How to Parse JSON in Python
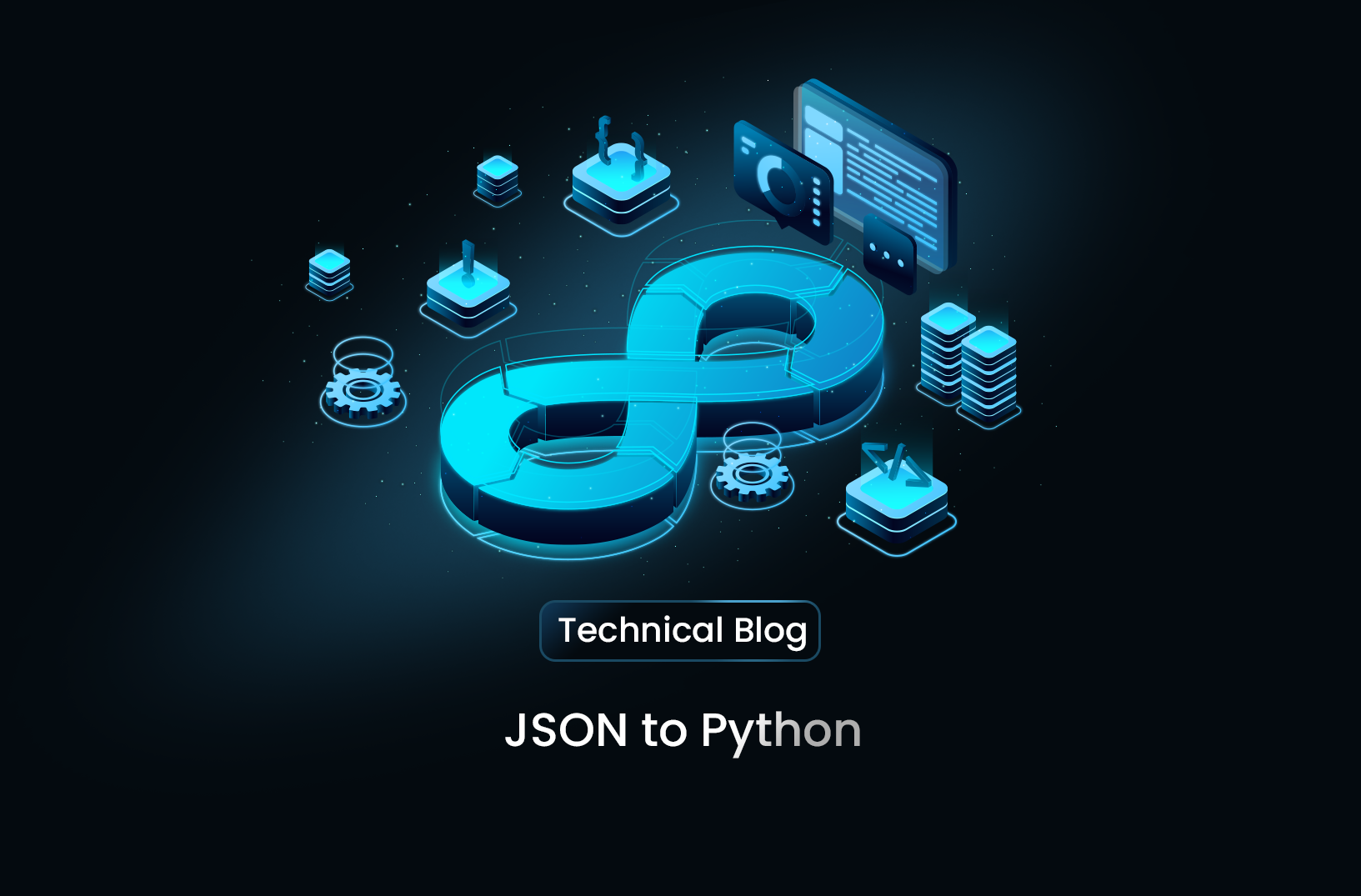
What is JSON?
JSON (JavaScript Object Notation) is a lightweight data interchange format that is easy for humans to read and write, and easy for machines to parse and generate. It is commonly used for transmitting data in web applications between servers and clients due to its simplicity and flexibility.
JSON is built on two structures:
- Key-Value Pairs (Objects): Enclosed in curly braces {}, where the keys are strings and the values can be strings, numbers, arrays, or other objects.
- Arrays (Lists): An ordered collection of values enclosed in square brackets [].
Example of a JSON Object
Here’s an example of a simple JSON object representing a user:
{
"name": "Alice",
"age": 30,
"city": "Wonderland",
"is_verified": true,
"favorites": {
"color": "blue",
"food": "pizza"
},
"phones": ["123-4567", "987-6543"]
}
In this JSON:
"name"
,"age"
, and"city”
are key-value pairs."favorites"
is an object containing two key-value pairs."phones"
is an array (or list) of phone numbers. Now that you have an understanding of JSON, let's explore how to parse it in Python using thejson
module.
Parsing JSON in Python with json Module
Python’s json
module provides built-in support to handle JSON data. The key methods are:
json.loads()
: Parses a JSON string into a Python dictionary.json.load()
: Parses JSON data directly from a file.json.dumps()
: Converts a Python object into a JSON string.
1. Parsing a JSON String
The json.loads()
function is used to convert a JSON string into a Python dictionary:
import json
#Sample JSON string
json_data = '{"name": "Alice", "age": 30, "city": "Wonderland"}'
#Parse JSON string to Python dictionary
data = json.loads(json_data)
#Accessing values
print(data['name']) # Output: Alice
In this example, the JSON string is parsed into a Python dictionary, and we can access values like any regular dictionary.
2. Parsing JSON from a File
The json.load()
function can be used to read and parse JSON directly from a file. This is useful for working with larger datasets stored in JSON format.
import json
#Open JSON file
with open('data.json') as file:
data = json.load(file)
#Accessing values
print(data['city']) # Example: Output might be 'Wonderland'
This example assumes there’s a file data.json containing JSON data, which gets loaded into a Python dictionary.
Handling Nested JSON Data
Sometimes, JSON data can have more complex structures like nested dictionaries and lists. Let’s look at how to handle this.
import json
#Nested JSON string
json_data = '''
{
"name": "Alice",
"age": 30,
"address": {
"street": "123 Main St",
"city": "Wonderland"
},
"phones": ["123-4567", "987-6543"]
}
'''
#Parse the JSON string
data = json.loads(json_data)
#Access nested data
print(data['address']['city']) # Output: Wonderland
print(data['phones'][0]) # Output: 123-4567
In this example, we navigate through the nested structure to access values like "city" and the first phone number.
Parsing JSON from APIs
When working with APIs, JSON is often the format of choice for transmitting data. Using the requests
library, you can fetch JSON data from an API and parse it in Python.
import json
import requests
#API URL
url = "https://api.example.com/data"
#Fetch data from API
response = requests.get(url)
#Parse JSON from API response
data = response.json()
#Accessing values
print(data['result'])
In this example, the API response is automatically parsed into a Python dictionary using response.json()
.
Error Handling with JSON Parsing
When parsing JSON, errors may occur if the data is malformed or there are issues with reading the file. Python raises a JSONDecodeError
in these cases.
import json
#Invalid JSON string (notice the trailing comma)
json_data = '{"name": "Alice", "age": 30,}'
try:
data = json.loads(json_data)
except json.JSONDecodeError as e:
print(f"Error parsing JSON: {e}")
This will catch and handle any parsing errors, helping you debug invalid JSON data.
Converting Python Objects to JSON
You can also use json.dumps()
to convert Python objects (like dictionaries) into JSON strings, which can then be saved to a file or transmitted via HTTP.
import json
#Python dictionary
data = {
"name": "Alice",
"age": 30,
"city": "Wonderland"
}
#Convert dictionary to JSON string
json_string = json.dumps(data)
print(json_string)
This method is useful when you need to serialize Python objects to JSON for storage or communication.
Conclusion
Python's json
module provides a simple and effective way to parse and manipulate JSON data. Whether you’re retrieving data from an API, working with nested structures, or converting Python objects to JSON for storage or transmission, Python makes it easy to handle JSON in a flexible and robust way.
If you're looking to scrape data from websites without the hassle of complex coding, Mrscraper offers an AI-powered solution that extracts data and returns it in JSON format. Simply submit a URL, and Mrscraper will do the heavy lifting, delivering structured JSON data ready for analysis.
For more information, visit Python’s official documentation.
Table of Contents
Take a Taste of Easy Scraping!
Get started now!
Step up your web scraping
Find more insights here
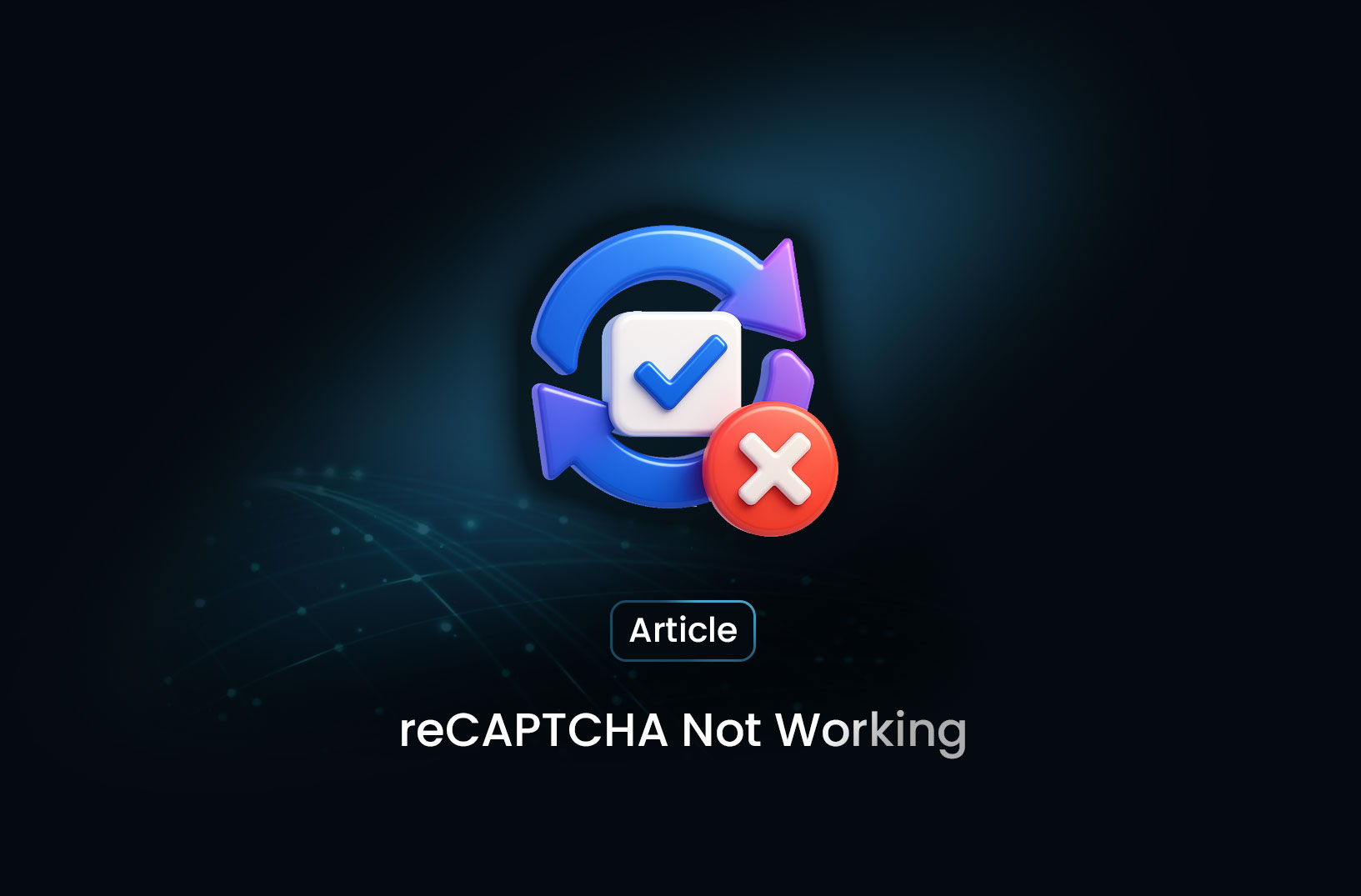
How to Diagnose and Resolve “reCAPTCHA Not Working” Issues
When users encounter an unresponsive or broken reCAPTCHA—such as an endless loading spinner or invalid site key—it disrupts both user experience and site security. This guide helps administrators and end users troubleshoot effectively.
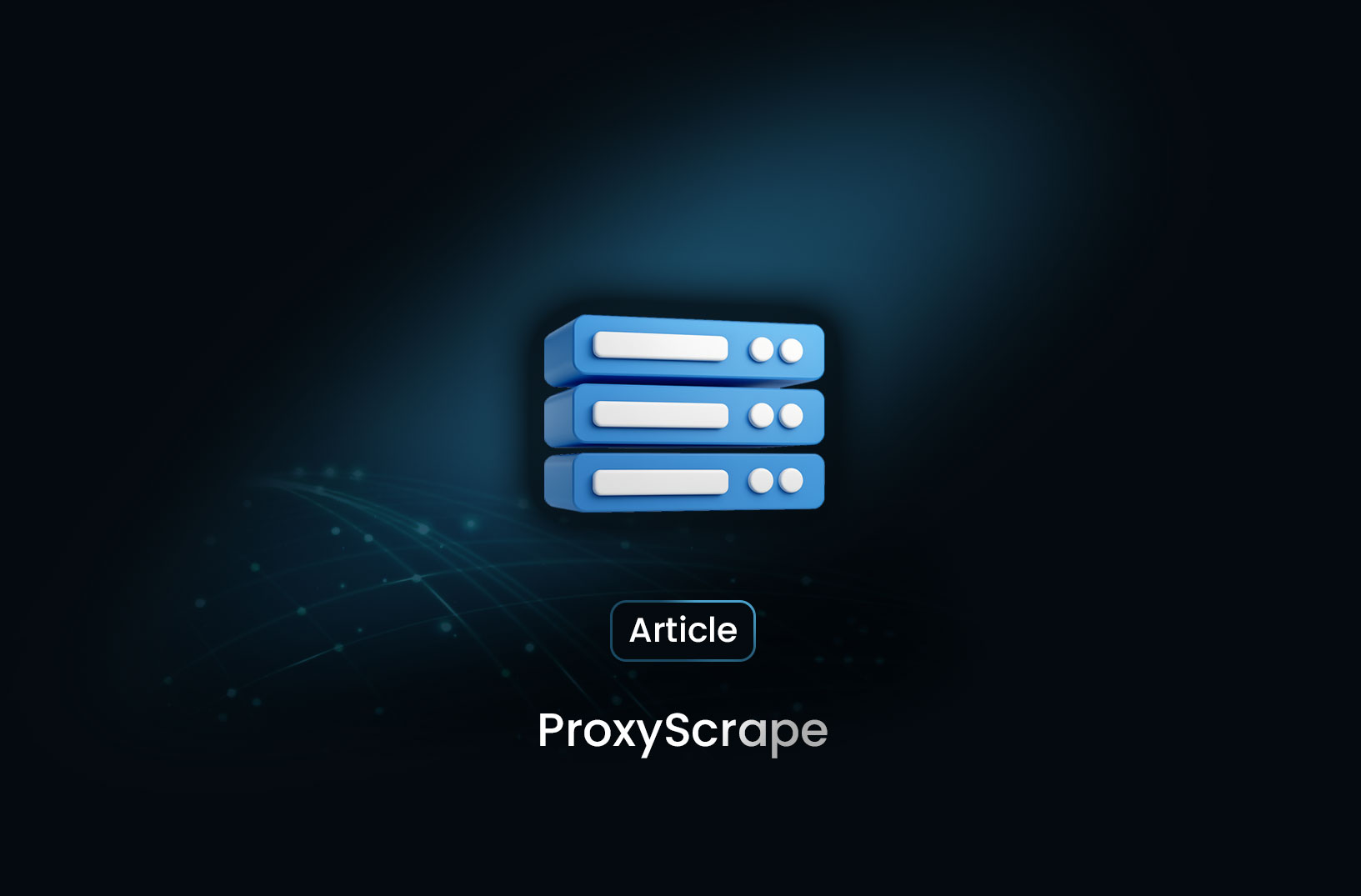
What is ProxyScrape? A Comprehensive Overview
ProxyScrape is a well-known proxy provider offering both free and premium proxy solutions. These include residential, datacenter, HTTP/S, and SOCKS proxies designed to enhance online anonymity, bypass geo-restrictions, and support activities such as web scraping, SEO monitoring, and brand protection.
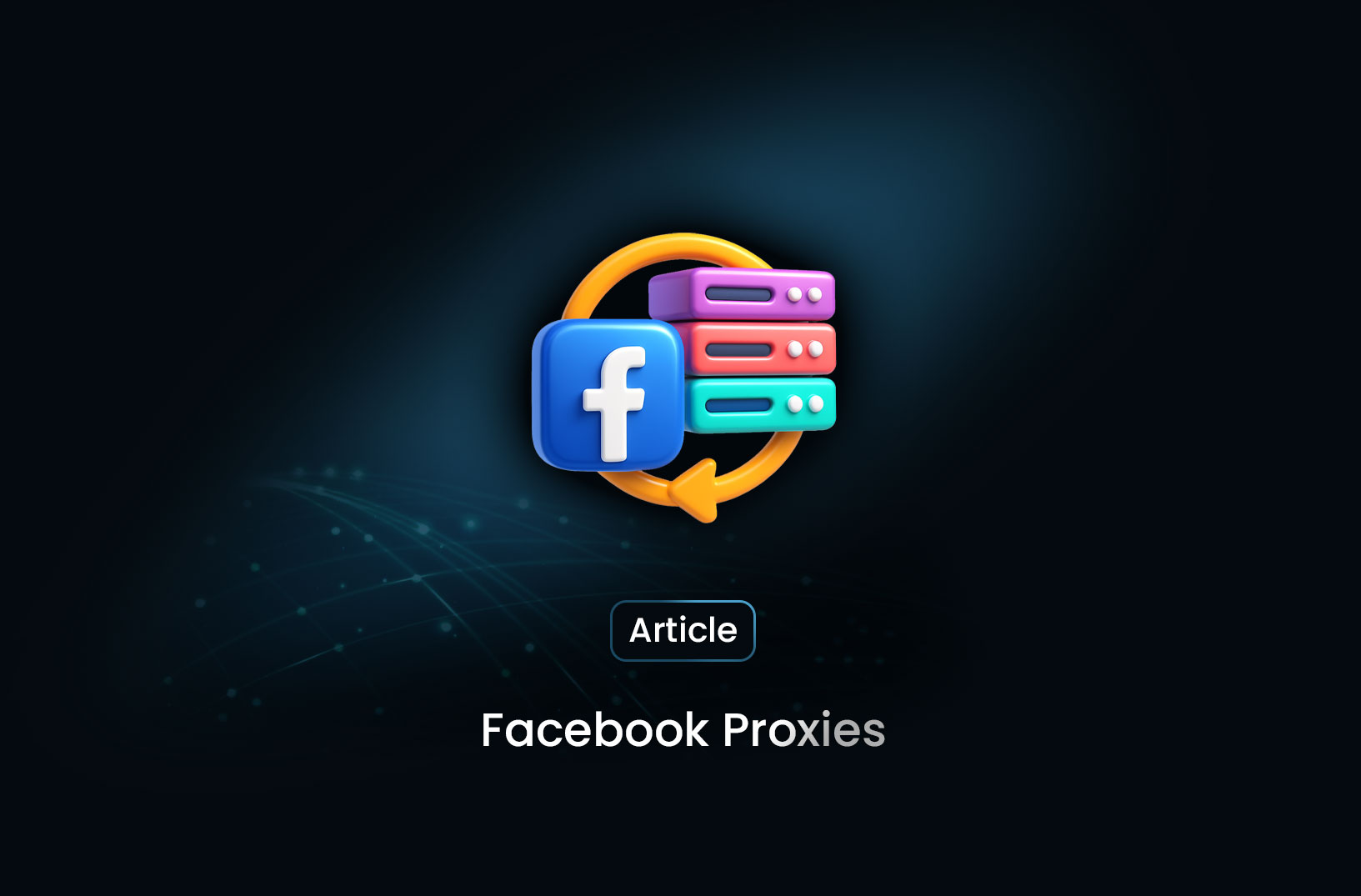
Best Facebook Proxies for Ads, Scraping, and Privacy in 2025
Facebook proxy routes your device’s Facebook traffic through an intermediary server. Instead of connecting directly, you use the proxy server to access Facebook, masking your real IP and location.
@MrScraper_
@MrScraper