('puppeteer');
// Proxy list example
const proxyList = [
'http://user:pass@proxy1.example.com:8000',
'http://user:pass@proxy2.example.com:8000',
'http://user:pass@proxy3.example.com:8000',
];
// Function to pick a random proxy from the residential proxies list
function getRandomProxy() {
return proxyList[Math.floor(Math.random() * proxyList.length)];
}
(async () => {
const proxy = getRandomProxy();
// Launch the browser with the selected proxy
const browser = await puppeteer.launch({
args: [`--proxy-server=${proxy}`], // Set the proxy for the browser instance
});
const page = await browser.newPage();
// Authenticate proxy if required
await page.authenticate({
username: 'user',
password: 'pass'
});
// Example: Go to a target website
await page.goto('https://example.com');
// Perform scraping actions
const content = await page.evaluate(() => document.body.innerText);
console.log(content);
await browser.close();
})();
In this code example:
- We define a list of residential proxies in the format
http://user:pass@proxy_address:port
. - The
getRandomProxy
function picks one proxy from the list at random. - We pass the selected proxy to Puppeteer’s
launch
function, ensuring that all requests go through the proxy. - If the proxy requires authentication, we use the
page.authenticate
function to pass the credentials.
Why Coding Web Scrapers Yourself Can Be Difficult
While using residential proxies can help avoid getting blocked, web scraping is still a complex task that involves several challenges:
- CAPTCHA Handling: Many websites use CAPTCHAs to prevent automated access. Solving these programmatically requires additional tools.
- Dynamic Content: Some websites load data dynamically using JavaScript frameworks like React or Angular, which means traditional scraping methods won’t work.
- Website Updates: Websites change their structure often, which means you need to frequently update your scraping code.
- Rate Limits: Even with proxies, scraping too quickly can result in your requests being throttled.
Rather than dealing with the complexity of coding, debugging, and maintaining your own web scrapers, why not use MrScraper? We provide an easy-to-use interface, handle proxy management, and adapt to changes on websites so you can focus on getting the data you need, quickly and efficiently.
Let us do the heavy lifting so you don’t have to!
Table of Contents
Take a Taste of Easy Scraping!
Get started now!
Step up your web scraping
Find more insights here
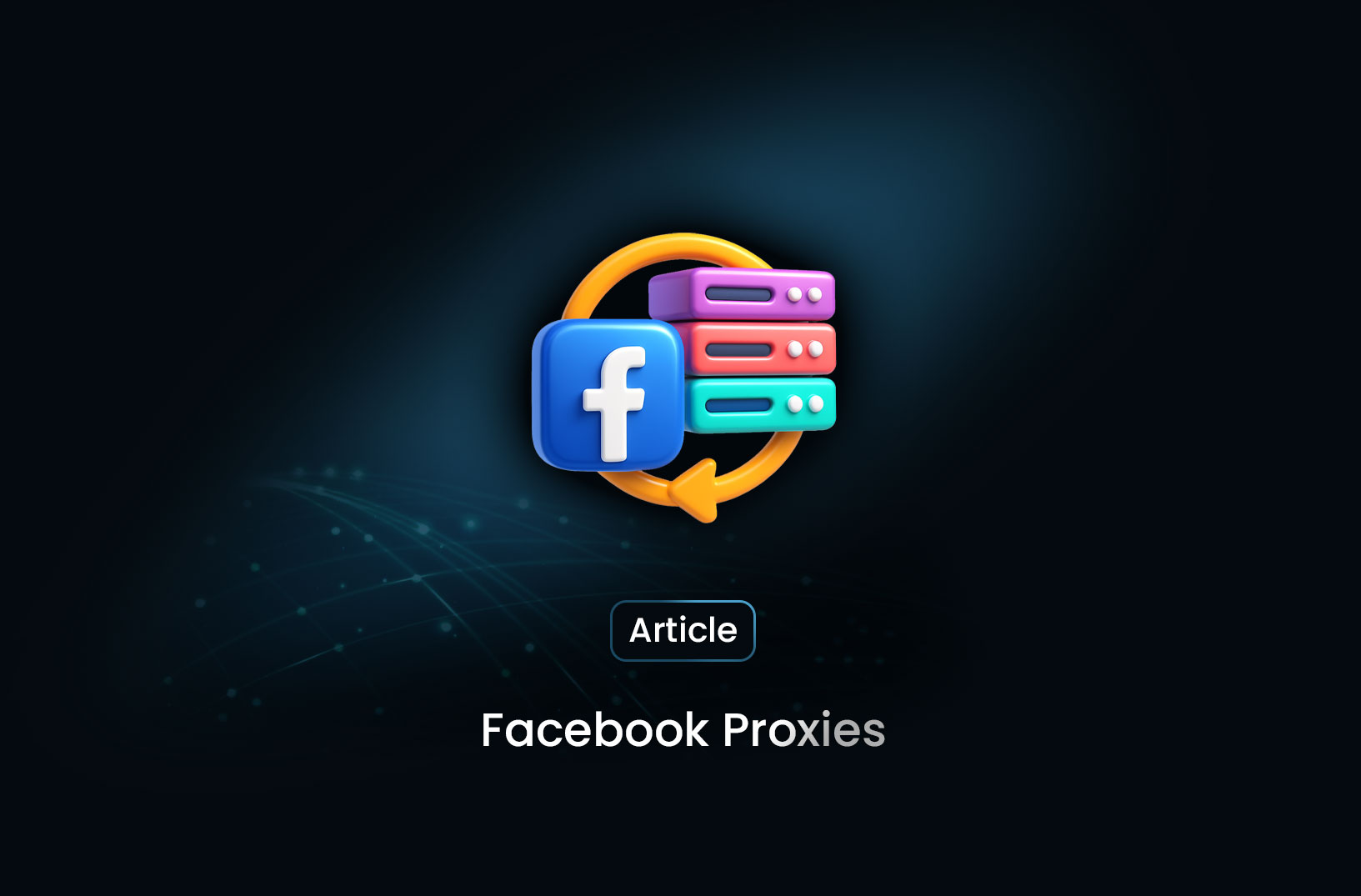
Best Facebook Proxies for Ads, Scraping, and Privacy in 2025
Facebook proxy routes your device’s Facebook traffic through an intermediary server. Instead of connecting directly, you use the proxy server to access Facebook, masking your real IP and location.
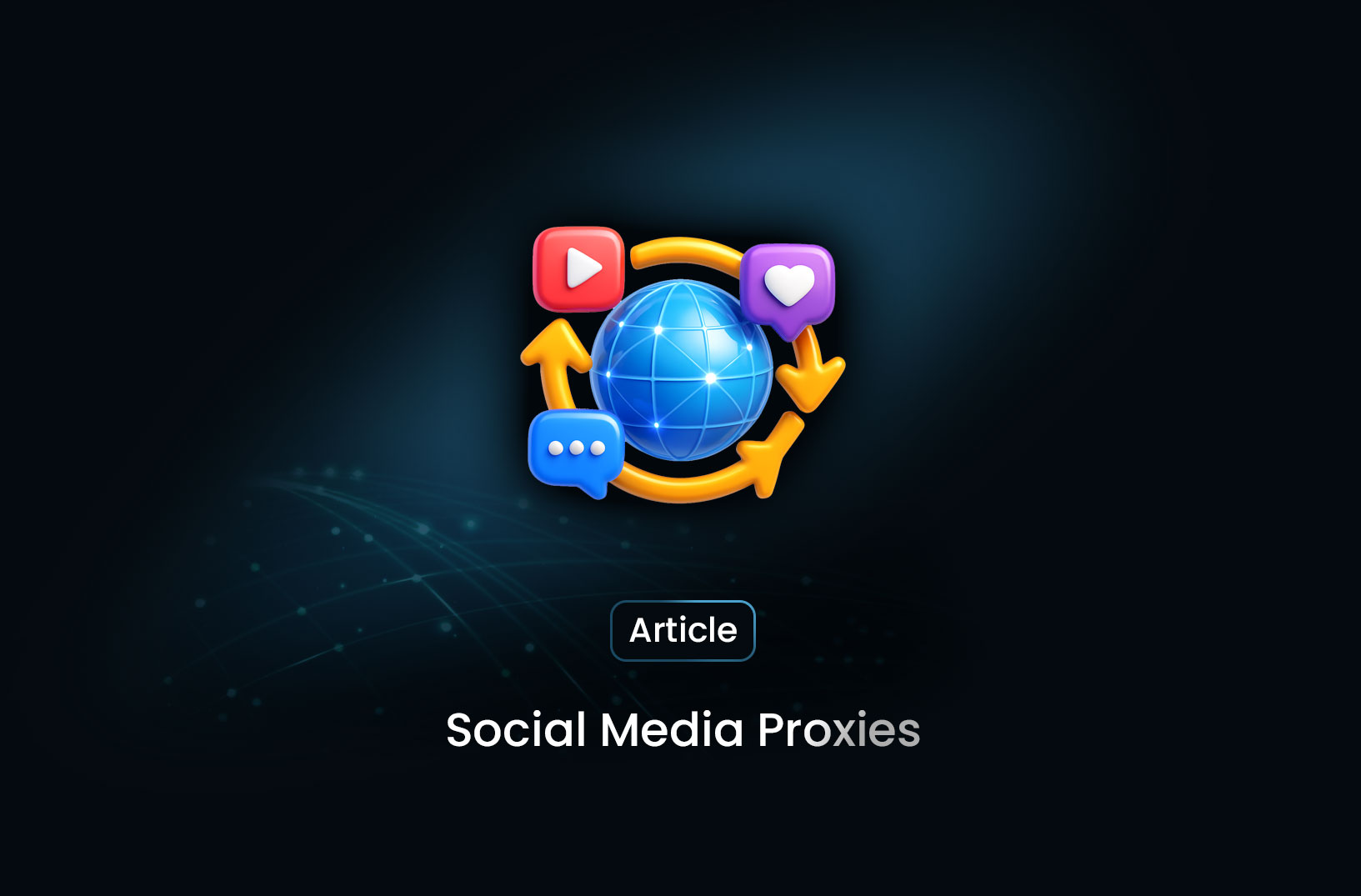
Social Media Proxies: Boost Privacy and Bypass Geo-Restrictions
Social media proxies are a cornerstone of modern online operations—be it account management, scraping, or ad campaigns.
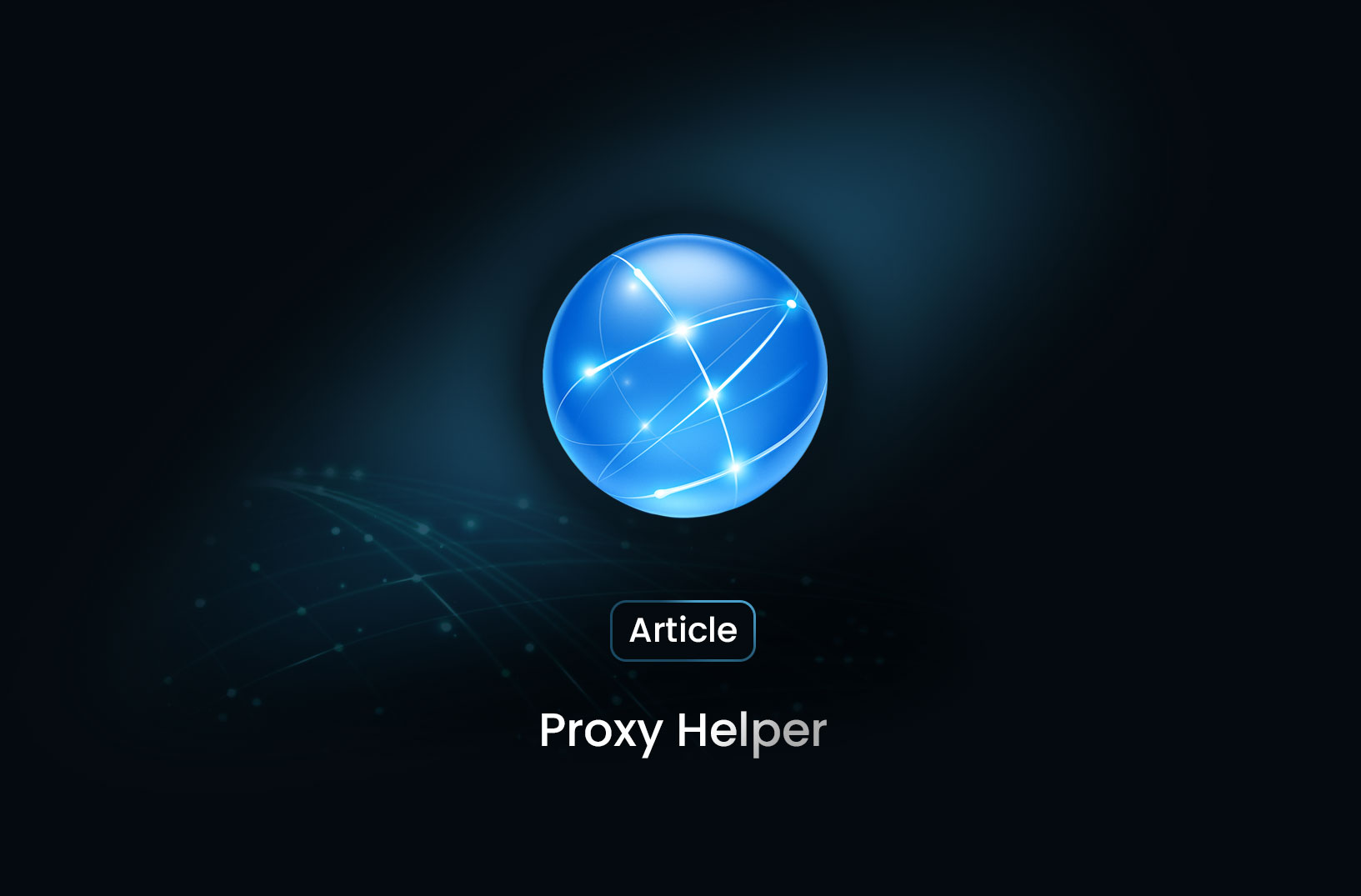
Proxy Helper: Your Chrome Extension for Browser-Level Proxy Control
Proxy Helper is a Chrome extension that allows users to configure and switch proxies directly within the browser, without touching system-wide settings.
@MrScraper_
@MrScraper