Python Requests Timeout
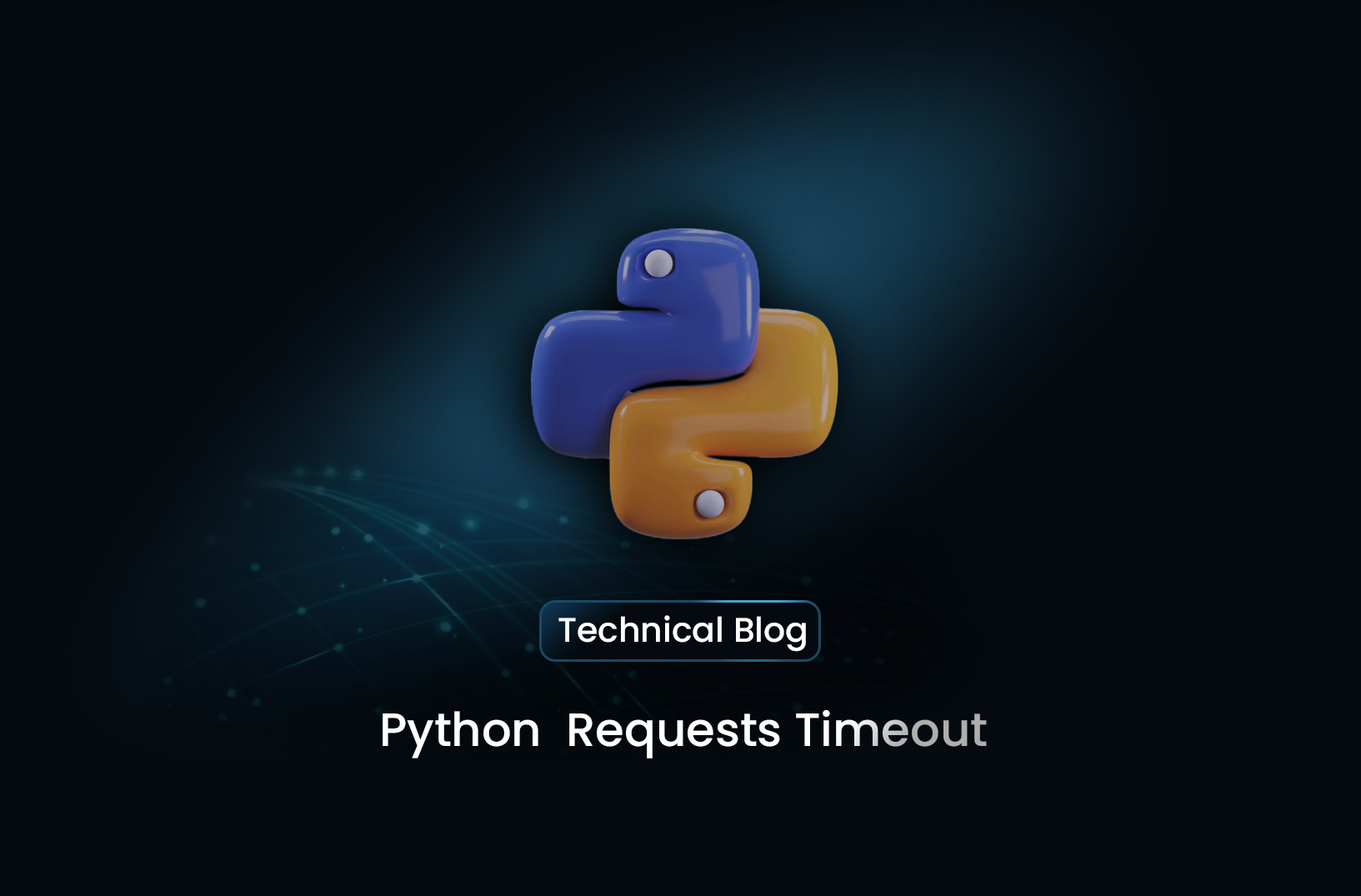
When working with network requests in Python, managing timeouts is essential for ensuring that your application remains responsive and doesn't hang indefinitely while waiting for a server’s response. The requests
library offers built-in functionality to set timeouts for network requests, which can help avoid delays and handle network latency more effectively.
Introduction to Timeout in Python Requests
What is a Timeout?
A timeout specifies the maximum time that a request will wait for a server to respond. If the server does not respond within the specified time, a timeout error is raised.
Why Timeouts are Important
- This prevents the application from hanging indefinitely.
- Helps manage network issues by retrying or terminating requests that exceed the set time limit.
- Ensures better user experience by reducing waiting times.
Basic Usage of Timeouts in Python Requests
The timeout
parameter can be passed in the requests.get()
or requests.post()
methods to limit the time spent waiting for a response.
import requests
try:
response = requests.get("https://example.com", timeout=5)
response.raise_for_status()
print(response.text)
except requests.exceptions.Timeout:
print("The request timed out")
except requests.exceptions.RequestException as e:
print(f"An error occurred: {e}")
Explanation
timeout=5:
The request will wait for up to 5 seconds for the server to respond.requests.exceptions.Timeout
: Catches timeout-specific errors.requests.exceptions.RequestException
: Catches all other exceptions related to the request.
Types of Timeout Parameters
You can specify different timeouts for different stages of the request:
- Connect Timeout: The time to establish a connection to the server.
- Read Timeout: The time to wait for the server to send a response.
Example:
response = requests.get("https://example.com", timeout=(3, 10))
In this case, the request will wait up to 3 seconds to establish a connection and 10 seconds to receive a response from the server.
Best Practices for Setting Timeout Values
- Consider Application Requirements:
- Real-time applications (e.g., trading, live data): Short timeouts are ideal to maintain quick response times.
- Data scraping: Longer timeouts may be necessary when fetching large amounts of data or when dealing with slow servers.
- Use Default Short Timeouts: A timeout of 5 seconds is a common starting point. You can adjust it depending on the needs of your application.
- Retry on Timeout: Implement retries for requests that time out, especially if the issue is intermittent.
Handling Timeout Exceptions Gracefully
Handling timeouts gracefully improves user experience by providing feedback or retry mechanisms.
Example of Handling Timeouts and Retrying Requests
from requests.adapters import HTTPAdapter
from requests.packages.urllib3.util.retry import Retry
session = requests.Session()
retries = Retry(total=3, backoff_factor=1, status_forcelist=[500, 502, 503, 504])
session.mount("https://", HTTPAdapter(max_retries=retries))
try:
response = session.get("https://example.com", timeout=5)
print(response.text)
except requests.exceptions.Timeout:
print("The request timed out after multiple attempts")
Explanation:
- Retry Strategy: Retries the request up to 3 times in case of server errors (status codes 500, 502, 503, 504).
- Backoff Factor: Increases the delay between retries, making retries less aggressive.
Common Timeout Errors and Solutions
- Timeout Error: Caused by server delays or unresponsiveness. Increase the timeout value if the server is known to take longer to respond.
- Connection Timeout: Happens when the client cannot establish a connection to the server within the specified time. Check the server's availability or increase the connect timeout.
- Read Timeout: Occurs when the server is taking too long to respond. Increase the read timeout or investigate the server's performance.
Use Cases for Python Requests Timeout
Timeouts are useful in various scenarios:
- Web Scraping: Helps prevent long delays when scraping multiple pages.
- API Integrations: Ensures that your application doesn’t hang when the API is unresponsive.
- Real-Time Data Processing: Critical for time-sensitive applications like trading systems or monitoring dashboards.
Conclusion
Properly handling timeouts is essential for building stable and responsive applications. By using the timeout
parameter, you can prevent requests from hanging indefinitely, reducing the risk of wasted resources and improving the overall user experience. You should tailor your timeout settings based on your application’s needs—real-time applications require faster timeouts, while data scraping may need more generous time limits.
Additionally, implementing error handling for timeouts and using retries ensures your application can gracefully handle network issues and keep running smoothly. Testing different timeout configurations will help you find the optimal balance for your specific use case, whether you're scraping data, calling APIs, or processing real-time information.
Table of Contents
Take a Taste of Easy Scraping!
Get started now!
Step up your web scraping
Find more insights here
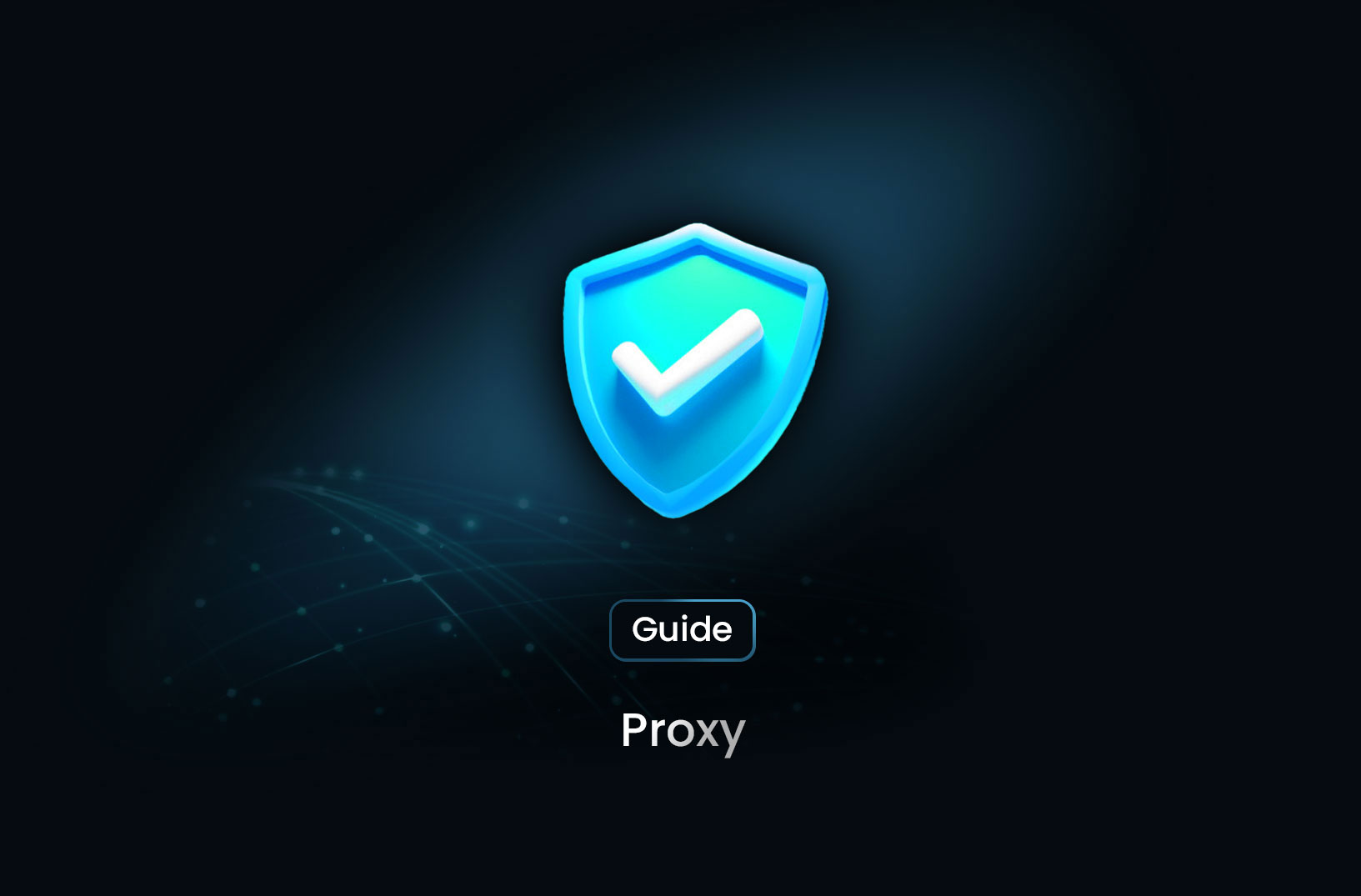
A Beginner’s Guide to Proxy Servers
A proxy acts as an intermediary—a substitute—between you and something else. In technology, this typically refers to a proxy server, which handles data on your behalf. Here's a detailed look at what proxies are, how they work, and why they're essential.
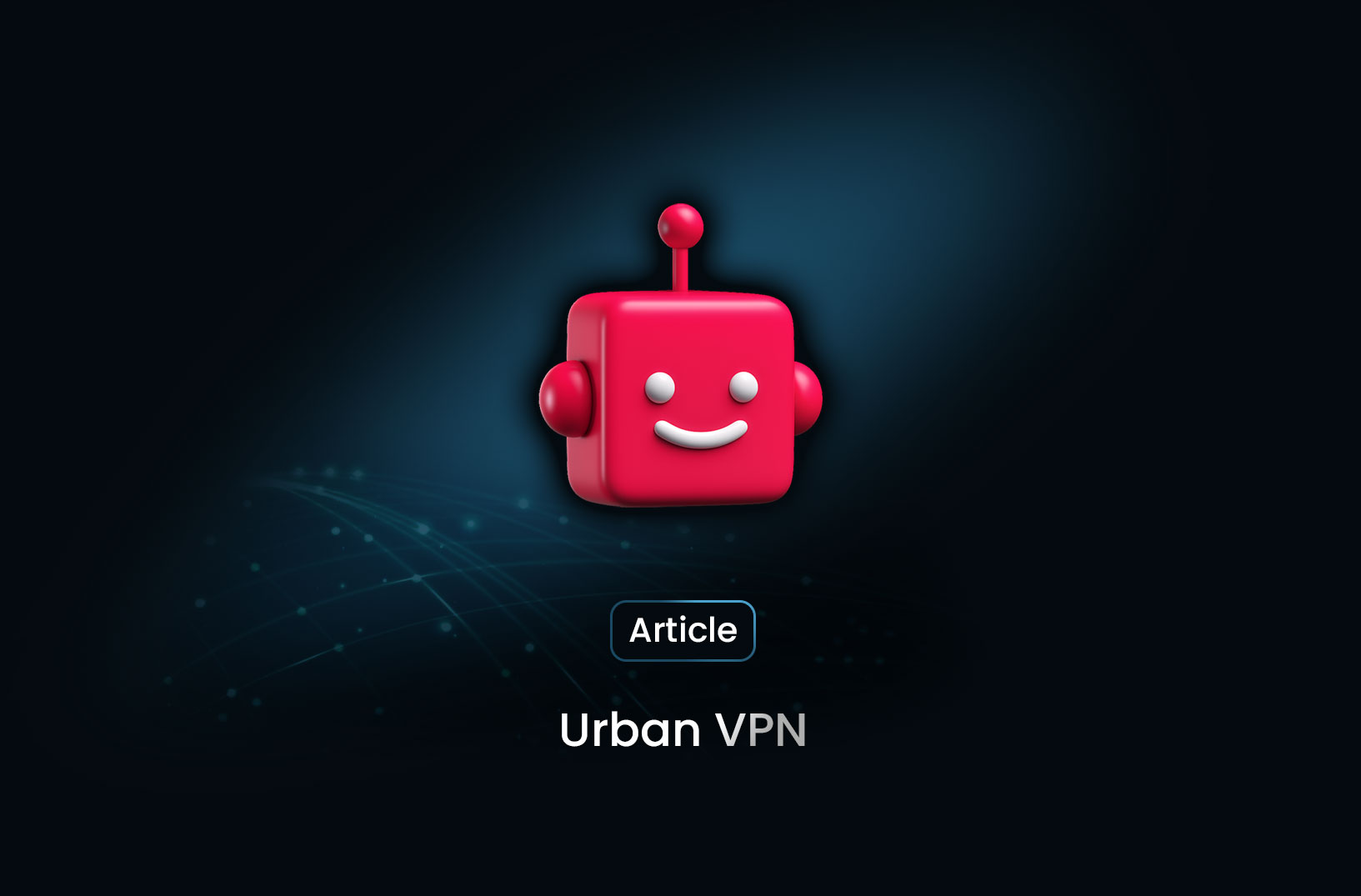
Urban VPN: A Free VPN Worth Using?
Urban VPN is a 100% free VPN with global server options and unlimited bandwidth—but its usability comes with significant trade-offs.
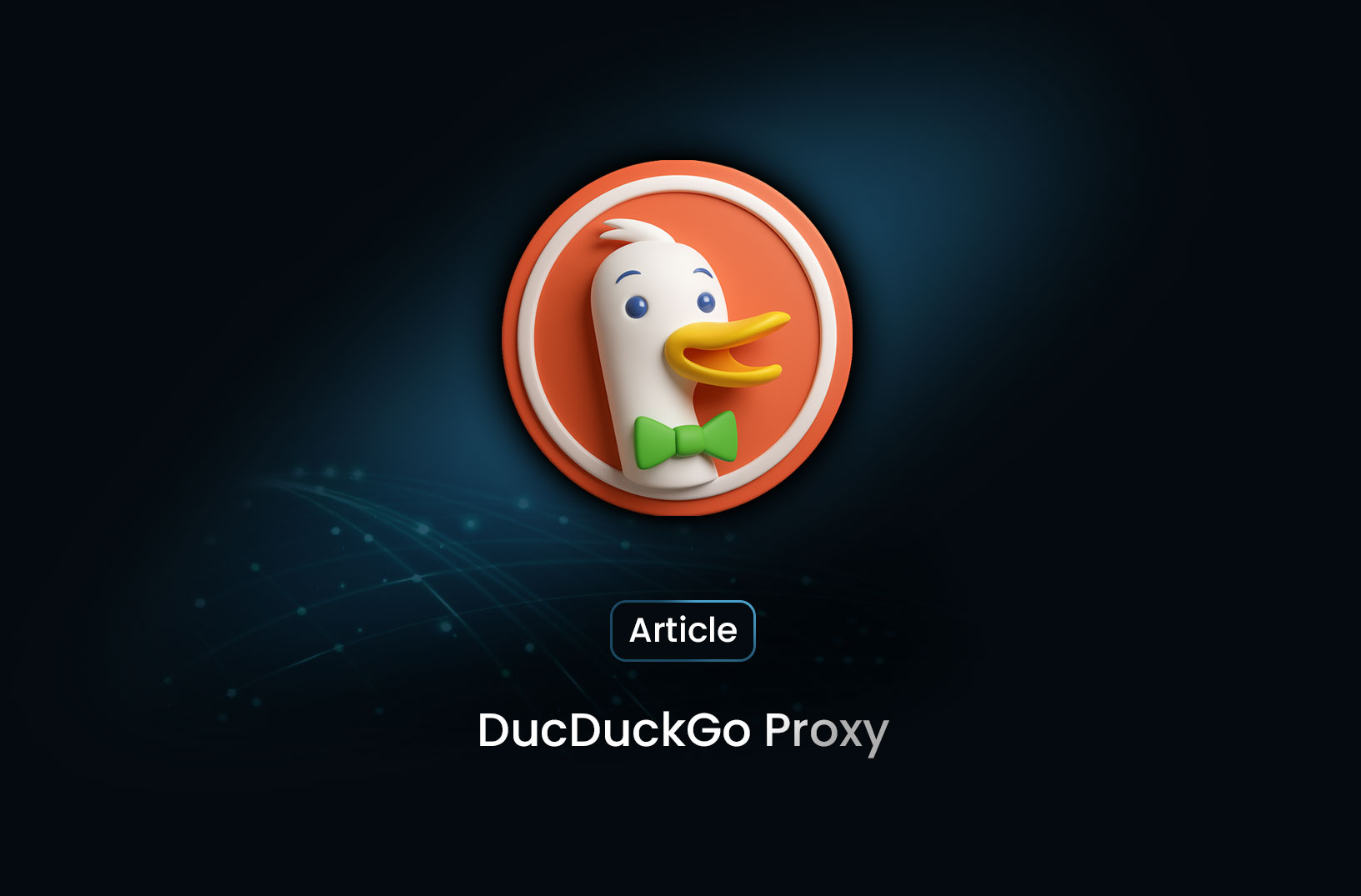
DuckDuckGo Proxy: Browse Anonymously Without a VPN
DuckDuckGo isn’t just a privacy-focused search engine—it also offers a built-in web proxy that allows users to browse sites anonymously via its servers.
@MrScraper_
@MrScraper