HTTP 429 Error: Too Many Requests
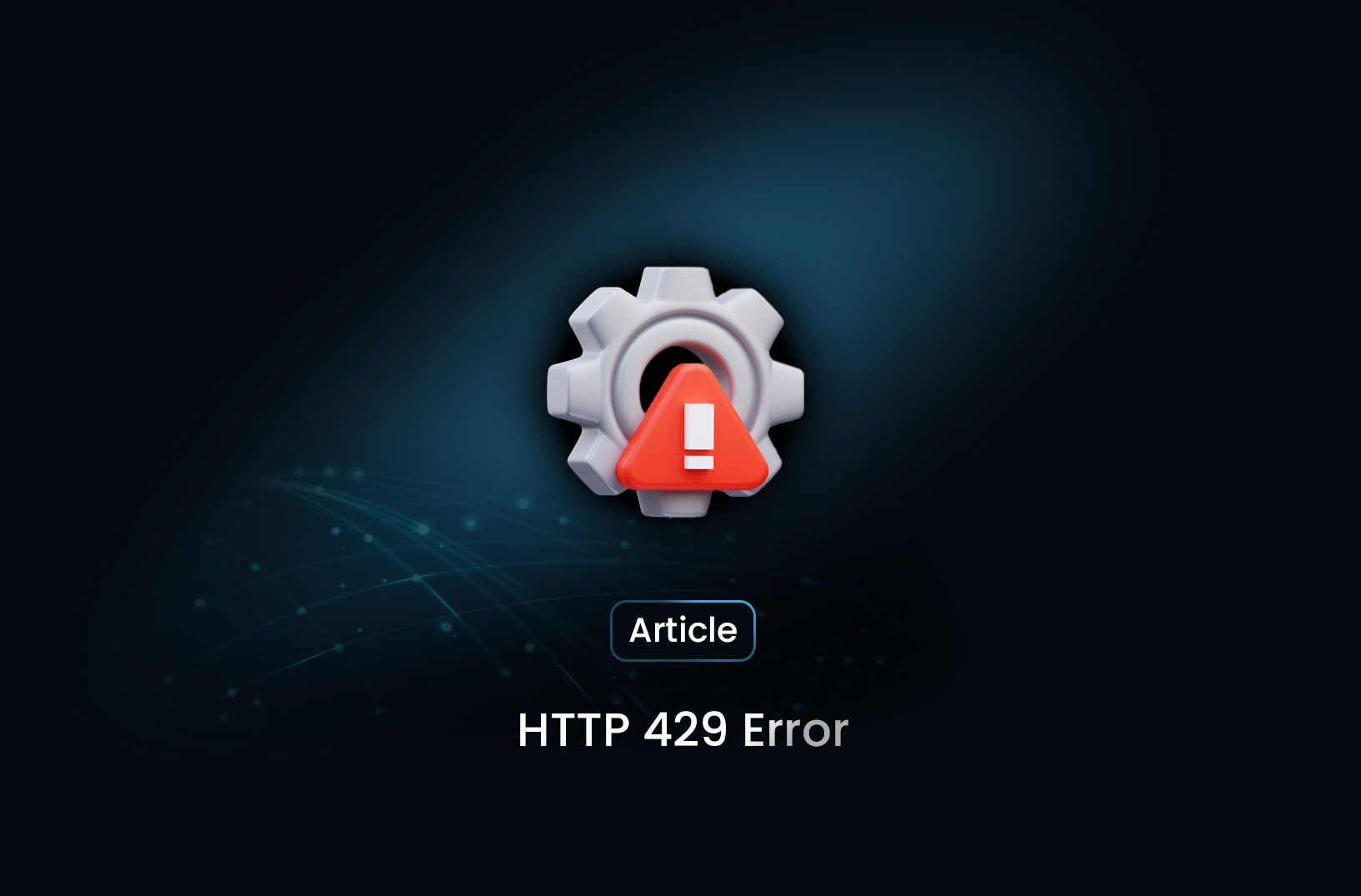
HTTP 429 is a status code that indicates the client has sent too many requests in a given timeframe. This is often triggered by rate-limiting mechanisms put in place by servers to prevent excessive or abusive traffic.
Common Causes of HTTP 429 Error
- API Rate Limits: Many APIs restrict the number of requests per minute/hour.
- Web Scraping Restrictions: Websites implement rate limits to prevent automated scraping.
- High Traffic Load: A sudden spike in user requests can trigger throttling.
- Bot Detection Mechanisms: Some servers identify excessive requests as bot activity.
- Repeated Login Attempts: Too many login attempts in a short period can cause a 429 error.
How to Handle HTTP 429 Error
1. Implement Exponential Backoff
Instead of retrying immediately, use an increasing delay between retries:
import time
import requests
def fetch_data_with_backoff(url, max_retries=5):
retries = 0
while retries < max_retries:
response = requests.get(url)
if response.status_code == 429:
wait_time = 2 ** retries # Exponential backoff
print(f"Rate limit hit. Retrying in {wait_time} seconds...")
time.sleep(wait_time)
retries += 1
else:
return response.json()
return None
2. Respect Rate Limits and Headers
Most APIs provide rate-limiting headers, such as:
- Retry-After: Suggests the wait time before retrying.
- X-RateLimit-Limit: Shows the allowed request limit.
- X-RateLimit-Remaining: Indicates remaining requests before hitting the limit.
Example of handling Retry-After
:
response = requests.get("https://api.example.com/data")
if response.status_code == 429:
retry_after = int(response.headers.get("Retry-After", 10)) # Default to 10s if missing
time.sleep(retry_after)
3. Use API Keys and Authenticated Requests
Some APIs allow higher rate limits for authenticated users.
headers = {"Authorization": "Bearer YOUR_ACCESS_TOKEN"}
requests.get("https://api.example.com/data", headers=headers)
4. Implement Request Throttling
Limit your request rate programmatically.
import time
def rate_limited_request(url, interval=1):
time.sleep(interval) # Wait before each request
return requests.get(url)
Use Cases for HTTP 429 Error Handling
1. API Clients and Integrations
Developers using APIs need to handle rate limits properly to avoid disruptions.
2. Web Scraping and Data Collection
Scrapers must implement delays and respect robots.txt
rules to avoid bans.
3. High-Traffic Applications
Web applications with sudden user surges should handle rate limits gracefully.
4. Security Measures Against Bots
Rate limiting is used to prevent brute-force attacks and credential stuffing.
Conclusion
Handling HTTP 429 errors properly ensures smooth operation of API clients, scrapers, and high-traffic applications. Implementing backoff strategies, respecting rate limits, and using authenticated requests can help mitigate issues related to request throttling.
Table of Contents
Take a Taste of Easy Scraping!
Get started now!
Step up your web scraping
Find more insights here
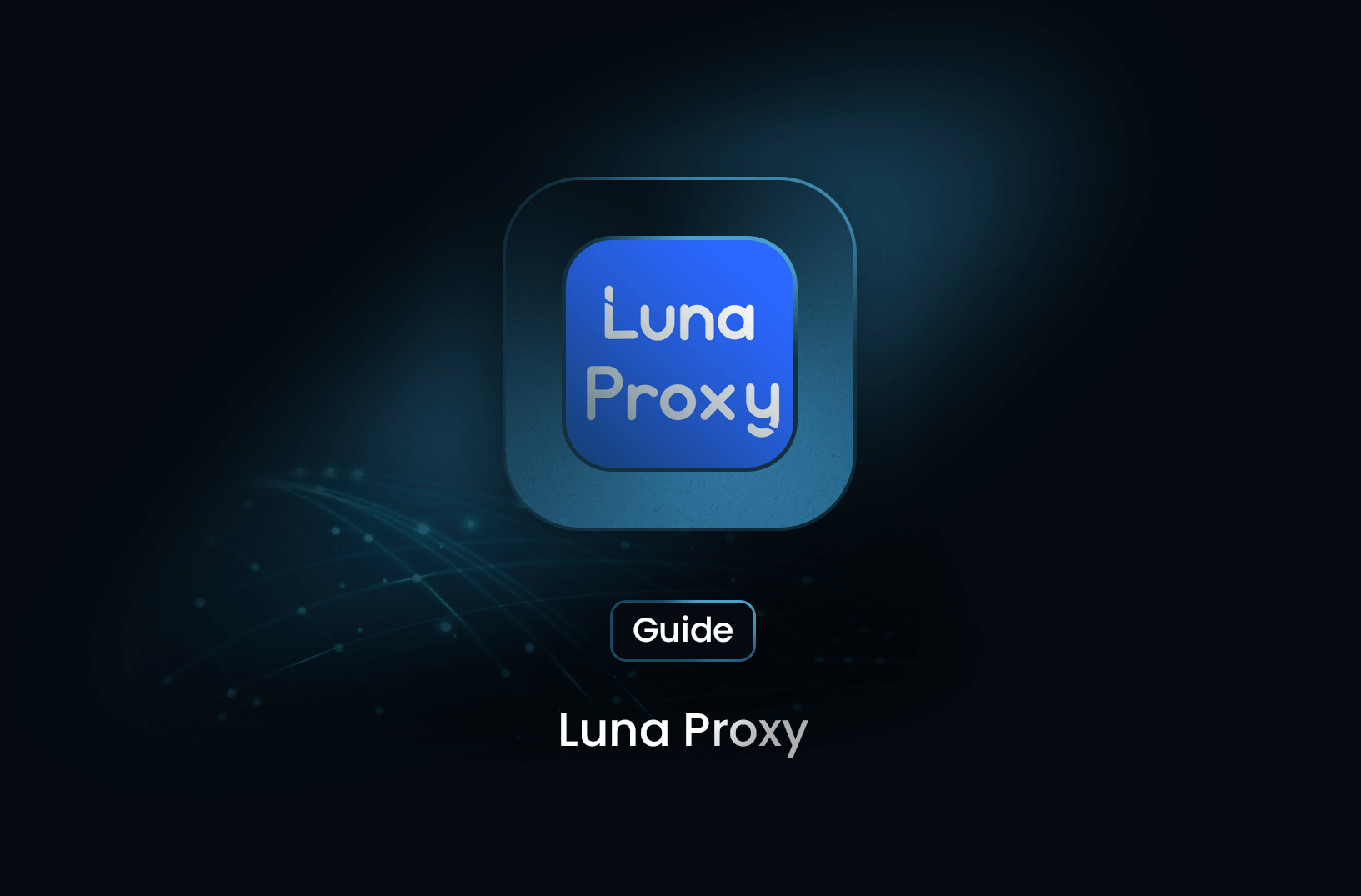
Unlocking a New Realm of Data Crawling: LunaProxy Helps Efficient Collection
Boost your data crawling efficiency with LunaProxy! Break through IP restrictions, enhance speed, and ensure high-quality data extraction with flexible proxy solutions.
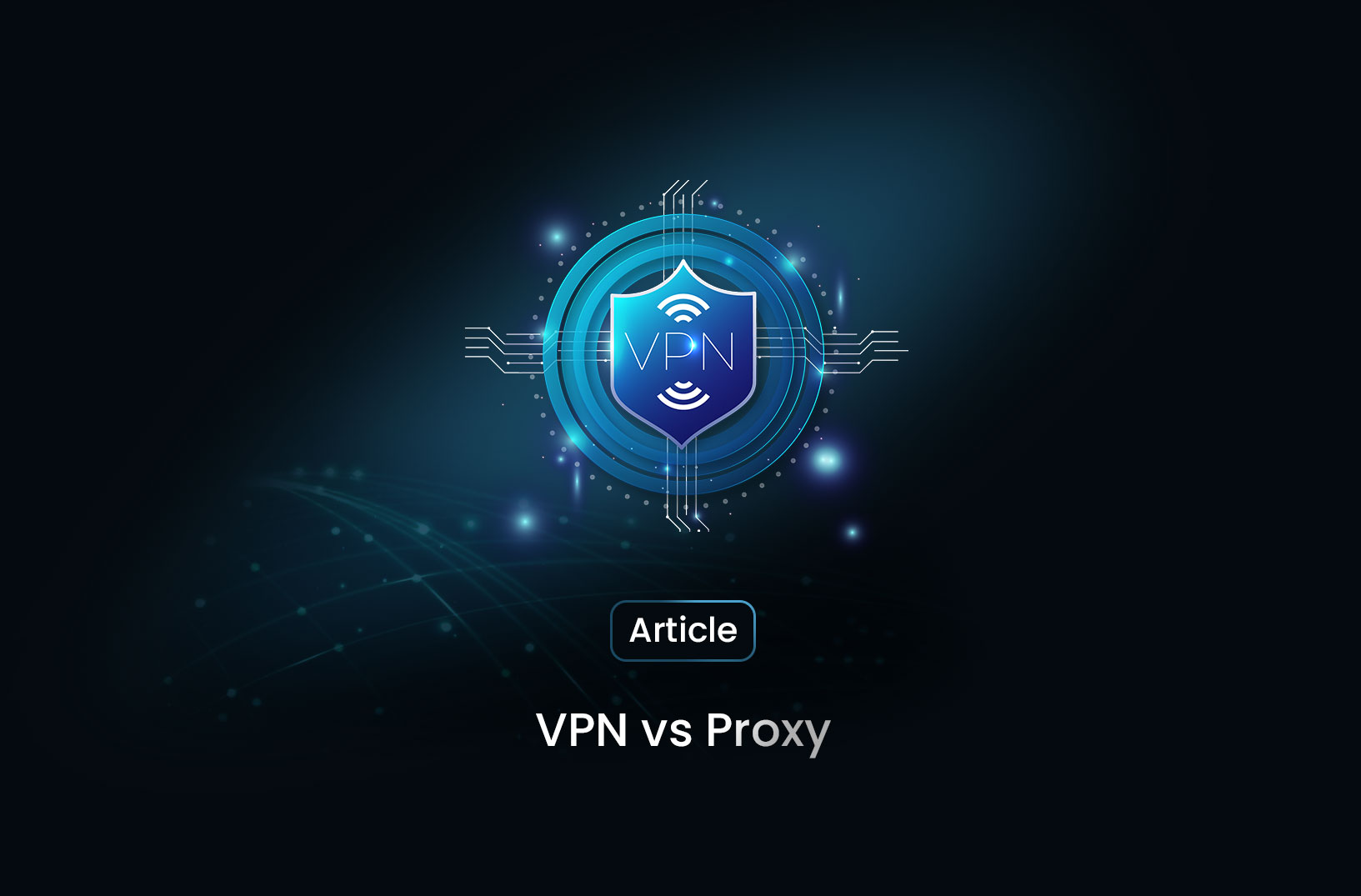
VPN vs Proxy: Which One Should You Use for Web Scraping?
VPN vs Proxy: Which one is better for web scraping? Learn the key differences between VPNs and proxies, their pros and cons, and which is best for data extraction.
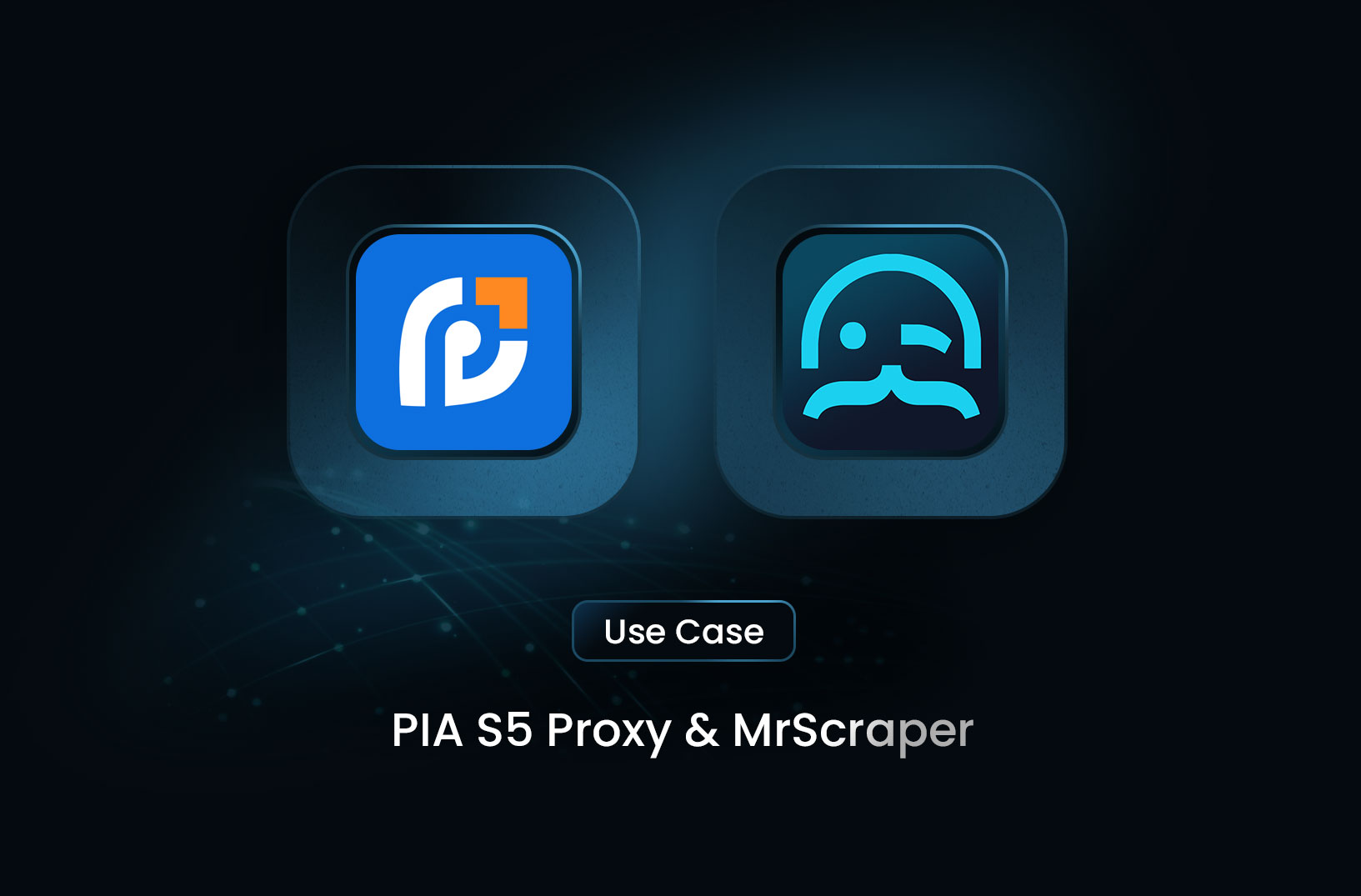
PIA S5 Proxy & MrScraper: A Powerful Scraping Combo
Discover how PIA S5 Proxy enhances data scraping with high-speed, secure, and geo-targeted proxy solutions. Avoid IP blocking, bypass restrictions, and improve scraping efficiency with real residential IPs. Learn how to integrate PIA S5 Proxy with Mrscraper for seamless data extraction.
@MrScraper_
@MrScraper