Parsing Data: A Technical Guide for Developers
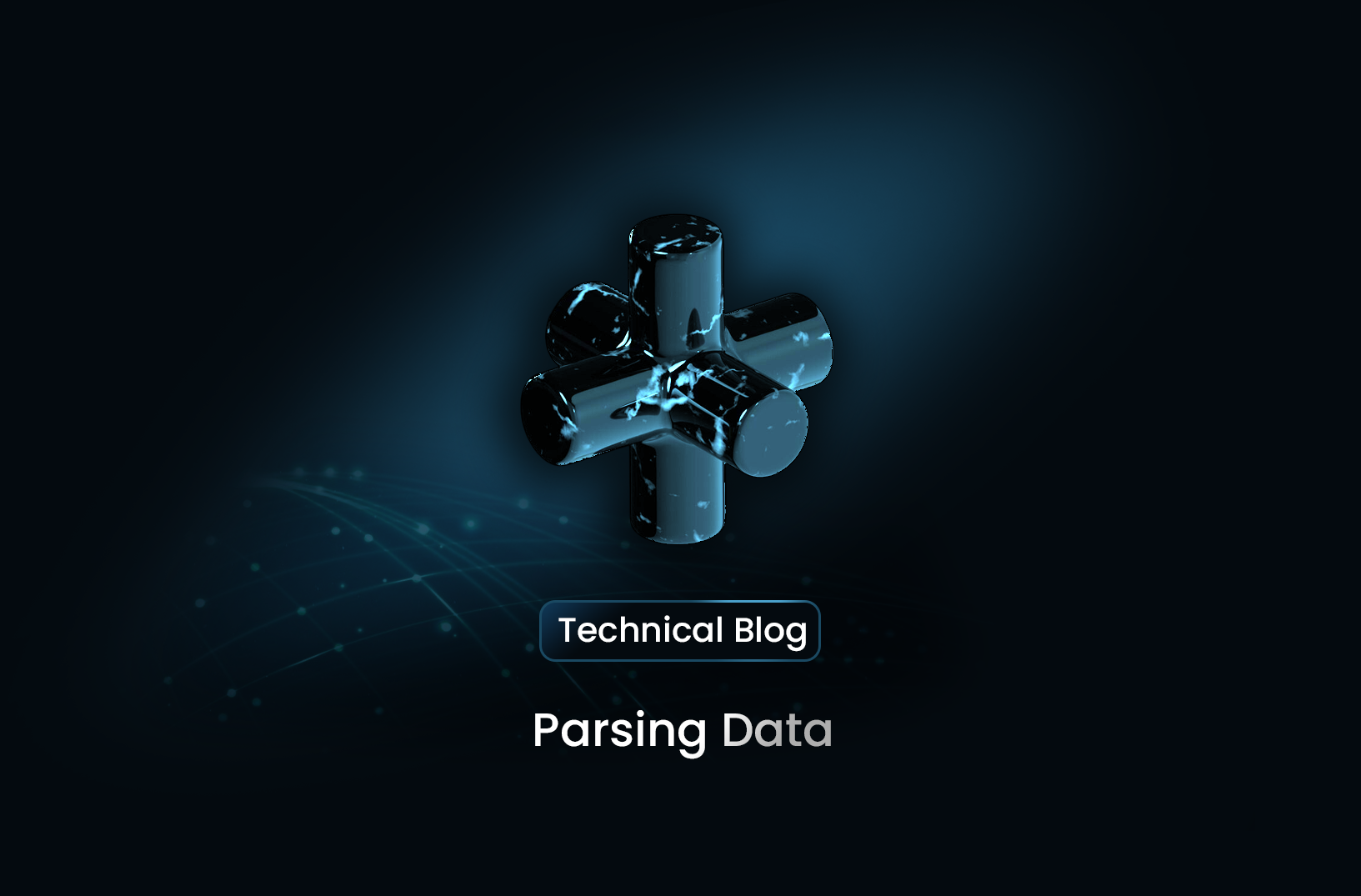
Data parsing is a crucial process in software development, enabling programs to analyze and manipulate data from various sources. This guide delves into the technical aspects of parsing data, covering common methods, data formats, and practical examples in Python. Whether you’re parsing JSON, XML, or text data, understanding parsing techniques will enhance your data-handling capabilities.
What is Data Parsing?
Data parsing is the process of breaking down structured data into more manageable components for processing. This involves analyzing and extracting key information, then transforming it into a desired format. Parsing is commonly applied to structured data formats, like JSON and XML, but also to plain text.
Why Parsing is Important
Parsing is foundational in tasks like web scraping, API response handling, and file manipulation. Effective parsing allows developers to:
- Extract relevant information quickly
- Structure data for analysis or storage
- Interact with external data sources through APIs
Common Data Formats for Parsing
- JSON (JavaScript Object Notation)
- Lightweight and commonly used in APIs and data interchange.
- Easy to parse in most programming languages.
- XML (Extensible Markup Language)
- Heavily used in data transmission, especially in older APIs.
- Structured in a tree-like format.
- CSV (Comma-Separated Values)
- Often used for tabular data.
- Simple and lightweight but lacks complex structure.
- HTML
- Web page format that often requires parsing for scraping and data extraction.
- Plain Text
- Raw text data, which may require custom parsing logic.
Parsing Techniques
- Regex (Regular Expressions)
- Useful for extracting specific patterns in text data.
- Suitable for custom formats but may become complex for nested structures.
- Library-based Parsing
- Many libraries exist for parsing standard formats (e.g.,
json
andxml.etree.ElementTree
in Python). - Offers built-in functionality for efficient parsing.
- Custom Parsers
- Write your parsers if dealing with highly specific formats or unstructured data.
Examples of Parsing Data in Python
- Parsing JSON Data
The json
module in Python is used to parse JSON data easily. Here’s how to parse JSON from an API response:
import json
# Sample JSON string
json_data = '{"name": "John", "age": 30, "city": "New York"}'
# Parse JSON into a dictionary
data = json.loads(json_data)
print(data['name']) # Output: John
For reading JSON from a file:
with open('data.json') as f:
data = json.load(f)
print(data)
- Parsing XML Data
XML data parsing is done with the xml.etree.ElementTree
module:
import xml.etree.ElementTree as ET
# Sample XML data
xml_data = '''<person><name>John</name><age>30</age><city>New York</city></person>'''
# Parse the XML data
root = ET.fromstring(xml_data)
# Extract values
name = root.find('name').text
print(name) # Output: John
- Parsing CSV Data
The csv
module is commonly used to parse CSV data:
import csv
# Open and parse a CSV file
with open('data.csv', newline='') as csvfile:
reader = csv.reader(csvfile)
for row in reader:
print(row)
Using csv.DictReader
to work with CSV data as dictionaries:
with open('data.csv', newline='') as csvfile:
reader = csv.DictReader(csvfile)
for row in reader:
print(row['name'], row['age'])
- Parsing HTML Data with BeautifulSoup
For web scraping, BeautifulSoup
is a powerful library:
from bs4 import BeautifulSoup
import requests
# Fetch webpage content
response = requests.get('https://example.com')
soup = BeautifulSoup(response.text, 'html.parser')
# Parse and extract data
title = soup.title.string
print(title)
- Parsing Text with Regex
For custom text formats, regular expressions offer flexibility:
import re
# Sample text
text = "Contact: John Doe, Phone: 123-456-7890"
# Regex pattern for phone numbers
pattern = r'\d{3}-\d{3}-\d{4}'
match = re.search(pattern, text)
if match:
print(match.group()) # Output: 123-456-7890
Best Practices for Data Parsing
-
Choose the Right Parsing Method: Use libraries for common formats, and only use custom parsing for unique data.
-
Validate Parsed Data: Always validate data after parsing to catch errors early.
-
Handle Errors Gracefully: Implement error handling to manage unexpected data issues or format changes.
-
Optimize for Efficiency: Parsing large files can be slow; consider using iterative parsing techniques (e.g.,
json.load()
for large JSON files). -
Document Parsing Logic: If using complex or custom parsing logic, document it for easier maintenance.
Conclusion
Data parsing is a vital skill in data science, web development, and automation. By understanding the various parsing techniques and using the right libraries, you can effectively manage and manipulate data from multiple sources.
Table of Contents
Take a Taste of Easy Scraping!
Get started now!
Step up your web scraping
Find more insights here
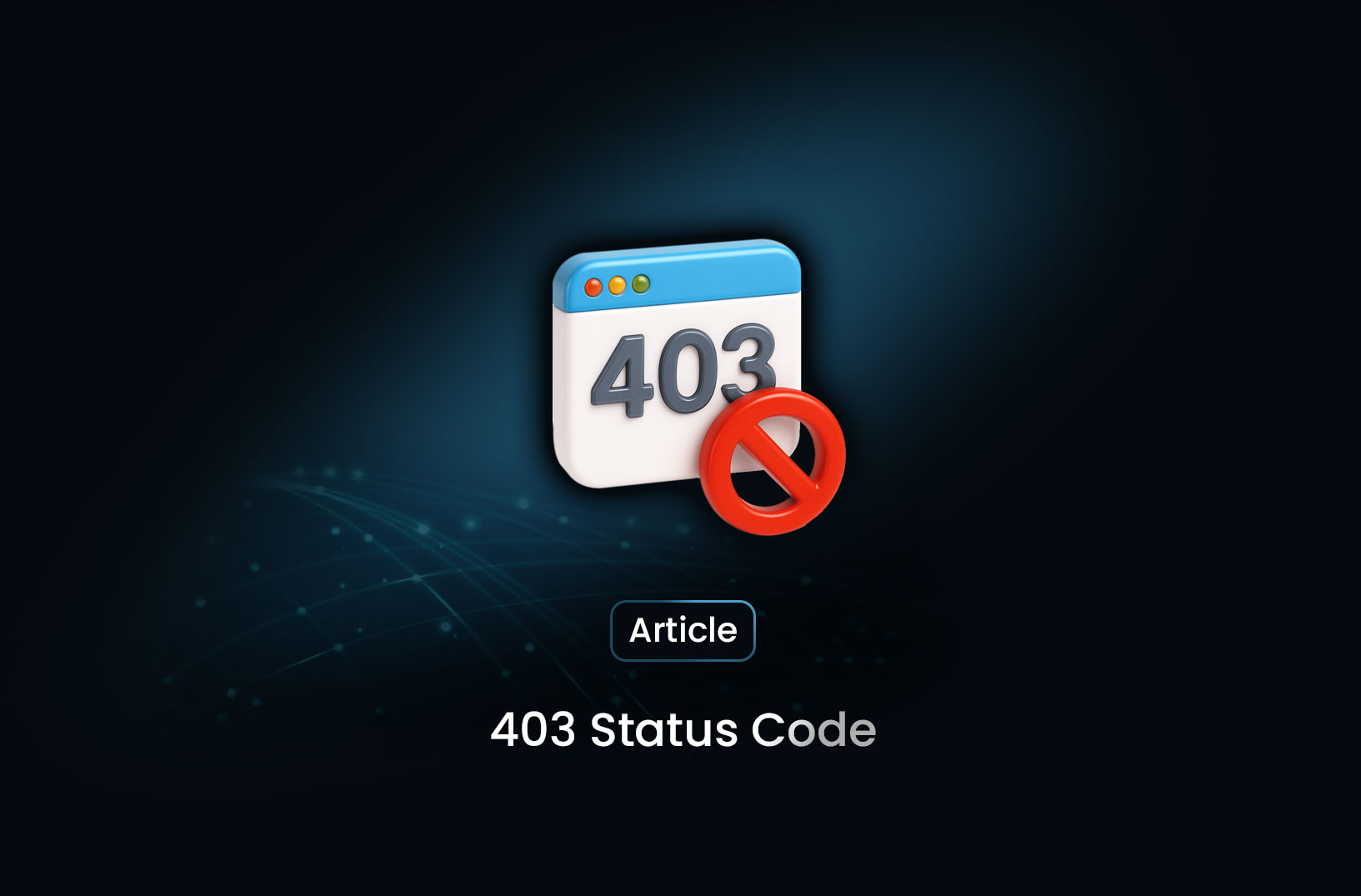
403 Status Code: Meaning, Causes, and Solutions
403 Forbidden status means the server received a valid request but refuses to process it due to insufficient permissions.
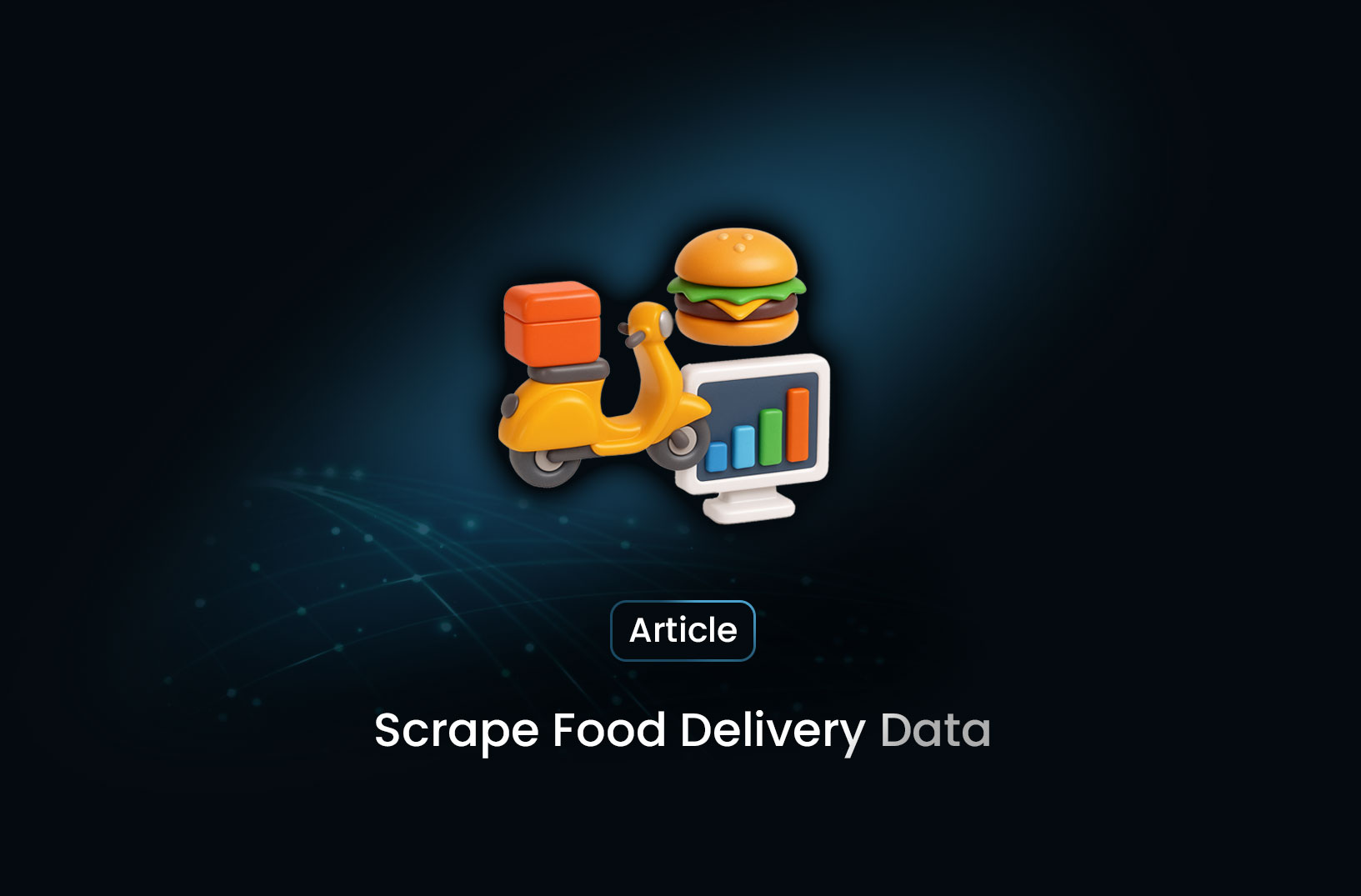
How to Scrape Food Delivery Data for Competitive Intelligence
Learn how to scrape food delivery data from platforms like ShopeeFood, GrabFood, and GoFood to uncover pricing trends, customer reviews, and market insights. Discover the tools, challenges, and how MrScraper can simplify the process.
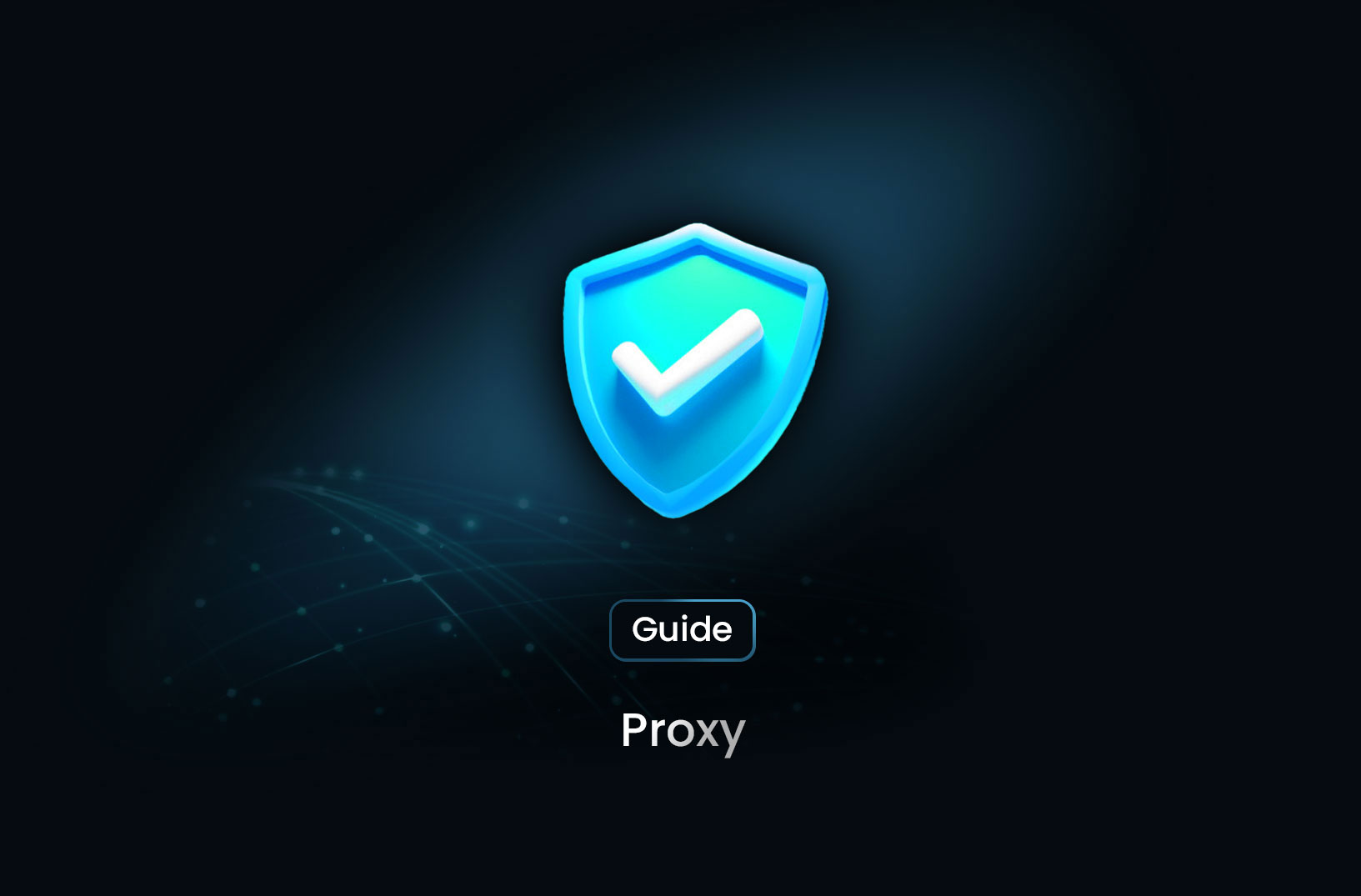
A Beginner’s Guide to Proxy Servers
A proxy acts as an intermediary—a substitute—between you and something else. In technology, this typically refers to a proxy server, which handles data on your behalf. Here's a detailed look at what proxies are, how they work, and why they're essential.
@MrScraper_
@MrScraper