API for Dummies: A Technical Introduction
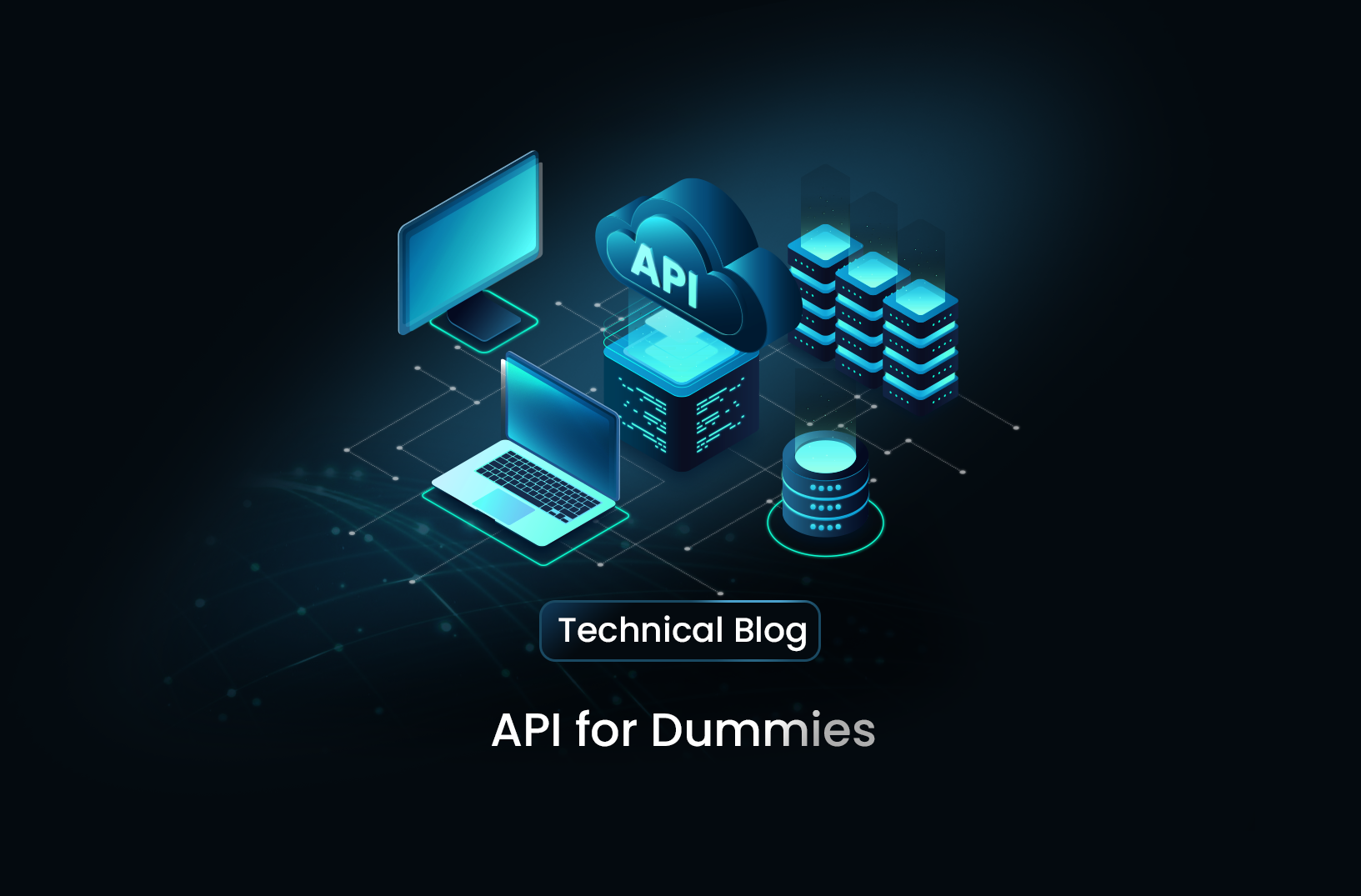
APIs (Application Programming Interfaces) have become a cornerstone of modern software development, connecting different applications, platforms, and services in ways that are both seamless and efficient. Whether you’re building an app, integrating third-party services, or creating automated workflows, APIs provide the glue that holds many systems together. In this article, we’ll dive into the technical details behind APIs to help you understand how they work and how to use them effectively.
What is an API?
At its core, an API is a set of rules that allows one piece of software to interact with another. Think of an API as a contract between two systems that outlines how they can communicate. It defines the methods, data formats, and protocols that two applications will use to exchange information. For example, when your app fetches data from a server, it uses an API to request and retrieve that data. This interaction typically happens over the internet using HTTP (HyperText Transfer Protocol), making APIs a critical part of web-based applications.
Types of APIs
APIs come in various flavors, depending on their purpose and scope. Here are the most common types:
Types of APIs
APIs come in various flavors, depending on their purpose and scope. Here are the most common types:
-
Web APIs: These are the most widely used APIs, enabling communication between a client (such as a browser or mobile app) and a server over the internet. Web APIs often use HTTP/HTTPS as the protocol.
- REST (Representational State Transfer): A popular architectural style for designing networked applications. REST APIs follow principles like statelessness, resource-based URLs, and standard HTTP methods (GET, POST, PUT, DELETE).
- SOAP (Simple Object Access Protocol): A more complex protocol for APIs that uses XML for message formatting. SOAP is known for its robustness and strict standards, often used in enterprise environments.
- GraphQL: A query language for APIs developed by Facebook. GraphQL allows clients to request specific data rather than fetching a fixed structure, making it more flexible than REST for certain use cases.
-
Operating System APIs: These APIs allow applications to interact with the underlying operating system. For instance, when an app accesses system resources like memory, file systems, or input/output devices, it often uses OS APIs.
-
Library APIs: Many programming libraries expose APIs that allow developers to leverage specific functionality without needing to build it from scratch. For example, a math library may offer APIs for complex calculations.
-
Hardware APIs: These interfaces enable software to interact directly with hardware devices, such as graphics cards, sensors, or printers.
API Architecture and Protocols
APIs typically follow specific architecture styles and protocols, which dictate how data is exchanged. The three most common protocols include
1. REST (Representational State Transfer)
REST is the most popular architectural style for designing APIs. It’s lightweight, simple to use, and built on standard HTTP methods such as:
- GET: Retrieve data from the server.
- POST: Send data to the server to create a new resource.
- PUT: Update an existing resource on the server.
- DELETE: Remove a resource from the server.
REST APIs rely on URL paths to specify resources, and they typically return data in JSON (JavaScript Object Notation) or XML format. Here's an example of a REST API call using the GET
method to retrieve a list of users:
GET https://api.example.com/users
The response might look like this (in JSON format):
[
{
"id": 1,
"name": "John Doe",
"email": "john@example.com"
},
{
"id": 2,
"name": "Jane Smith",
"email": "jane@example.com"
}
]
2. SOAP (Simple Object Access Protocol)
SOAP is a protocol that uses XML for message formatting and relies on a stricter set of rules compared to REST. SOAP is more suitable for applications that require strong security, ACID (Atomicity, Consistency, Isolation, Durability) transactions, and high reliability.
A SOAP request might look like this:
<soap:Envelope xmlns:soap="http://www.w3.org/2003/05/soap-envelope">
<soap:Body>
<GetUser xmlns="http://example.com/api">
<UserId>123</UserId>
</GetUser>
</soap:Body>
</soap:Envelope>
3. GraphQL
GraphQL allows clients to request exactly the data they need and nothing more. Unlike REST, which has a fixed set of endpoints returning fixed data structures, GraphQL allows more flexibility in querying.
A GraphQL query to retrieve a user's name and email might look like this:
{
user(id: "1") {
name
email
}
}
And the response could be:
{
"data": {
"user": {
"name": "John Doe",
"email": "john@example.com"
}
}
}
Authentication and Security in APIs
Security is a critical aspect of API development, especially for public-facing APIs. Some common authentication methods include:
- API Keys: A simple method where clients include an API key (a unique identifier) in their request headers. This key is provided by the API provider when you sign up for their service.
- OAuth 2.0: A more advanced authentication protocol that allows applications to act on behalf of users. OAuth 2.0 involves the exchange of tokens rather than passwords, making it more secure.
- JWT (JSON Web Tokens): A compact, self-contained token that securely transmits information between parties as a JSON object. JWTs are commonly used in token-based authentication.
API Rate Limiting
To protect against abuse or overuse, APIs often implement rate limiting. This restricts the number of requests a client can make in a specific time frame. For example, a rate limit might allow 100 requests per minute, after which further requests are denied until the time limit resets.
Rate limits help ensure that the server remains responsive and that its resources are fairly allocated among all users.
Versioning APIs
API versioning is a practice where multiple versions of an API are maintained concurrently. This allows developers to make changes and improvements to the API without breaking existing clients.
There are several approaches to API versioning:
- URI Versioning: The API version is included in the URL path (e.g., /v1/users).
- Header Versioning: The API version is specified in the request header.
- Query String Versioning: The version is included as a query parameter (e.g., ?version=1).
Error Handling in APIs
Effective error handling is crucial in APIs. Most APIs return standard HTTP status codes to indicate the outcome of a request:
- 200 OK: The request was successful.
- 201 Created: A new resource was successfully created.
- 400 Bad Request: The request was malformed or contains invalid data.
- 401 Unauthorized: The client needs to authenticate before accessing the resource.
- 404 Not Found: The requested resource could not be found.
- 500 Internal Server Error: The server encountered an error and could not complete the request.
In addition to HTTP status codes, many APIs return detailed error messages in the response body to help developers troubleshoot issues.
Building Your Own API
If you’re looking to build your own API, here are the steps you can follow:
- Define Your Resources: Identify the entities or resources your API will expose (e.g., users, products, orders).
- Choose an Architecture: Decide whether to use REST, SOAP, or GraphQL based on your use case.
- Set Up Endpoints: Create endpoints that map to specific resources and operations. For example, /users might retrieve or create users, while /orders might handle order data.
- Implement Authentication: Secure your API by implementing API keys, OAuth, or JWTs.
- Test and Document: Ensure that your API is well-tested and well-documented so that other developers can easily use it. Tools like Postman can help with testing, while platforms like Swagger or OpenAPI can assist with documentation.
Conclusion
APIs are a powerful tool for connecting applications and enabling communication between different systems. Whether you’re consuming APIs from third-party services or building your own, understanding the technical details behind API architecture, protocols, and security is crucial to becoming a proficient developer. By mastering these concepts, you can leverage the full potential of APIs in your projects.
Table of Contents
Take a Taste of Easy Scraping!
Get started now!
Step up your web scraping
Find more insights here
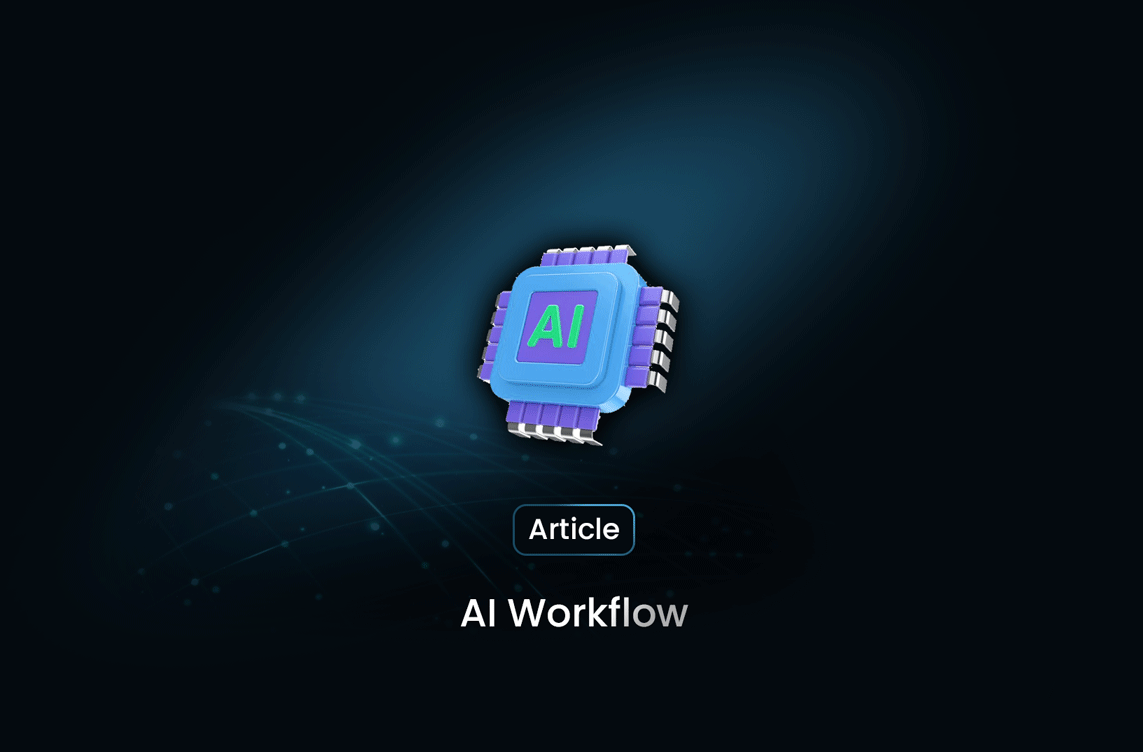
AI Workflow: Automating Customer Support with AI
Artificial Intelligence (AI) workflows are structured processes that guide the development, deployment, and usage of AI systems to solve specific problems or automate tasks. This guide provides a clear understanding of AI workflows, a practical use case, and simple, beginner-friendly steps to implement one.
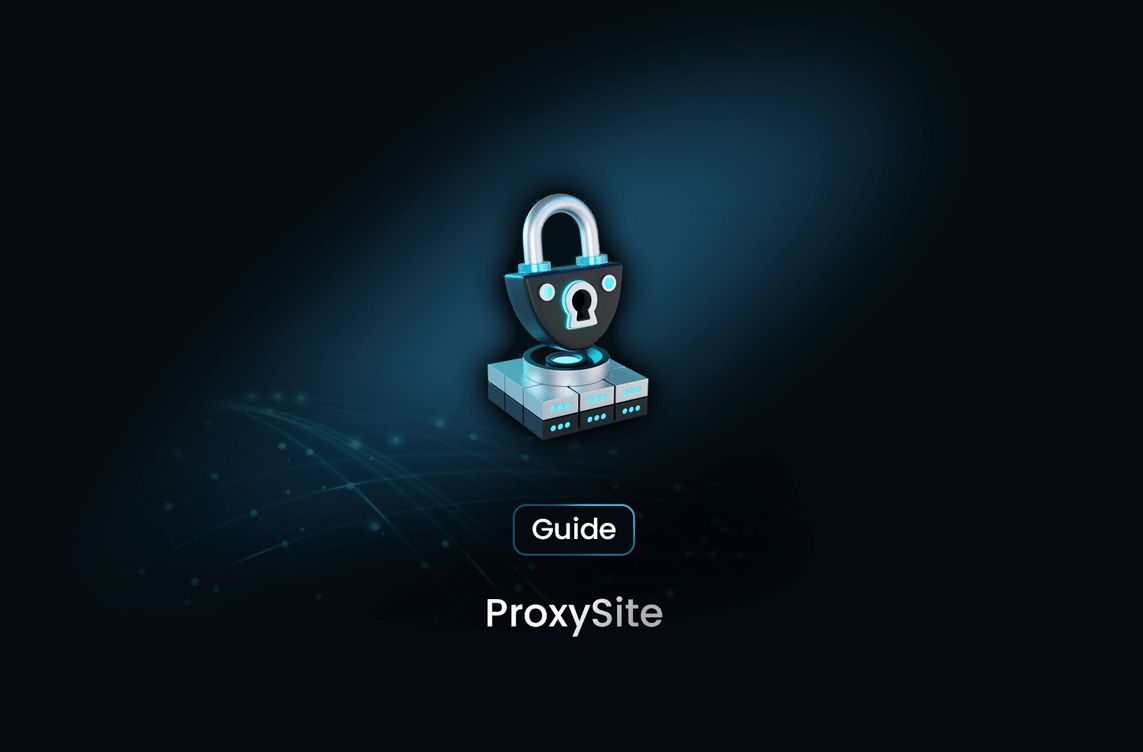
ProxySite: A Beginner's Guide to Anonymity Online
A ProxySite is an online service that routes your web traffic through a remote server, hiding your original IP address.
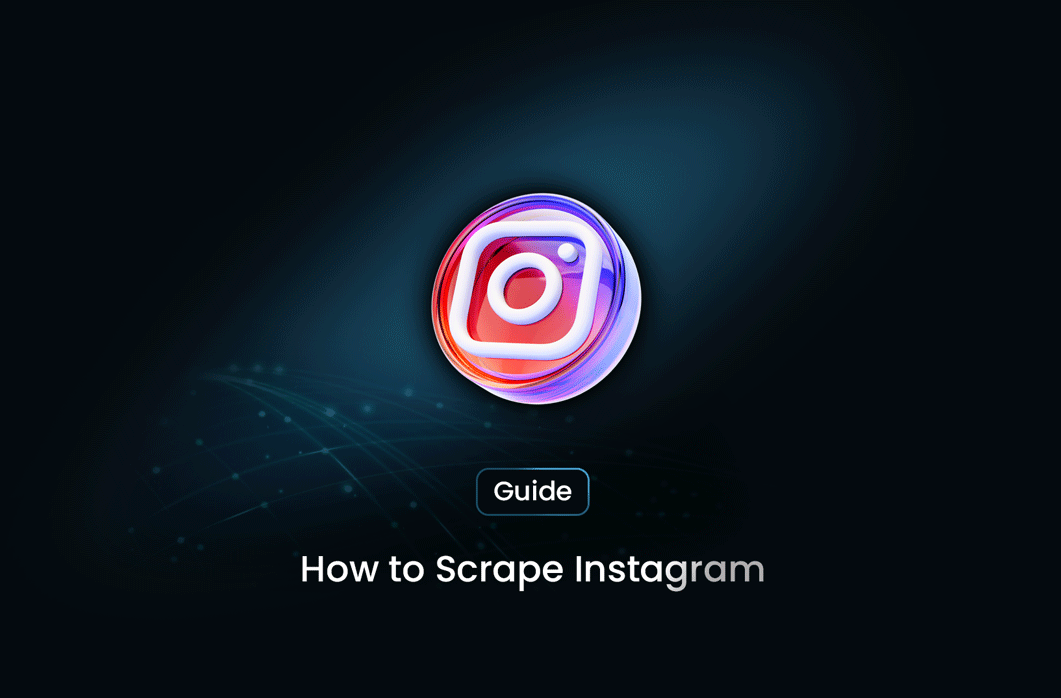
How to Scrape Instagram using Python
An Instagram scraper automates the extraction of this data, providing valuable insights for marketing, research, and trend analysis. This guide will walk you through the basics of Instagram scraping, highlight a practical use case, and provide a step-by-step technical guide for beginners.
@MrScraper_
@MrScraper